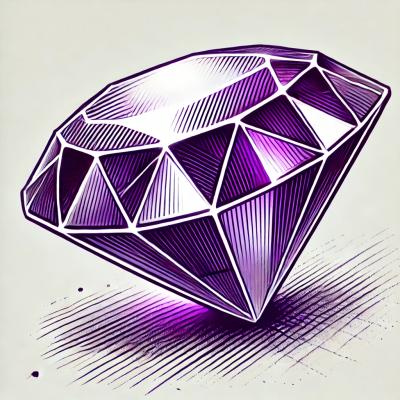
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
extra-fyers.web
Advanced tools
A Javascript interface for FYERS API.
š¦ Node.js,
š Web,
š Files,
š° Docs.
FYERS is one of the cheapest online stock brokers in India, that offers
trading in the equity (NSE, BSE), currency (NSE), and commodity segments (MCX).
The objective of this package is to provide a cleaner interface to FYERS API.
The http
namespace provides the same interface as FYERS HTTP API. The
websocket
namespace provides the same interface as FYERS WebSocket API, along
with parsing of binary market data. This allows you to recieve instant
notifications of order update and market data. The top namespace (global
functions, classes) provide a facade for the HTTP and the WebSocket APIs and
provides additional utility functions, such as calculating charges.
Global functions associated with FYERS API, such as getPositions()
, are
stateless and accept Authorization
as the first parameter. On the other
hand, the Api
class includes stateful functions which do not require
the Authorization
parameter (required while creating object). Note that
this authorization can be obtained be performing login with loginStep1()
and loginStep2()
.
The goals for the future include doing a thorough interface check, and possibly writing a CLI interface. Obtaining details of symbols, including images and more could be done as part of a separate package.
This package is available in both Node.js and Web formats. The web format
is exposed as extra_fyers
standalone variable and can be loaded from
jsDelivr CDN.
Stability: Experimental.
const fyers = require('extra-fyers');
async function main() {
var appId = '****'; // app_id recieved after creating app
var accessToken = '****'; // access_token recieved after login
var api = new fyers.Api(appId, accessToken);
// List equity and commodity fund limits.
console.log(await api.getFunds());
// List holdings.
console.log(await api.getHoldings());
// Place CNC market order for SBIN (equity) on NSE for 5 shares
var id = await api.placeOrder({symbol: 'NSE:SBIN-EQ', quantity: 5});
// List postions for today (should list NSE:SBIN-EQ-CNC).
console.log(await api.getPositions());
// Connect to Market data with WebSocket
// and recieve real-time market quotes.
await api.connectMarketData(quote => {
console.log(quote);
});
// Choose which symbols you want to subscribe to.
await api.subscribeMarketDepth(['NSE:SBIN-EQ']);
// Connect to Order update with WebSocket
// and recieve real-time order status updates.
await api.connectOrderUpdate(order => {
console.log(order);
});
// Subscribe to order status updates.
