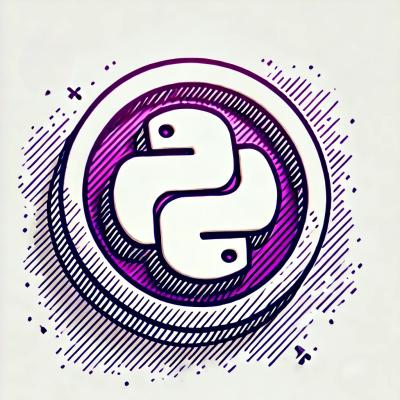
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
expo-crypto
Advanced tools
Provides cryptography primitives for Android, iOS and web.
The expo-crypto package provides cryptographic utilities for Expo and React Native applications. It allows developers to perform various cryptographic operations such as hashing, HMAC, and generating random bytes.
Hashing
This feature allows you to generate a hash of a given string using various algorithms like SHA-256. The code sample demonstrates how to generate a SHA-256 hash of the string 'Hello, world!'.
const Crypto = require('expo-crypto');
async function generateHash() {
const digest = await Crypto.digestStringAsync(
Crypto.CryptoDigestAlgorithm.SHA256,
'Hello, world!'
);
console.log(digest);
}
generateHash();
HMAC
This feature allows you to generate an HMAC (Hash-based Message Authentication Code) using a specified algorithm and key. The code sample demonstrates how to generate an HMAC using SHA-256 and a secret key.
const Crypto = require('expo-crypto');
async function generateHMAC() {
const hmac = await Crypto.hmacStringAsync(
Crypto.CryptoDigestAlgorithm.SHA256,
'Hello, world!',
'secret-key'
);
console.log(hmac);
}
generateHMAC();
Random Bytes
This feature allows you to generate a specified number of random bytes. The code sample demonstrates how to generate 16 random bytes.
const Crypto = require('expo-crypto');
async function generateRandomBytes() {
const randomBytes = await Crypto.getRandomBytesAsync(16);
console.log(randomBytes);
}
generateRandomBytes();
CryptoJS is a widely-used library that provides a variety of cryptographic algorithms including hashing, HMAC, encryption, and decryption. Unlike expo-crypto, CryptoJS is not specifically designed for React Native or Expo, but it can be used in both web and mobile applications.
react-native-crypto is a library that provides a native implementation of Node's crypto module for React Native. It offers similar functionalities to expo-crypto, such as hashing and HMAC, but with a broader scope as it aims to be a drop-in replacement for Node's crypto module.
Stanford Javascript Crypto Library (SJCL) is a library focused on cryptographic operations in JavaScript. It provides features like hashing, HMAC, and encryption. While it is not specifically designed for React Native or Expo, it can be used in those environments with some adjustments.
Provides cryptography primitives.
For managed Expo projects, please follow the installation instructions in the API documentation for the latest stable release.
For bare React Native projects, you must ensure that you have installed and configured the expo
package before continuing.
npx expo install expo-crypto
No additional set up necessary.
Run npx pod-install
after installing the npm package.
Contributions are very welcome! Please refer to guidelines described in the contributing guide.
FAQs
Provides cryptography primitives for Android, iOS and web.
The npm package expo-crypto receives a total of 132,190 weekly downloads. As such, expo-crypto popularity was classified as popular.
We found that expo-crypto demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 28 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.