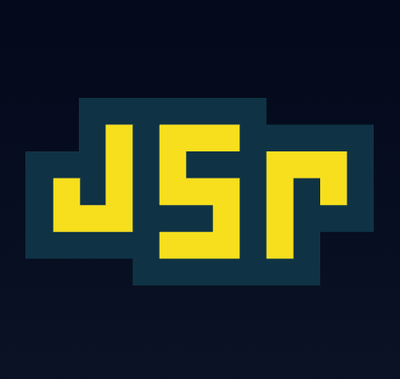
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
factory-girl
Advanced tools
factory-girl
is a factory library for Node.js and the browser that is inspired by Factory_girl. It works asynchronously and supports associations and the use of functions for generating attributes.
It started out as a fork of factory-lady, but the fork deviated quite a bit. This module uses an adapter to talk to your models so it can support different ORMs such as Bookshelf, Sequelize, JugglingDB, and Mongoose (and doesn't use throw
for errors that might occur during save).
Node.js:
npm install factory-girl
To use factory-girl
in the browser or other JavaScript environments, just include index.js
and access window.Factory
.
var factory = require('factory-girl'),
User = require('../../app/models/user'),
Post = require('../../app/models/post');
var emailCounter = 1;
// define a factory using define()
factory.define('user', User, {
// define attributes using properties
state: 'active',
// ...or functions
email: function() {
return 'user' + emailCounter++ + '@demo.com';
},
// provide async functions by accepting a callback
async: function(callback) {
somethingAsync(callback);
}
});
factory.build('user', function(err, user) {
console.log(user.attributes); // => {state: 'active', email: 'user1@demo.com', async: 'foo'}
));
factory.define('comment', Comment, {
text: 'hello'
});
factory.define('post', Post, {
// create associations using factory.assoc(model, key)
// or factory.assoc('user') to return the user object itself.
user_id: factory.assoc('user', 'id'),
// create array of associations using factory.assocMany(model, key, num)
comments: factory.assocMany('comment', 'text', 2)
subject: 'Hello World',
// you can refer to other attributes using `this`
slug: function() {
return slugify(this.subject);
}
});
factory.build('post', function(err, post) {
console.log(post.attributes); // => {user_id: 1, comments: [{ text: 'hello' }, { text: 'hello' }],
// subject: 'Hello World', slug: 'hello-world'}
));
factory.build('post', function(err, post) {
// post is a Post instance that is not saved
});
factory.build('post', {title: 'Foo', content: 'Bar'}, function(err, post) {
// build a post and override title and content
});
factory.create('post', function(err, post) {
// post is a saved Post instance
});
You can optionally provide attributes to the associated factory by passing an object as third argument.
Allow you to create a number of models at once.
factory.buildMany('post', 10, function(err, posts) {
// build 10 posts
});
factory.buildMany('post', [{title: 'Foo'}, {title: 'Bar'}], function(err, posts) {
// build 2 posts using the specified attributes
});
factory.buildMany('post', [{title: 'Foo'}, {title: 'Bar'}], 10, function(err, posts) {
// build 10 posts using the specified attributes for the first and second
});
factory.buildMany('post', {title: 'Foo'}, 10, function(err, posts) {
// build 10 posts using the specified attributes for all of them
});
factory.createMany
takes the same arguments as buildMany
, but returns saved models.
When you have factories that don't use async property functions, you can use buildSync()
.
Be aware that assoc()
is an async function, so it can't be used with buildSync()
.
var doc = factory.buildSync('post', {title: 'Foo'});
Adapters provide support for different databases and ORMs.
var anotherFactory = new factory.Factory();
var BookshelfAdapter = require('factory-girl-bookshelf').BookshelfAdapter;
anotherFactory.setAdapter(BookshelfAdapter); // use the Bookshelf adapter
Or use the ObjectAdapter that simply returns raw objects.
var ObjectAdapter = factory.ObjectAdapter;
anotherFactory.setAdapter(ObjectAdapter, 'post'); // use the ObjectAdapter for posts
Copyright (c) 2014 Simon Wade. This software is licensed under the MIT License.
Copyright (c) 2011 Peter Jihoon Kim. This software is licensed under the MIT License.
FAQs
A factory library for Node.js and JavaScript inspired by factory_girl
The npm package factory-girl receives a total of 30,586 weekly downloads. As such, factory-girl popularity was classified as popular.
We found that factory-girl demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.