await api.subscribeOrderUpdate();
}
main();
Property | Description |
---|---|
exchangeDescription | Get exchange description. |
exchange | Get exchange code. |
segmentDescription | Get segment description. |
segment | Get segment code. |
positionSideDescription | Get position side description. |
positionSide | Get position side code. |
orderSideDescription | Get order side description. |
orderSide | Get order side code. |
orderSourceDescription | Get order source description. |
orderSource | Get order source code. |
orderStatusDescription | Get order status description. |
orderStatus | Get order status code. |
orderTypeDescription | Get order type description. |
orderType | Get order type code. |
orderValidityDescription | Get order validity description. |
orderValidity | Get order validity code. |
optionTypeDescription | Get option type description. |
optionType | Get option type code. |
derivativeTypeDescription | Get derivative type description. |
derivativeType | Get derivative type code. |
holdingTypeDescription | Get holding type description. |
holdingType | Get holding type code. |
productTypeDescription | Get product type description. |
productType | Get product type code. |
instrumentTypeDescription | Get instrument type description. |
instrumentType | Get instrument type code. |
symbolName | Get symbol exchange, underlying, currency-pair, or commodity name. |
symbolExchange | Get symbol exchange. |
symbolSeries | Get symbol exchange series. |
symbolOptionType | Get symbol option type. |
symbolDerivativeType | Get symbol derivative type. |
symbolStrikePrice | Get symbol strike price. |
symbolToken | Get symbol token, a unique identifier. |
symbolDescription | Get symbol description. |
symbolIsin | Get symbol ISIN. |
symbolLotSize | Get symbol minimum lot size. |
equityDeliveryCharges | Get equity delivery charges. |
equityIntradayCharges | Get equity intraday charges. |
equityFuturesCharges | Get equity futures charges. |
equityOptionsCharges | Get equity options charges. |
currencyFuturesCharges | Get currency futures charges. |
currencyOptionsCharges | Get currency options charges. |
commodityFuturesCharges | Get commodity futures charges. |
commodityOptionsCharges | Get commodity options charges. |
loginStep1 | Get request step 1 for authorization. |
loginStep2 | Get request step 2 for authorization. |
getProfile | Get basic details of the client. |
getFunds | Get balance available for the user for capital as well as the commodity market. |
getHoldings | Get the equity and mutual fund holdings which the user has in this demat account. |
getOrder | Get details of an order placed in the current trading day. |
getOrders | Get details of all the orders placed in the current trading day. |
getPositions | Get details of all the positions in the current trading day. |
getTrades | Get details of all the trades in the current trading day. |
placeOrder | Place an order to any exchange via Fyers. |
placeOrders | Place multiple orders to any exchange via Fyers. |
modifyOrder | Modifies an order placed on any exchange via Fyers. |
modifyOrders | Modifies orders placed on any exchange via Fyers. |
cancelOrder | Cancels an order placed on any exchange via Fyers. |
cancelOrders | Cancels orders placed on any exchange via Fyers. |
exitPosition | Exits a position on the current trading day. |
exitAllPositions | Exits all positions on the current trading day. |
convertPosition | Converts a position on the current trading day. |
getMarketStatus | Get the current market status of all the exchanges and their segments. |
getMarketHistory | Get the market history for a particular symbol. |
getMarketQuotes | Get the current market quotes for a set of symbols. |
getMarketDepth | Get the current market depth for a particular symbol. |
getSymbolMaster | Get all the latest symbols of all the exchanges from the symbol master files. |
processSymbolMaster | Get details of symbols from the symbol master file text. |
loadSymbolMaster | Get details of symbols from the symbol master files. |
generateEdisTpin | Generate e-DIS TPIN for validating/authorising transaction. |
getEdisTransactions | Get the necessary information regarding the holdings you have on your and also the status of the holdings. |
submitEdisHoldingsStep | Redirect to CDSL page for login where you can submit your Holdings information and accordingly you can provide the same to exchange to Sell your holdings (browser only). |
inquireEdisTransaction | Inquire the information/status of the provided transaction Id for the respective holdings you have on your end. |
connectMarketData | Connect to Market data URL with WebSocket. |
subscribeMarketQuote | Subscribe to market quote. |
subscribeMarketDepth | Subscribe to market depth. |
unsubscribeMarketQuote | Unsubscribe to market quote. |
unsubscribeMarketDepth | Unsubscribe to market depth. |
connectOrderUpdate | Connect to Order update URL with WebSocket. |
subscribeOrderUpdate | Subscribe to order update. |
unsubscribeOrderUpdate | Unsubscribe to order update. |
Api | Stateful interface for FYERS API. |
FAQs
A Javascript interface for FYERS API web.
The npm package extra-fyers.web receives a total of 14 weekly downloads. As such, extra-fyers.web popularity was classified as not popular.
We found that extra-fyers.web demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.Ā It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.