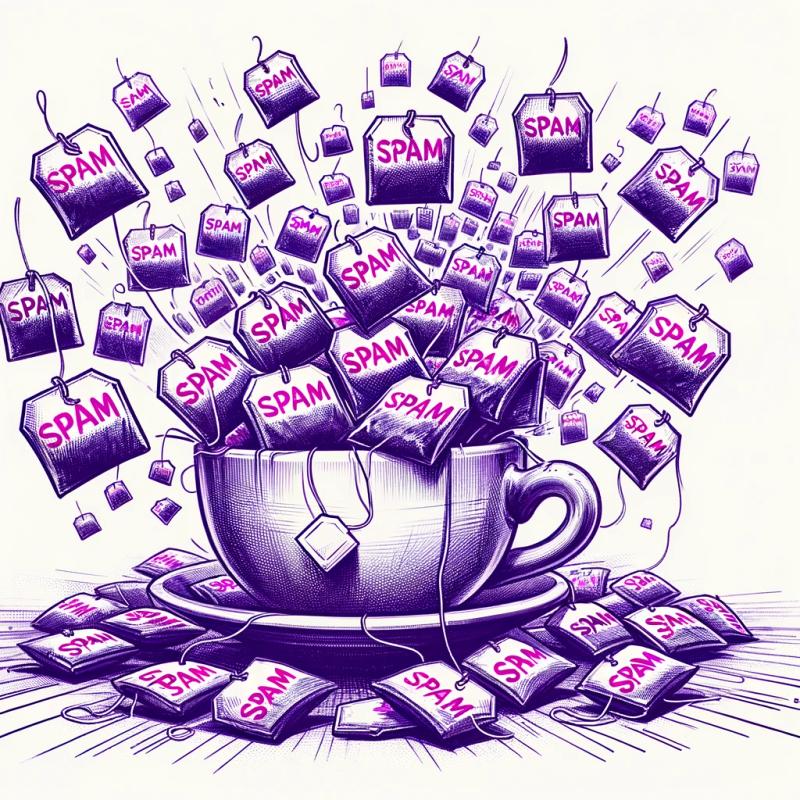
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
expo-location
Advanced tools
Readme
Allows reading geolocation information from the device. Your app can poll for the current location or subscribe to location update events.
For managed Expo projects, please follow the installation instructions in the API documentation for the latest stable release.
For bare React Native projects, you must ensure that you have installed and configured the expo
package before continuing.
npx expo install expo-location
Add NSLocationAlwaysAndWhenInUseUsageDescription
, NSLocationAlwaysUsageDescription
and NSLocationWhenInUseUsageDescription
keys to your Info.plist
:
<key>NSLocationAlwaysAndWhenInUseUsageDescription</key>
<string>Allow $(PRODUCT_NAME) to use your location</string>
<key>NSLocationAlwaysUsageDescription</key>
<string>Allow $(PRODUCT_NAME) to use your location</string>
<key>NSLocationWhenInUseUsageDescription</key>
<string>Allow $(PRODUCT_NAME) to use your location</string>
Run npx pod-install
after installing the npm package.
This module requires the permissions for approximate and exact device location. It also needs the foreground service permission to subscribe to location updates, while the app is in use. These permissions are automatically added.
<!-- Added permissions -->
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
<!-- Optional permissions -->
<uses-permission android:name="android.permission.FOREGROUND_SERVICE" />
<uses-permission android:name="android.permission.FOREGROUND_SERVICE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_BACKGROUND_LOCATION" />
Note: on Android, you have to submit your app for review and request access to use the background location permission or foreground location permissions.
Contributions are very welcome! Please refer to guidelines described in the contributing guide.
FAQs
Allows reading geolocation information from the device. Your app can poll for the current location or subscribe to location update events.
The npm package expo-location receives a total of 80,610 weekly downloads. As such, expo-location popularity was classified as popular.
We found that expo-location demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 31 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.