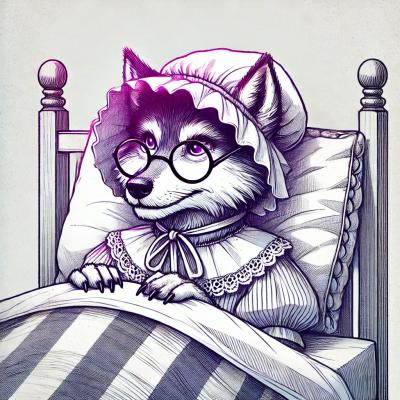
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
expo-server-sdk
Advanced tools
The expo-server-sdk is a Node.js library for sending push notifications to devices using the Expo push notification service. It allows you to send notifications to both iOS and Android devices with ease.
Sending Push Notifications
This feature allows you to send push notifications to multiple devices. The code sample demonstrates how to create a list of messages and send them in chunks using the Expo push notification service.
const { Expo } = require('expo-server-sdk');
let expo = new Expo();
let messages = [];
let somePushTokens = ['ExponentPushToken[xxxxxxxxxxxxxxxxxxxxxx]', 'ExponentPushToken[yyyyyyyyyyyyyyyyyyyyyy]'];
for (let pushToken of somePushTokens) {
if (!Expo.isExpoPushToken(pushToken)) {
console.error(`Push token ${pushToken} is not a valid Expo push token`);
continue;
}
messages.push({
to: pushToken,
sound: 'default',
body: 'This is a test notification',
data: { withSome: 'data' },
});
}
let chunks = expo.chunkPushNotifications(messages);
let tickets = [];
(async () => {
for (let chunk of chunks) {
try {
let ticketChunk = await expo.sendPushNotificationsAsync(chunk);
tickets.push(...ticketChunk);
} catch (error) {
console.error(error);
}
}
})();
Handling Receipts
This feature allows you to handle receipts for the notifications you have sent. The code sample demonstrates how to retrieve and process the status of sent notifications using their receipt IDs.
let receiptIds = ['xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'];
(async () => {
try {
let receipts = await expo.getPushNotificationReceiptsAsync(receiptIds);
for (let receiptId in receipts) {
let { status, message, details } = receipts[receiptId];
if (status === 'ok') {
continue;
} else if (status === 'error') {
console.error(`There was an error sending a notification: ${message}`);
if (details && details.error) {
console.error(`The error code is ${details.error}`);
}
}
}
} catch (error) {
console.error(error);
}
})();
node-pushnotifications is a Node.js library for sending push notifications to multiple platforms including iOS, Android, and Windows. It provides a unified API for different push notification services, making it versatile but potentially more complex to set up compared to expo-server-sdk.
firebase-admin is the official Firebase SDK for server-side use. It allows you to send push notifications via Firebase Cloud Messaging (FCM). While it offers more features beyond push notifications, it requires Firebase project setup and is more complex compared to the straightforward setup of expo-server-sdk.
onesignal-node is a Node.js client for OneSignal, a service that provides push notifications, email, and SMS messaging. It offers a rich set of features and analytics but requires integration with the OneSignal service, which can be more involved than using expo-server-sdk.
Server-side library for working with Expo using Node.js.
If you have problems with the code in this repository, please file issues & bug reports at https://github.com/expo/expo. Thanks!
yarn add expo-server-sdk
Note for Firebase: You must have a paid plan to send push notifications with this library. As Firebase's pricing page says, "The Spark plan allows outbound network requests only to Google-owned services."
import Expo from 'expo-server-sdk';
// Create a new Expo SDK client
let expo = new Expo();
// Create the messages that you want to send to clents
let messages = [];
for (let pushToken of somePushTokens) {
// Each push token looks like ExponentPushToken[xxxxxxxxxxxxxxxxxxxxxx]
// Check that all your push tokens appear to be valid Expo push tokens
if (!Expo.isExpoPushToken(pushToken)) {
console.error(`Push token ${pushToken} is not a valid Expo push token`);
continue;
}
// Construct a message (see https://docs.expo.io/versions/latest/guides/push-notifications.html)
messages.push({
to: pushToken,
sound: 'default',
body: 'This is a test notification',
data: { withSome: 'data' },
})
}
// The Expo push notification service accepts batches of notifications so
// that you don't need to send 1000 requests to send 1000 notifications. We
// recommend you batch your notifications to reduce the number of requests
// and to compress them (notifications with similar content will get
// compressed).
let chunks = expo.chunkPushNotifications(messages);
(async () => {
// Send the chunks to the Expo push notification service. There are
// different strategies you could use. A simple one is to send one chunk at a
// time, which nicely spreads the load out over time:
for (let chunk of chunks) {
try {
let receipts = await expo.sendPushNotificationsAsync(chunk);
console.log(receipts);
} catch (error) {
console.error(error);
}
}
})();
The source code is in the src/
directory and babel is used to turn it into ES5 that goes in the build/
directory.
To build, npm run build
.
To build and watch for changes, npm run watch
.
FAQs
Server-side library for working with Expo using Node.js
The npm package expo-server-sdk receives a total of 105,467 weekly downloads. As such, expo-server-sdk popularity was classified as popular.
We found that expo-server-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 27 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.