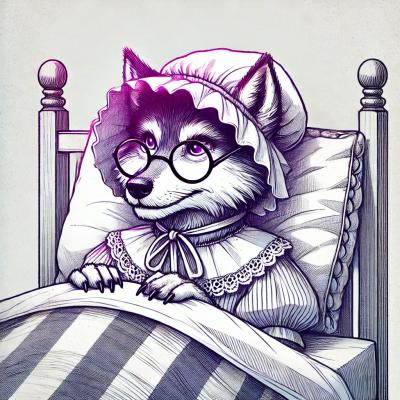
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
fake-indexeddb
Advanced tools
Fake IndexedDB: a pure JS in-memory implementation of the IndexedDB API
The fake-indexeddb npm package is a mock implementation of the IndexedDB API, which is useful for testing and development purposes. It allows developers to simulate IndexedDB operations without relying on a real browser environment.
Creating a Database
This feature allows you to create a new IndexedDB database and an object store within it. The code sample demonstrates how to open a database, handle the upgrade event to create an object store, and log the success event.
const { indexedDB } = require('fake-indexeddb');
const request = indexedDB.open('test-db', 1);
request.onupgradeneeded = function(event) {
const db = event.target.result;
db.createObjectStore('store', { keyPath: 'id' });
};
request.onsuccess = function(event) {
const db = event.target.result;
console.log('Database created:', db);
};
Adding Data to Object Store
This feature allows you to add data to an object store within the IndexedDB database. The code sample demonstrates how to open a database, create a transaction, add data to the object store, and log the completion of the transaction.
const { indexedDB } = require('fake-indexeddb');
const request = indexedDB.open('test-db', 1);
request.onsuccess = function(event) {
const db = event.target.result;
const transaction = db.transaction('store', 'readwrite');
const store = transaction.objectStore('store');
store.add({ id: 1, name: 'John Doe' });
transaction.oncomplete = function() {
console.log('Data added');
};
};
Retrieving Data from Object Store
This feature allows you to retrieve data from an object store within the IndexedDB database. The code sample demonstrates how to open a database, create a transaction, retrieve data from the object store, and log the retrieved data.
const { indexedDB } = require('fake-indexeddb');
const request = indexedDB.open('test-db', 1);
request.onsuccess = function(event) {
const db = event.target.result;
const transaction = db.transaction('store', 'readonly');
const store = transaction.objectStore('store');
const getRequest = store.get(1);
getRequest.onsuccess = function() {
console.log('Data retrieved:', getRequest.result);
};
};
The indexeddbshim package is a polyfill that provides a full implementation of the IndexedDB API for browsers that do not support it natively. Unlike fake-indexeddb, which is primarily for testing, indexeddbshim aims to provide a real IndexedDB experience in environments where it is not available.
LocalForage is a library that provides a simple API for offline storage, using IndexedDB, WebSQL, or localStorage under the hood. It abstracts away the differences between these storage mechanisms, making it easier to use. While fake-indexeddb is focused on testing, LocalForage is designed for actual application use.
Dexie is a wrapper library for IndexedDB that provides a more developer-friendly API and additional features like versioning and schema management. It is designed for real-world use in applications, whereas fake-indexeddb is intended for testing purposes.
This is a pure JS in-memory implementation of the IndexedDB API.
It passes the W3C IndexedDB test suite (a feat that all browsers except Chrome fail) plus a couple hundred more tests just to be sure. It also works well enough to run fairly complex IndexedDB-based software.
For use with CommonJS (Node.js/Browserify), install through npm:
npm install fake-indexeddb
Otherwise, download the bundled version and include it in your page like:
<script type="text/javascript" src="fakeIndexedDB.js"></script>
If you're using AMD, you'll have to shim it.
Functionally, it works exactly like IndexedDB except data is not persisted to disk.
Example usage:
var fakeIndexedDB = require('fake-indexeddb');
var FDBKeyRange = require('fake-indexeddb/lib/FDBKeyRange');
var request = fakeIndexedDB.open('test', 3);
request.onupgradeneeded = function () {
var db = request.result;
var store = db.createObjectStore("books", {keyPath: "isbn"});
store.createIndex("by_title", "title", {unique: true});
store.put({title: "Quarry Memories", author: "Fred", isbn: 123456});
store.put({title: "Water Buffaloes", author: "Fred", isbn: 234567});
store.put({title: "Bedrock Nights", author: "Barney", isbn: 345678});
}
request.onsuccess = function (event) {
var db = event.target.result;
var tx = db.transaction("books");
tx.objectStore("books").index("by_title").get("Quarry Memories").addEventListener('success', function (event) {
console.log('From index:', event.target.result);
});
tx.objectStore("books").openCursor(FDBKeyRange.lowerBound(200000)).onsuccess = function (event) {
var cursor = event.target.result;
if (cursor) {
console.log('From cursor:', cursor.value);
cursor.continue();
}
};
tx.oncomplete = function () {
console.log('All done!');
};
};
Variable names of all the objects are like the normal IndexedDB ones except with F replacing I, e.g. FDBIndex
instead of IDBIndex
.
If you're using the bundled version (not installed through npm), then all of the variables are created and attached to window
(or self
in a Web Worker), like window.fakeIndexedDB
, window.FDBKeyRange
, etc.
Use as a mock database in unit tests.
Use the same API in Node.js and in the browser.
Support IndexedDB in old or crappy browsers.
Somehow use it within a caching layer on top of IndexedDB in the browser, since IndexedDB can be kind of slow.
Abstract the core database functions out, so what is left is a shell that allows the IndexedDB API to easily sit on top of many different backends.
Serve as a playground for experimenting with IndexedDB.
Apache 2.0
FAQs
Fake IndexedDB: a pure JS in-memory implementation of the IndexedDB API
The npm package fake-indexeddb receives a total of 295,517 weekly downloads. As such, fake-indexeddb popularity was classified as popular.
We found that fake-indexeddb demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.