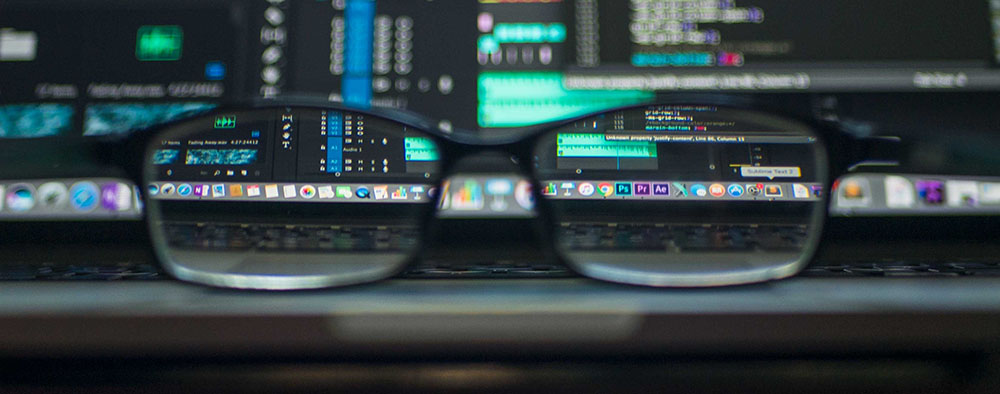

fastest-validator

:zap: The fastest JS validator library for NodeJS | Browser | Deno.
Key features
- blazing fast! Really!
- 20+ built-in validators
- many sanitizations
- custom validators & aliases
- nested objects & array handling
- strict object validation
- multiple validators
- customizable error messages
- programmable error object
- no dependencies
- unit tests & 100% coverage
How fast?
Very fast! 8 million validations/sec (on Intel i7-4770K, Node.JS: 12.14.1)
√ validate 8,678,752 rps
Compared to other popular libraries:
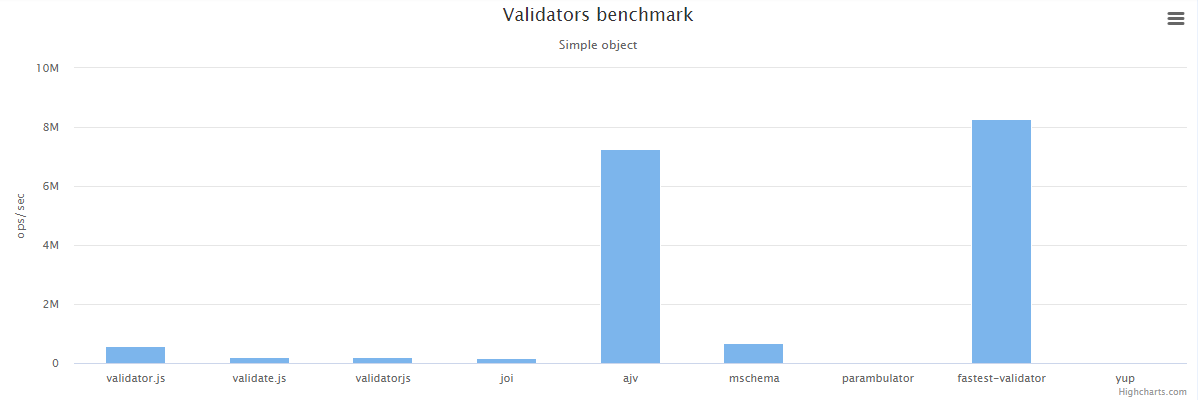
50x faster than Joi.
Would you like to test it?
$ git clone https://github.com/icebob/fastest-validator.git
$ cd fastest-validator
$ npm install
$ npm run bench
Installation
NPM
You can install it via NPM.
$ npm i fastest-validator --save
or
$ yarn add fastest-validator
Usage
Validate
The first step is to compile the schema to a compiled "checker" function. After that, to validate your object, just call this "checker" function.
