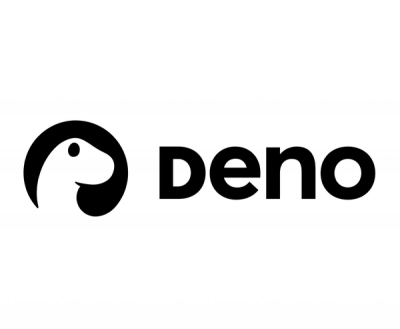
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
express-initialize
Advanced tools
A simple Express app initializer.
Install it with NPM or Yarn:
$ npm install --save express-initialize
$ yarn add express-initialize
Everything is done in the app.js file:
// app/app.js
const express = require('express');
const handlebars = require('express-handlebars');
const orm = require('orm');
const app = express();
const hbs = handlebars.create({
defaultLayout: 'main',
helpers: {
someFunction: () => { return 'Something'; }
},
// More configuration etc.
});
app.engine('handlebars', hbs.engine);
app.set('view engine', 'handlebars');
app.use(express.static('./static'));
app.use(compression());
app.get('/home', (request, response) => {
response.render('home');
});
app.get('/other-page', (request, response) => {
response.render('other-page');
});
orm.connect('mysql://root:password@host/database')
.then(connection => {
// etc.
})
.catch(console.error);
app.listen(80);
Everything is moved to separate files:
// app/app.js
const express = require('express');
const expressInitialize = require('express-initialize');
const app = express();
const initializers = getInitializers(); // Load the initializers
expressInitialize.initialize(app, initializers)
.then(() => {
app.listen(80);
})
.catch(console.error);
The function initialize
takes two arguments: the Express app that will be passed to the initializers and an array containing the initializers.
An initializer is an object which can have three properties:
Name | Type | Description |
---|---|---|
name | string | Name of the initializer, for usage with after . |
after | string or string[] | Name(s) of the initializer(s) after which the initializer should be run. |
configure | function | The function that will be run, takes app as argument and may return a Promise. |
For example, a file for Handlebars.
// app/initializers/handlebars.js
module.exports = {
configure: app => {
const hbs = handlebars.create({
defaultLayout: 'main',
helpers: {
someFunction: () => { return 'Something'; }
},
// More configuration etc.
});
app.engine('handlebars', hbs.engine);
app.set('view engine', 'handlebars');
}
};
You can also return a Promise.
// app/initializers/database.js
module.exports = {
configure: app => {
return new Promise((resolve, reject) => {
orm.connect('mysql://root:password@host/database')
.then(connection => {
// do something with connection
resolve();
})
.catch(reject);
});
}
}
Or specify what initializers have to run first.
// app/initializers/routes.js
module.exports = {
after: 'middleware',
configure: app => {
app.get('/home', (request, response) => {
response.render('home');
});
}
}
FAQs
A simple Express app initializer
The npm package express-initialize receives a total of 0 weekly downloads. As such, express-initialize popularity was classified as not popular.
We found that express-initialize demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.