express-generator
Advanced tools
Comparing version 4.15.5 to 4.16.0
@@ -5,2 +5,3 @@ #!/usr/bin/env node | ||
var fs = require('fs') | ||
var minimatch = require('minimatch') | ||
var mkdirp = require('mkdirp') | ||
@@ -15,8 +16,7 @@ var path = require('path') | ||
var MODE_0755 = parseInt('0755', 8) | ||
var TEMPLATE_DIR = path.join(__dirname, '..', 'templates') | ||
var VERSION = require('../package').version | ||
var _exit = process.exit | ||
var pkg = require('../package.json') | ||
var version = pkg.version | ||
// Re-assign process.exit because of commander | ||
@@ -51,3 +51,3 @@ // TODO: Switch to a different command framework | ||
.name('express') | ||
.version(version, ' --version') | ||
.version(VERSION, ' --version') | ||
.usage('[options] [dir]') | ||
@@ -59,2 +59,3 @@ .option('-e, --ejs', 'add ejs engine support', renamedOption('--ejs', '--view=ejs')) | ||
.option('-v, --view <engine>', 'add view <engine> support (dust|ejs|hbs|hjs|jade|pug|twig|vash) (defaults to jade)') | ||
.option(' --no-view', 'use static html instead of view engine') | ||
.option('-c, --css <engine>', 'add stylesheet <engine> support (less|stylus|compass|sass) (defaults to plain css)') | ||
@@ -117,33 +118,39 @@ .option(' --git', 'add .gitignore') | ||
function copyTemplate (from, to) { | ||
from = path.join(__dirname, '..', 'templates', from) | ||
write(to, fs.readFileSync(from, 'utf-8')) | ||
write(to, fs.readFileSync(path.join(TEMPLATE_DIR, from), 'utf-8')) | ||
} | ||
/** | ||
* Create application at the given directory `path`. | ||
* Copy multiple files from template directory. | ||
*/ | ||
function copyTemplateMulti (fromDir, toDir, nameGlob) { | ||
fs.readdirSync(path.join(TEMPLATE_DIR, fromDir)) | ||
.filter(minimatch.filter(nameGlob, { matchBase: true })) | ||
.forEach(function (name) { | ||
copyTemplate(path.join(fromDir, name), path.join(toDir, name)) | ||
}) | ||
} | ||
/** | ||
* Create application at the given directory. | ||
* | ||
* @param {String} path | ||
* @param {string} name | ||
* @param {string} dir | ||
*/ | ||
function createApplication (name, path) { | ||
var wait = 5 | ||
function createApplication (name, dir) { | ||
console.log() | ||
function complete () { | ||
if (--wait) return | ||
var prompt = launchedFromCmd() ? '>' : '$' | ||
console.log() | ||
console.log(' install dependencies:') | ||
console.log(' %s cd %s && npm install', prompt, path) | ||
console.log() | ||
console.log(' run the app:') | ||
if (launchedFromCmd()) { | ||
console.log(' %s SET DEBUG=%s:* & npm start', prompt, name) | ||
} else { | ||
console.log(' %s DEBUG=%s:* npm start', prompt, name) | ||
// Package | ||
var pkg = { | ||
name: name, | ||
version: '0.0.0', | ||
private: true, | ||
scripts: { | ||
start: 'node ./bin/www' | ||
}, | ||
dependencies: { | ||
'debug': '~2.6.9', | ||
'express': '~4.16.0' | ||
} | ||
console.log() | ||
} | ||
@@ -159,194 +166,200 @@ | ||
// App modules | ||
app.locals.localModules = Object.create(null) | ||
app.locals.modules = Object.create(null) | ||
app.locals.mounts = [] | ||
app.locals.uses = [] | ||
mkdir(path, function () { | ||