This method is the fastest.
const Validator = require("fastest-validator");
const v = new Validator();
const schema = {
id: { type: "number", positive: true, integer: true },
name: { type: "string", min: 3, max: 255 },
status: "boolean"
};
const check = v.compile(schema);
console.log("First:", check({ id: 5, name: "John", status: true }));
console.log("Second:", check({ id: 2, name: "Adam" }));
Try it on Repl.it
Browser usage
<script src="https://unpkg.com/fastest-validator"></script>
const v = new FastestValidator();
const schema = {
id: { type: "number", positive: true, integer: true },
name: { type: "string", min: 3, max: 255 },
status: "boolean"
};
const check = v.compile(schema);
console.log(check({ id: 5, name: "John", status: true }));
Deno usage
With esm.sh
, now Typescript is supported
import FastestValidator from "https://esm.sh/fastest-validator@1"
const v = new FastestValidator();
const check = v.compile({
name: "string",
age: "number",
});
console.log(check({ name: "Erf", age: 18 }));
Supported frameworks
- Moleculer: Natively supported
- Fastify: By using fastify-fv
Optional, Required & Nullable fields
Optional
Every field in the schema will be required by default. If you'd like to define optional fields, set optional: true
.
const schema = {
name: { type: "string" },
age: { type: "number", optional: true }
}
v.validate({ name: "John", age: 42 }, schema);
v.validate({ name: "John" }, schema);
v.validate({ age: 42 }, schema);
Nullable
If you want disallow undefined
value but allow null
value, use nullable
instead of optional
.
const schema = {
age: { type: "number", nullable: true }
}
v.validate({ age: 42 }, schema);
v.validate({ age: null }, schema);
v.validate({ age: undefined }, schema);
v.validate({}, schema);
Strict validation
Object properties which are not specified on the schema are ignored by default. If you set the $$strict
option to true
any additional properties will result in an strictObject
error.
const schema = {
name: { type: "string" },
$$strict: true
}
v.validate({ name: "John" }, schema);
v.validate({ name: "John", age: 42 }, schema);
Remove additional fields
To remove the additional fields in the object, set $$strict: "remove"
.
Multiple validators
It is possible to define more validators for a field. In this case, only one validator needs to succeed for the field to be valid.
const schema = {
cache: [
{ type: "string" },
{ type: "boolean" }
]
}
v.validate({ cache: true }, schema);
v.validate({ cache: "redis://" }, schema);
v.validate({ cache: 150 }, schema);
Root element schema
Basically the validator expects that you want to validate a Javascript object. If you want others, you can define the root level schema, as well. In this case set the $$root: true
property.
Example to validate a string
variable instead of object
const schema = {
$$root: true,
type: "string",
min: 3,
max: 6
};
v.validate("John", schema);
v.validate("Al", schema);
Sanitizations
The library contains several sanitizers. Please note, the sanitizers change the original checked object.
Default values
The most common sanitizer is the default
property. With it, you can define a default value for all properties. If the property value is null
or undefined
, the validator set the defined default value into the property.
Static Default value example:
const schema = {
roles: { type: "array", items: "string", default: ["user"] },
status: { type: "boolean", default: true },
};
const obj = {}
v.validate(obj, schema);
console.log(obj);
Dynamic Default value:
Also you can use dynamic default value by defining a function that returns a value. For example, in the following code, if createdAt
field not defined in object`, the validator sets the current time into the property:
const schema = {
createdAt: {
type: "date",
default: () => new Date()
}
};
const obj = {}
v.validate(obj, schema);
console.log(obj);
Shorthand definitions
You can use string-based shorthand validation definitions in the schema.
const schema = {
password: "string|min:6",
age: "number|optional|integer|positive|min:0|max:99",
state: ["boolean", "number|min:0|max:1"]
}
Array of X
const schema = {
foo: "string[]"
}
check({ foo: ["bar"] })
Nested objects
const schema = {
dot: {
$$type: "object",
x: "number",
y: "number",
},
circle: {
$$type: "object|optional",
o: {
$$type: "object",
x: "number",
y: "number",
},
r: "number"
}
};
Alias definition
You can define custom aliases.
v.alias('username', {
type: 'string',
min: 4,
max: 30
});
const schema = {
username: "username|max:100",
password: "string|min:6",
}
Default options
You can set default rule options.
const v = new FastestValidator({
defaults: {
object: {
strict: "remove"
}
}
});
Built-in validators
any
This does not do type validation. Accepts any types.
const schema = {
prop: { type: "any" }
}
v.validate({ prop: true }, schema);
v.validate({ prop: 100 }, schema);
v.validate({ prop: "John" }, schema);
array
This is an Array
validator.
