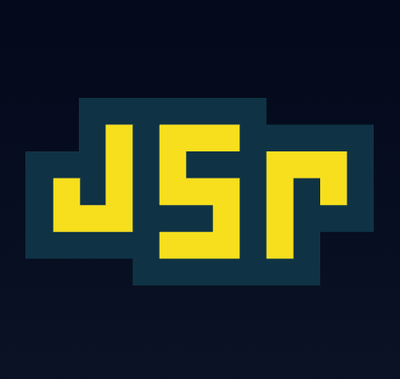
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
factory-girl
Advanced tools
factory-girl
is a factory library for Node.js / JavaScript inspired by Factory_girl. It works asynchronously and supports lazy attributes as well as associations.
It is based on factory-lady, but uses an adapter to talk to your models (and doesn't use throw
for errors that might occur during save).
Node.js:
npm install factory-girl
To use factory-girl
in the browser or other JavaScript environments, just include index.js
.
JavaScript:
var factory = require('factory-lady'),
User = require('../../app/models/user'),
Post = require('../../app/models/post');
var emailCounter = 1;
factory.define('user', User, {
email : function() { return 'user' + emailCounter++ + '@example.com'; }, // lazy attribute
async : function(cb) { somethingAsync(cb); }, // async lazy attribute
state : 'activated',
password : '123456'
});
factory.define('post', Post, {
user_id : factory.assoc('user', 'id') // simply factory.assoc('user') for user object itself,
subject : 'Hello World',
content : 'Lorem ipsum dolor sit amet...'
});
JavaScript:
factory.build('post', function(err, post) {
// post is a Post instance that is not saved
});
factory.build('post', {title: 'Foo', content: 'Bar'}, function(err, post) {
// build a post and override title and content
});
factory.create('post', function(err, post) {
// post is a saved Post instance
});
factory('post', function(err, post) {
// same as factory.create
});
Copyright (c) 2011 Peter Jihoon Kim. This software is licensed under the MIT License. Copyright (c) 2014 Simon Wade. This software is licensed under the MIT License.
FAQs
A factory library for Node.js and JavaScript inspired by factory_girl
The npm package factory-girl receives a total of 30,586 weekly downloads. As such, factory-girl popularity was classified as popular.
We found that factory-girl demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.