mkdir(path + '/public', function () { | ||
mkdir(path + '/public/javascripts') | ||
mkdir(path + '/public/images') | ||
mkdir(path + '/public/stylesheets', function () { | ||
switch (program.css) { | ||
case 'less': | ||
copyTemplate('css/style.less', path + '/public/stylesheets/style.less') | ||
break | ||
case 'stylus': | ||
copyTemplate('css/style.styl', path + '/public/stylesheets/style.styl') | ||
break | ||
case 'compass': | ||
copyTemplate('css/style.scss', path + '/public/stylesheets/style.scss') | ||
break | ||
case 'sass': | ||
copyTemplate('css/style.sass', path + '/public/stylesheets/style.sass') | ||
break | ||
default: | ||
copyTemplate('css/style.css', path + '/public/stylesheets/style.css') | ||
break | ||
} | ||
complete() | ||
}) | ||
}) | ||
// Request logger | ||
app.locals.modules.logger = 'morgan' | ||
app.locals.uses.push("logger('dev')") | ||
pkg.dependencies.morgan = '~1.9.0' | ||
mkdir(path + '/routes', function () { | ||
copyTemplate('js/routes/index.js', path + '/routes/index.js') | ||
copyTemplate('js/routes/users.js', path + '/routes/users.js') | ||
complete() | ||
}) | ||
// Body parsers | ||
app.locals.uses.push('express.json()') | ||
app.locals.uses.push('express.urlencoded({ extended: false })') | ||
mkdir(path + '/views', function () { | ||
switch (program.view) { | ||
case 'dust': | ||
copyTemplate('dust/index.dust', path + '/views/index.dust') | ||
copyTemplate('dust/error.dust', path + '/views/error.dust') | ||
break | ||
case 'ejs': | ||
copyTemplate('ejs/index.ejs', path + '/views/index.ejs') | ||
copyTemplate('ejs/error.ejs', path + '/views/error.ejs') | ||
break | ||
case 'jade': | ||
copyTemplate('jade/index.jade', path + '/views/index.jade') | ||
copyTemplate('jade/layout.jade', path + '/views/layout.jade') | ||
copyTemplate('jade/error.jade', path + '/views/error.jade') | ||
break | ||
case 'hjs': | ||
copyTemplate('hogan/index.hjs', path + '/views/index.hjs') | ||
copyTemplate('hogan/error.hjs', path + '/views/error.hjs') | ||
break | ||
case 'hbs': | ||
copyTemplate('hbs/index.hbs', path + '/views/index.hbs') | ||
copyTemplate('hbs/layout.hbs', path + '/views/layout.hbs') | ||
copyTemplate('hbs/error.hbs', path + '/views/error.hbs') | ||
break | ||
case 'pug': | ||
copyTemplate('pug/index.pug', path + '/views/index.pug') | ||
copyTemplate('pug/layout.pug', path + '/views/layout.pug') | ||
copyTemplate('pug/error.pug', path + '/views/error.pug') | ||
break | ||
case 'twig': | ||
copyTemplate('twig/index.twig', path + '/views/index.twig') | ||
copyTemplate('twig/layout.twig', path + '/views/layout.twig') | ||
copyTemplate('twig/error.twig', path + '/views/error.twig') | ||
break | ||
case 'vash': | ||
copyTemplate('vash/index.vash', path + '/views/index.vash') | ||
copyTemplate('vash/layout.vash', path + '/views/layout.vash') | ||
copyTemplate('vash/error.vash', path + '/views/error.vash') | ||
break | ||
} | ||
complete() | ||
}) | ||
// Cookie parser | ||
app.locals.modules.cookieParser = 'cookie-parser' | ||
app.locals.uses.push('cookieParser()') | ||
pkg.dependencies['cookie-parser'] = '~1.4.3' | ||
// CSS Engine support | ||