Simple example with strings:
const schema = {
roles: { type: "array", items: "string" }
}
v.validate({ roles: ["user"] }, schema);
v.validate({ roles: [] }, schema);
v.validate({ roles: "user" }, schema);
Example with only positive numbers:
const schema = {
list: { type: "array", min: 2, items: {
type: "number", positive: true, integer: true
} }
}
v.validate({ list: [2, 4] }, schema);
v.validate({ list: [1, 5, 8] }, schema);
v.validate({ list: [1] }, schema);
v.validate({ list: [1, -7] }, schema);
Example with an object list:
const schema = {
users: { type: "array", items: {
type: "object", props: {
id: { type: "number", positive: true },
name: { type: "string", empty: false },
status: "boolean"
}
} }
}
v.validate({
users: [
{ id: 1, name: "John", status: true },
{ id: 2, name: "Jane", status: true },
{ id: 3, name: "Bill", status: false }
]
}, schema);
Example for enum
:
const schema = {
roles: { type: "array", items: "string", enum: [ "user", "admin" ] }
}
v.validate({ roles: ["user"] }, schema);
v.validate({ roles: ["user", "admin"] }, schema);
v.validate({ roles: ["guest"] }, schema);
Example for unique
:
const schema = {
roles: { type: "array", unique: true }
}
v.validate({ roles: ["user"] }, schema);
v.validate({ roles: [{role:"user"},{role:"admin"},{role:"user"}] }, schema);
v.validate({ roles: ["user", "admin", "user"] }, schema);
v.validate({ roles: [1, 2, 1] }, schema);
Properties
Property | Default | Description |
---|
empty | true | If true , the validator accepts an empty array [] . |
min | null | Minimum count of elements. |
max | null | Maximum count of elements. |
length | null | Fix count of elements. |
contains | null | The array must contain this element too. |
unique | null | The array must be unique (array of objects is always unique). |
enum | null | Every element must be an element of the enum array. |
items | null | Schema for array items. |
boolean
This is a Boolean
validator.
const schema = {
status: { type: "boolean" }
}
v.validate({ status: true }, schema);
v.validate({ status: false }, schema);
v.validate({ status: 1 }, schema);
v.validate({ status: "true" }, schema);
Properties
Property | Default | Description |
---|
convert | false | if true and the type is not Boolean , it will be converted. 1 , "true" , "1" , "on" will be true. 0 , "false" , "0" , "off" will be false. It's a sanitizer, it will change the value in the original object. |
Example for convert
:
v.validate({ status: "true" }, {
status: { type: "boolean", convert: true}
});
class
This is a Class
validator to check the value is an instance of a Class.
const schema = {
rawData: { type: "class", instanceOf: Buffer }
}
v.validate({ rawData: Buffer.from([1, 2, 3]) }, schema);
v.validate({ rawData: 100 }, schema);
Properties
Property | Default | Description |
---|
instanceOf | null | Checked Class. |
currency
This is a Currency
validator to check if the value is a valid currency string.
const schema = {
money_amount: { type: "currency", currencySymbol: '$' }
}
v.validate({ money_amount: '$12.99'}, schema);
v.validate({ money_amount: '$0.99'}, schema);
v.validate({ money_amount: '$12,345.99'}, schema);
v.validate({ money_amount: '$123,456.99'}, schema);
v.validate({ money_amount: '$1234,567.99'}, schema);
v.validate({ money_amount: '$1,23,456.99'}, schema);
v.validate({ money_amount: '$12,34.5.99' }, schema);
Properties
Property | Default | Description |
---|
currencySymbol | null | The currency symbol expected in string (as prefix). |
symbolOptional | false | Toggle to make the symbol optional in string, although, if present it would only allow the currencySymbol. |
thousandSeparator | , | Thousand place separator character. |
decimalSeparator | . | Decimal place character. |
customRegex | null | Custom regular expression, to validate currency strings (For eg: /[0-9]*/g). |
date
This is a Date
validator.
