A map is a collection of key-value pairs, with unique keys.
:package: NPM,
:smiley_cat: GitHub,
:running: RunKit,
:vhs: Asciinema,
:moon: Minified,
:scroll: Files,
:newspaper: JSDoc,
:blue_book: Wiki.
All functions except from*()
take Map
as 1st parameter. Some names
are borrowed from Haskell, Python, Java, Processing.
Methods look like:
swap()
: doesn't modify the map itself (pure).swap$()
: modifies the map itself (update).
Methods as separate packages:
Stability: Experimental.
const map = require('extra-map');
var x = new Map([["a", 1], ["b", 2], ["c", 3], ["d", 4]]);
map.swap(x, "a", "b");
var x = new Map([["a", 1], ["b", 2], ["c", 3], ["d", 4]]);
var y = new Map([["b", 20], ["c", 30], ["e", 50]]);
map.intersection(x, y);
var x = new Map([["a", 1], ["b", 2], ["c", 3], ["d", -2]]);
map.searchAll(x, v => Math.abs(v) === 2);
var x = new Map([["a", 1], ["b", 2], ["c", 3]]);
[...map.subsets(x)];
Index
Method | Action |
---|
is | Checks if value is map. |
get | Gets value at key. |
set | Sets value at key. |
remove | Deletes an entry. |
swap | Exchanges two values. |
size | Gets size of map. |
| |
head | Gets first entry. |
take | Keeps first n entries only. |
shift | Removes first entry. |
from | Creates map from entries. |
| |
concat | Appends entries from maps, preferring last. |
flat | Flattens nested map to given depth. |
chunk | Breaks map into chunks of given size. |
filterAt | Gets map with given keys. |
| |
map | Updates values based on map function. |
filter | Keeps entries which pass a test. |
reduce | Reduces values to a single value. |
range | Finds smallest and largest entries. |
count | Counts values which satisfy a test. |
partition | Segregates values by test result. |
cartesianProduct | Lists cartesian product of maps. |
some | Checks if any value satisfies a test. |
zip | Combines matching entries from maps. |
| |
union | Gives entries present in any map. |
intersection | Gives entries present in both maps. |
difference | Gives entries of map not present in another. |
symmetricDifference | Gives entries not present in both maps. |
isDisjoint | Checks if maps have no common keys. |
| |
key | Picks an arbitrary key. |
value | Picks an arbitrary value. |
entry | Picks an arbitrary entry. |
subset | Picks an arbitrary subset. |
| |
isEmpty | Checks if map is empty. |
isEqual | Checks if two maps are equal. |
compare | Compares two maps. |
find | Finds a value passing a test. |
search | Finds key of an entry passing a test. |
scanWhile | Finds key of first entry not passing a test. |
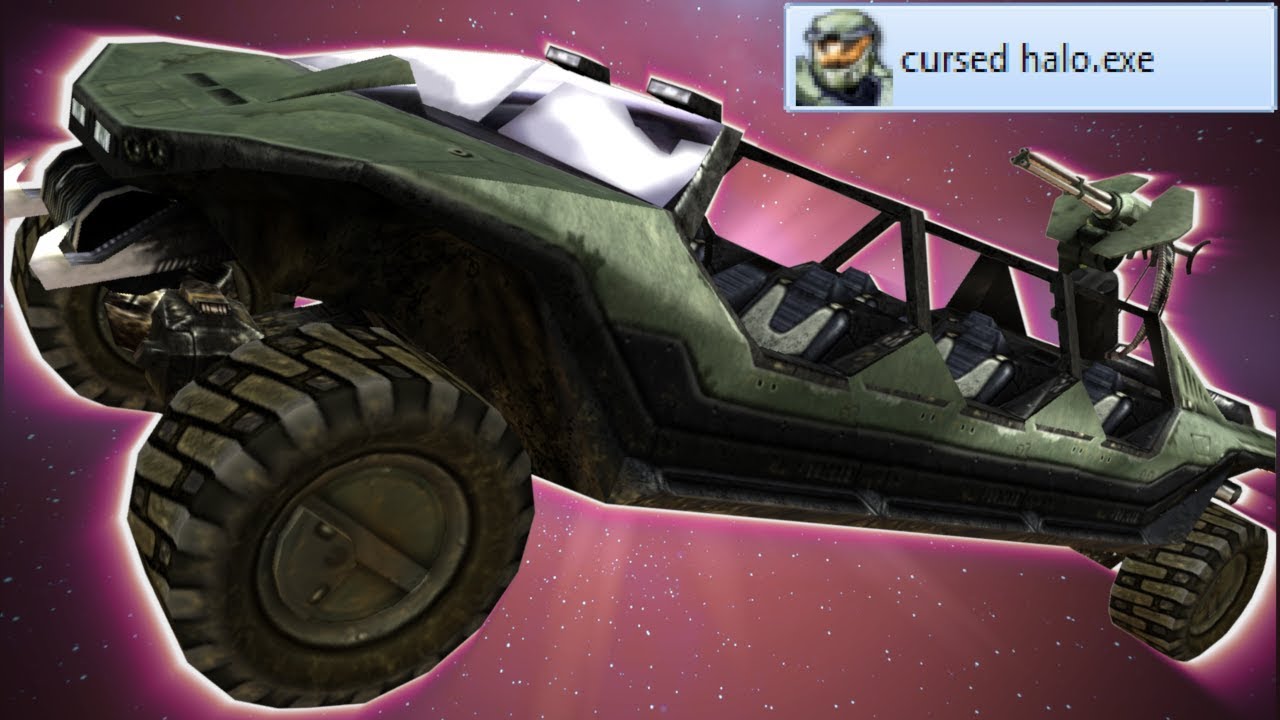