switch (program.css) { | ||
case 'less': | ||
app.locals.modules.lessMiddleware = 'less-middleware' | ||
app.locals.uses.push("lessMiddleware(path.join(__dirname, 'public'))") | ||
break | ||
case 'stylus': | ||
app.locals.modules.stylus = 'stylus' | ||
app.locals.uses.push("stylus.middleware(path.join(__dirname, 'public'))") | ||
break | ||
case 'compass': | ||
app.locals.modules.compass = 'node-compass' | ||
app.locals.uses.push("compass({ mode: 'expanded' })") | ||
break | ||
case 'sass': | ||
app.locals.modules.sassMiddleware = 'node-sass-middleware' | ||
app.locals.uses.push("sassMiddleware({\n src: path.join(__dirname, 'public'),\n dest: path.join(__dirname, 'public'),\n indentedSyntax: true, // true = .sass and false = .scss\n sourceMap: true\n})") | ||
break | ||
} | ||
if (dir !== '.') { | ||
mkdir(dir, '.') | ||
} | ||
// Template support | ||
switch (program.view) { | ||
case 'dust': | ||
app.locals.modules.adaro = 'adaro' | ||
app.locals.view = { | ||
engine: 'dust', | ||
render: 'adaro.dust()' | ||
} | ||
break | ||
default: | ||
app.locals.view = { | ||
engine: program.view | ||
} | ||
break | ||
} | ||
mkdir(dir, 'public') | ||
mkdir(dir, 'public/javascripts') | ||
mkdir(dir, 'public/images') | ||
mkdir(dir, 'public/stylesheets') | ||
// package.json | ||
var pkg = { | ||
name: name, | ||
version: '0.0.0', | ||
private: true, | ||
scripts: { | ||
start: 'node ./bin/www' | ||
}, | ||
dependencies: { | ||
'body-parser': '~1.18.2', | ||
'cookie-parser': '~1.4.3', | ||
'debug': '~2.6.9', | ||
'express': '~4.15.5', | ||
'morgan': '~1.9.0', | ||
'serve-favicon': '~2.4.5' | ||
} | ||
} | ||
// copy css templates | ||
switch (program.css) { | ||
case 'less': | ||
copyTemplateMulti('css', dir + '/public/stylesheets', '*.less') | ||
break | ||
case 'stylus': | ||
copyTemplateMulti('css', dir + '/public/stylesheets', '*.styl') | ||
break | ||
case 'compass': | ||
copyTemplateMulti('css', dir + '/public/stylesheets', '*.scss') | ||
break | ||
case 'sass': | ||
copyTemplateMulti('css', dir + '/public/stylesheets', '*.sass') | ||
break | ||
default: | ||
copyTemplateMulti('css', dir + '/public/stylesheets', '*.css') | ||
break | ||
} | ||
// copy route templates | ||
mkdir(dir, 'routes') | ||
copyTemplateMulti('js/routes', dir + '/routes', '*.js') | ||
if (program.view) { | ||
// Copy view templates | ||
mkdir(dir, 'views') | ||
pkg.dependencies['http-errors'] = '~1.6.2' | ||
switch (program.view) { | ||
case 'dust': | ||
pkg.dependencies.adaro = '~1.0.4' | ||
copyTemplateMulti('views', dir + '/views', '*.dust') | ||
break | ||
case 'jade': | ||
pkg.dependencies['jade'] = '~1.11.0' | ||
break | ||
case 'ejs': | ||
pkg.dependencies['ejs'] = '~2.5.7' | ||
copyTemplateMulti('views', dir + '/views', '*.ejs') | ||
break | ||
case 'hbs': | ||
copyTemplateMulti('views', dir + '/views', '*.hbs') | ||
break | ||
case 'hjs': | ||
pkg.dependencies['hjs'] = '~0.0.6' | ||
copyTemplateMulti('views', dir + '/views', '*.hjs') | ||
break | ||
case 'hbs': | ||
pkg.dependencies['hbs'] = '~4.0.1' | ||
case 'jade': | ||
copyTemplateMulti('views', dir + '/views', '*.jade') | ||
break | ||
case 'pug': | ||
