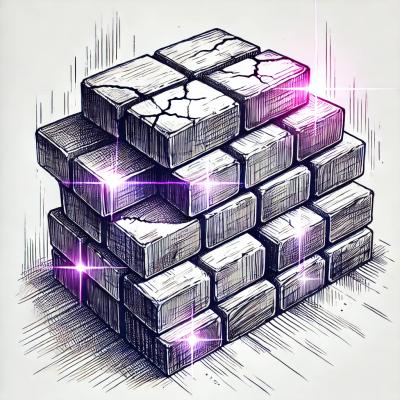
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
faster-computing
Advanced tools
Small Node.js lib.
npm i --save faster-computing
This package is intended for multiprocessing. If your computing device supports parallelisation of the processes, then this module may speedup your daily data computation. It does a very simple task - takes your code and data, splits the data into the batches, replicates your code into the tmp file, forks the process your-machine-cpu-threads-number times, and then each child process executes the code for the specific batch of data. If your data processing takes a very small amount of time (e.g, milliseconds), you may not find it useful, but if you are working on big data and you have to do many computations, this module should be helpful.
const FasterComputing = require('faster-computing');
const fc = new FasterComputing();
const dataSource = {
data: [1, 2, 3, 4]
};
function computeProcedure(params) {
const { data, foo, bar } = params;
return data.map(val => val + 1);
}
const computeProcedureParams = {
foo: 1,
bar: 2
};
const memory = 25;
// First version of calling the `compute` method.
(async () => {
try {
const data = await fc.compute(dataSource, computeProcedure, computeProcedureParams, memory);
} catch (err) { ... }
})();
// Second version of calling the `compute` method.
fc.compute(dataSource, computeProcedure, computeProcedureParams, memory)
.then(data => { ... })
.catch(err => { ... });
Another example
const FasterComputing = require('faster-computing');
const fc = new FasterComputing();
const mongoose = require('mongoose');
const path = require('path');
const System = require(path.join(__dirname, '/../models/system'));
await mongoose.connect('mongodb://localhost:27017/foo-bar');
const dataSource = {
data: await System.find({ }).limit(1e5)
};
async function computeProcedure(params) {
const { data, $__dirname } = params;
const path = require('path');
const { someAsyncFunction, someSyncFunction } = require(path.join($__dirname, '/../../subroutines'));
const results = [];
for (let item of data) {
const result = await someAsyncFunction(item);
results.push(someSyncFunction(result));
}
return results;
}
const computeProcedureParams = {
$__dirname: __dirname
};
const memory = 3500;
(async () => {
try {
const data = await fc.compute(dataSource, computeProcedure, computeProcedureParams, memory);
} catch (err) { ... }
})();
compute
method.data
or dataFetchProcedure
(this property is not implemented yet).
params
(which contains computeProcedureParams
(third parameter)).
data
, which will be a specific batch of your data.data
is reserved and if you pass this key it will throw an
invalid_params
error. You can access these params in computeProcedure
like this:
const { data, foo, bar, baz } = params;
. Also consider that computeProcedure
's scope is
this module's scope and if you want to access some file in your local directory using __dirname
you must pass this param for example like this { $__dirname: __dirname }
.MIT
FAQs
Package for faster processing.
We found that faster-computing demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.