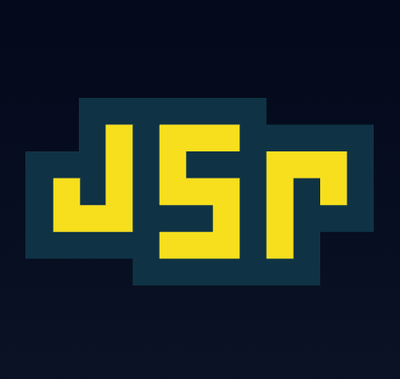
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Expo is a framework and a platform for universal React applications. It provides a set of tools and services built around React Native and native platforms that help you develop, build, deploy, and quickly iterate on iOS, Android, and web apps from the same JavaScript/TypeScript codebase.
Development Environment
Expo provides a managed workflow that simplifies the setup of a development environment. The `registerRootComponent` function is used to register the main component of your application, making it easy to get started with a new project.
import { registerRootComponent } from 'expo';
import App from './App';
registerRootComponent(App);
Asset Management
Expo's asset management system allows you to easily manage and load assets such as images, fonts, and other media files. The `Asset` module helps in loading and caching these assets efficiently.
import { Asset } from 'expo-asset';
const image = Asset.fromModule(require('./path/to/image.png')).uri;
Push Notifications
Expo provides a robust push notification system that allows you to send and receive notifications. The `expo-notifications` module helps in setting up and handling push notifications in your app.
import * as Notifications from 'expo-notifications';
async function sendPushNotification(expoPushToken) {
const message = {
to: expoPushToken,
sound: 'default',
title: 'Original Title',
body: 'And here is the body!',
data: { someData: 'goes here' },
};
await fetch('https://exp.host/--/api/v2/push/send', {
method: 'POST',
headers: {
Accept: 'application/json',
'Content-Type': 'application/json',
},
body: JSON.stringify(message),
});
}
Location Services
Expo provides access to device location services through the `expo-location` module. This allows you to request permissions and get the current location of the device.
import * as Location from 'expo-location';
async function getLocation() {
let { status } = await Location.requestForegroundPermissionsAsync();
if (status !== 'granted') {
console.log('Permission to access location was denied');
return;
}
let location = await Location.getCurrentPositionAsync({});
console.log(location);
}
Camera Access
Expo provides easy access to the device's camera through the `expo-camera` module. This allows you to request permissions, open the camera, and take pictures.
import { Camera } from 'expo-camera';
async function takePicture() {
const { status } = await Camera.requestPermissionsAsync();
if (status === 'granted') {
const camera = await Camera.openAsync();
const photo = await camera.takePictureAsync();
console.log(photo.uri);
}
}
React Native is a framework for building native apps using React. Unlike Expo, React Native requires more setup and configuration but offers more flexibility and control over the native code. It is suitable for developers who need to integrate custom native modules or require more advanced native functionalities.
Apache Cordova is a mobile application development framework that allows you to use standard web technologies such as HTML5, CSS3, and JavaScript for cross-platform development. Compared to Expo, Cordova provides a different approach by wrapping web applications in a native container, which can be less performant than React Native-based solutions.
Flutter is a UI toolkit from Google for building natively compiled applications for mobile, web, and desktop from a single codebase. Unlike Expo, which is based on React Native, Flutter uses the Dart programming language and provides a different set of tools and widgets for building applications.
The expo
package is a single package you can install in any React Native app to begin using Expo modules.
expo-modules-core
and expo-modules-autolinking
.expo-asset
.@expo/cli
, a small CLI that provides a clean interface around both bundlers (such as Metro and Webpack) and native build tools (Xcode, Simulator.app, Android Studio, ADB, etc.), can generate native projects with npx expo prebuild
, and aligns compatible package versions with npx expo install
.expo-font
and to function in Expo Go (optional, only if applicable).See CONTRIBUTING for instructions on working on this package.
FAQs
The Expo SDK
The npm package expo receives a total of 628,470 weekly downloads. As such, expo popularity was classified as popular.
We found that expo demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 29 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.