pkg.dependencies['pug'] = '2.0.0-beta11' | ||
copyTemplateMulti('views', dir + '/views', '*.pug') | ||
break | ||
case 'twig': | ||
pkg.dependencies['twig'] = '~0.10.3' | ||
copyTemplateMulti('views', dir + '/views', '*.twig') | ||
break | ||
case 'vash': | ||
pkg.dependencies['vash'] = '~0.12.2' | ||
copyTemplateMulti('views', dir + '/views', '*.vash') | ||
break | ||
} | ||
} else { | ||
// Copy extra public files | ||
copyTemplate('js/index.html', path.join(dir, 'public/index.html')) | ||
} | ||
// CSS Engine support | ||
switch (program.css) { | ||
case 'less': | ||
pkg.dependencies['less-middleware'] = '~2.2.1' | ||
break | ||
case 'compass': | ||
pkg.dependencies['node-compass'] = '0.2.3' | ||
break | ||
case 'stylus': | ||
pkg.dependencies['stylus'] = '0.54.5' | ||
break | ||
case 'sass': | ||
pkg.dependencies['node-sass-middleware'] = '0.9.8' | ||
break | ||
} | ||
// CSS Engine support | ||
switch (program.css) { | ||
case 'compass': | ||
app.locals.modules.compass = 'node-compass' | ||
app.locals.uses.push("compass({ mode: 'expanded' })") | ||
pkg.dependencies['node-compass'] = '0.2.3' | ||
break | ||
case 'less': | ||
app.locals.modules.lessMiddleware = 'less-middleware' | ||
app.locals.uses.push("lessMiddleware(path.join(__dirname, 'public'))") | ||
pkg.dependencies['less-middleware'] = '~2.2.1' | ||
break | ||
case 'sass': | ||
app.locals.modules.sassMiddleware = 'node-sass-middleware' | ||
app.locals.uses.push("sassMiddleware({\n src: path.join(__dirname, 'public'),\n dest: path.join(__dirname, 'public'),\n indentedSyntax: true, // true = .sass and false = .scss\n sourceMap: true\n})") | ||
pkg.dependencies['node-sass-middleware'] = '0.11.0' | ||
break | ||
case 'stylus': | ||
app.locals.modules.stylus = 'stylus' | ||
app.locals.uses.push("stylus.middleware(path.join(__dirname, 'public'))") | ||
pkg.dependencies['stylus'] = '0.54.5' | ||
break | ||
} | ||
// sort dependencies like npm(1) | ||
pkg.dependencies = sortedObject(pkg.dependencies) | ||
// Index router mount | ||
app.locals.localModules.indexRouter = './routes/index' | ||
app.locals.mounts.push({ path: '/', code: 'indexRouter' }) | ||
// write files | ||
write(path + '/package.json', JSON.stringify(pkg, null, 2) + '\n') | ||
write(path + '/app.js', app.render()) | ||
mkdir(path + '/bin', function () { | ||
write(path + '/bin/www', www.render(), MODE_0755) | ||
complete() | ||
}) | ||
// User router mount | ||
app.locals.localModules.usersRouter = './routes/users' | ||
app.locals.mounts.push({ path: '/users', code: 'usersRouter' }) | ||
if (program.git) { | ||
copyTemplate('js/gitignore', path + '/.gitignore') | ||
} | ||
// Template support | ||
switch (program.view) { | ||
case 'dust': | ||
app.locals.modules.adaro = 'adaro' | ||
app.locals.view = { | ||
engine: 'dust', | ||
render: 'adaro.dust()' | ||
} | ||
pkg.dependencies.adaro = '~1.0.4' | ||
break | ||
case 'ejs': | ||
app.locals.view = { engine: 'ejs' } | ||
pkg.dependencies.ejs = '~2.5.7' | ||
break | ||
case 'hbs': | ||
app.locals.view = { engine: 'hbs' } | ||
pkg.dependencies.hbs = '~4.0.1' | ||
break | ||
case 'hjs': | ||
app.locals.view = { engine: 'hjs' } | ||
pkg.dependencies.hjs = '~0.0.6' | ||
break | ||
case 'jade': | ||
app.locals.view = { engine: 'jade' } | ||
pkg.dependencies.jade = '~1.11.0' | ||
break | ||
case 'pug': | ||
app.locals.view = { engine: 'pug' } | ||
pkg.dependencies.pug = '2.0.0-beta11' | ||
break | ||
case 'twig': | ||
app.locals.view = { engine: 'twig' } | ||
pkg.dependencies.twig = '~0.10.3' | ||
break | ||
case 'vash': | ||
app.locals.view = { engine: 'vash' } | ||
pkg.dependencies.vash = '~0.12.4' | ||
break | ||
default: | ||
app.locals.view = false | ||
break | ||
} | ||
complete() | ||
}) | ||
// Static files | ||
app.locals.uses.push("express.static(path.join(__dirname, 'public'))") | ||
if (program.git) { | ||
copyTemplate('js/gitignore', path.join(dir, '.gitignore')) | ||
} | ||
// sort dependencies like npm(1) | ||
pkg.dependencies = sortedObject(pkg.dependencies) | ||
// write files | ||
write(path.join(dir, 'app.js'), app.render()) | ||
write(path.join(dir, 'package.json'), JSON.stringify(pkg, null, 2) + '\n') | ||
mkdir(dir, 'bin') | ||
write(path.join(dir, 'bin/www'), www.render(), MODE_0755) | ||
var prompt = launchedFromCmd() ? '>' : '$' | ||
if (dir !== '.') { | ||
console.log() | ||
console.log(' change directory:') | ||
console.log(' %s cd %s', prompt, dir) | ||
} | ||
console.log() | ||
console.log(' install dependencies:') | ||
console.log(' %s npm install', prompt) | ||
console.log() | ||
console.log(' run the app:') | ||
if (launchedFromCmd()) { | ||
console.log(' %s SET DEBUG=%s:* & npm start', prompt, name) | ||
} else { | ||
console.log(' %s DEBUG=%s:* npm start', prompt, name) | ||
} | ||
console.log() | ||
} | ||
@@ -362,3 +375,3 @@ | ||
return path.basename(pathName) | ||
.replace(/[^A-Za-z0-9.()!~*'-]+/g, '-') | ||
.replace(/[^A-Za-z0-9.-]+/g, '-') | ||
.replace(/^[-_.]+|-+$/g, '') | ||
@@ -369,10 +382,10 @@ .toLowerCase() | ||
/** | ||
* Check if the given directory `path` is empty. | ||
* Check if the given directory `dir` is empty. | ||
* | ||
* @param {String} path | ||
* @param {String} dir | ||
* @param {Function} fn | ||
*/ | ||
function emptyDirectory (path, fn) { | ||
fs.readdir(path, function (err, files) { | ||
function emptyDirectory (dir, fn) { | ||
fs.readdir(dir, function (err, files) { | ||
if (err && err.code !== 'ENOENT') throw err | ||
@@ -427,3 +440,5 @@ fn(!files || !files.length) | ||
function render () { | ||
return ejs.render(contents, locals) | ||
return ejs.render(contents, locals, { | ||
escape: util.inspect | ||
}) | ||
} | ||
@@ -449,3 +464,3 @@ | ||
// View engine | ||
if (program.view === undefined) { | ||
if (program.view === true) { | ||
if (program.ejs) program.view = 'ejs' | ||
@@ -458,3 +473,3 @@ if (program.hbs) program.view = 'hbs' | ||
// Default view engine | ||
if (program.view === undefined) { | ||
if (program.view === true) { | ||
warning('the default view engine will not be jade in future releases\n' + | ||
@@ -484,14 +499,13 @@ "use `--view=jade' or `--help' for additional options") | ||
/** | ||
* Mkdir -p. | ||
* Make the given dir relative to base. | ||
* | ||
* @param {String} path | ||
* @param {Function} fn | ||
* @param {string} base | ||
* @param {string} dir | ||
*/ | ||
function mkdir (path, fn) { | ||
mkdirp(path, MODE_0755, function (err) { | ||
if (err) throw err | ||
console.log(' \x1b[36mcreate\x1b[0m : ' + path) | ||
fn && fn() | ||
}) | ||
function mkdir (base, dir) { | ||
var loc = path.join(base, dir) | ||
console.log(' \x1b[36mcreate\x1b[0m : ' + loc + path.sep) | ||
mkdirp.sync(loc, MODE_0755) | ||
} | ||
@@ -528,11 +542,11 @@ | ||
/** | ||
* echo str > path. | ||
* echo str > file. | ||
* | ||
* @param {String} path | ||
* @param {String} file | ||
* @param {String} str | ||
*/ | ||
function write (path, str, mode) { | ||
fs.writeFileSync(path, str, { mode: mode || MODE_0666 }) | ||
console.log(' \x1b[36mcreate\x1b[0m : ' + path) | ||
function write (file, str, mode) { | ||
fs.writeFileSync(file, str, { mode: mode || MODE_0666 }) | ||
console.log(' \x1b[36mcreate\x1b[0m : ' + file) | ||
} |
{ | ||
"name": "express-generator", | ||
"description": "Express' application generator", | ||
"version": "4.15.5", | ||
"version": "4.16.0", | ||
"author": "TJ Holowaychuk <tj@vision-media.ca>", | ||
@@ -28,4 +28,5 @@ "contributors": [ | ||
"dependencies": { | ||
"commander": "2.11.0", | ||
"commander": "2.13.0", | ||
"ejs": "2.5.7", | ||
"minimatch": "3.0.4", | ||
"mkdirp": "0.5.1", | ||
@@ -40,7 +41,7 @@ "sorted-object": "2.0.1" | ||
"devDependencies": { | ||
"eslint": "3.19.0", | ||
"eslint-config-standard": "10.2.1", | ||
"eslint-plugin-import": "2.7.0", | ||
"eslint-plugin-node": "5.2.0", | ||
"eslint-plugin-promise": "3.5.0", | ||
"eslint": "4.18.2", | ||
"eslint-config-standard": "11.0.0", | ||
"eslint-plugin-import": "2.9.0", | ||
"eslint-plugin-node": "6.0.1", | ||
"eslint-plugin-promise": "3.7.0", | ||
"eslint-plugin-standard": "3.0.1", | ||
@@ -50,4 +51,5 @@ "mocha": "2.5.3", | ||
"supertest": "1.2.0", | ||
"tmp": "0.0.33", | ||
"tree-kill": "1.2.0", | ||
"validate-npm-package-name": "2.2.2" | ||
"validate-npm-package-name": "3.0.0" | ||
}, | ||
@@ -54,0 +56,0 @@ "engines": { |
@@ -9,3 +9,2 @@ [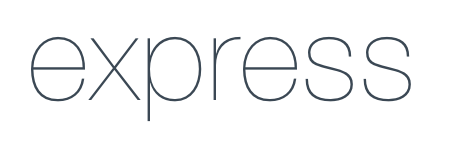](http://expressjs.com/) | ||
[![Windows Build][appveyor-image]][appveyor-url] | ||
[![Gratipay][gratipay-image]][gratipay-url] | ||
@@ -50,2 +49,3 @@ ## Installation | ||
-v, --view <engine> add view <engine> support (dust|ejs|hbs|hjs|jade|pug|twig|vash) (defaults to jade) | ||
--no-view use static html instead of view engine | ||
-c, --css <engine> add stylesheet <engine> support (less|stylus|compass|sass) (defaults to plain css) | ||
@@ -68,3 +68,1 @@ --git add .gitignore | ||
[downloads-url]: https://npmjs.org/package/express-generator | ||
[gratipay-image]: https://img.shields.io/gratipay/dougwilson.svg | ||
[gratipay-url]: https://gratipay.com/dougwilson/ |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
26249
36
478
5
12
66
+ Addedminimatch@3.0.4
+ Addedbalanced-match@1.0.2(transitive)
+ Addedbrace-expansion@1.1.11(transitive)
+ Addedcommander@2.13.0(transitive)
+ Addedconcat-map@0.0.1(transitive)
+ Addedminimatch@3.0.4(transitive)
- Removedcommander@2.11.0(transitive)
Updatedcommander@2.13.0