const schema = {
dob: { type: "date" }
}
v.validate({ dob: new Date() }, schema);
v.validate({ dob: new Date(1488876927958) }, schema);
v.validate({ dob: 1488876927958 }, schema);
Properties
Property | Default | Description |
---|
convert | false | if true and the type is not Date , try to convert with new Date() . It's a sanitizer, it will change the value in the original object. |
Example for convert
:
v.validate({ dob: 1488876927958 }, {
dob: { type: "date", convert: true}
});
email
This is an e-mail address validator.
const schema = {
email: { type: "email" }
}
v.validate({ email: "john.doe@gmail.com" }, schema);
v.validate({ email: "james.123.45@mail.co.uk" }, schema);
v.validate({ email: "abc@gmail" }, schema);
Properties
Property | Default | Description |
---|
empty | false | If true , the validator accepts an empty array "" . |
mode | quick | Checker method. Can be quick or precise . |
normalize | false | Normalize the e-mail address (trim & lower-case). It's a sanitizer, it will change the value in the original object. |
min | null | Minimum value length. |
max | null | Maximum value length. |
enum
This is an enum validator.
const schema = {
sex: { type: "enum", values: ["male", "female"] }
}
v.validate({ sex: "male" }, schema);
v.validate({ sex: "female" }, schema);
v.validate({ sex: "other" }, schema);
Properties
Property | Default | Description |
---|
values | null | The valid values. |
equal
This is an equal value validator. It checks a value with a static value or with another property.
Example with static value:
const schema = {
agreeTerms: { type: "equal", value: true, strict: true }
}
v.validate({ agreeTerms: true }, schema);
v.validate({ agreeTerms: false }, schema);
Example with other field:
const schema = {
password: { type: "string", min: 6 },
confirmPassword: { type: "equal", field: "password" }
}
v.validate({ password: "123456", confirmPassword: "123456" }, schema);
v.validate({ password: "123456", confirmPassword: "pass1234" }, schema);
Properties
Property | Default | Description |
---|
value | undefined | The expected value. It can be any primitive types. |
strict | false | if true , it uses strict equal === for checking. |
forbidden
This validator returns an error if the property exists in the object.
const schema = {
password: { type: "forbidden" }
}
v.validate({ user: "John" }, schema);
v.validate({ user: "John", password: "pass1234" }, schema);
Properties
Property | Default | Description |
---|
remove | false | If true , the value will be removed in the original object. It's a sanitizer, it will change the value in the original object. |
Example for remove
:
const schema = {
user: { type: "string" },
token: { type: "forbidden", remove: true }
};
const obj = {
user: "John",
token: "123456"
}
v.validate(obj, schema);
console.log(obj);
function
This a Function
type validator.
const schema = {
show: { type: "function" }
}
v.validate({ show: function() {} }, schema);
v.validate({ show: Date.now }, schema);
v.validate({ show: "function" }, schema);
luhn
This is an Luhn validator.
Luhn algorithm checksum
Credit Card numbers, IMEI numbers, National Provider Identifier numbers and others
const schema = {
cc: { type: "luhn" }
}
v.validate({ cc: "452373989901198" }, schema);
v.validate({ cc: 452373989901198 }, schema);
v.validate({ cc: "4523-739-8990-1198" }, schema);
v.validate({ cc: "452373989901199" }, schema);
mac
This is an MAC addresses validator.
const schema = {
mac: { type: "mac" }
}
v.validate({ mac: "01:C8:95:4B:65:FE" }, schema);
v.validate({ mac: "01:c8:95:4b:65:fe", schema);
v.validate({ mac: "01C8.954B.65FE" }, schema);
v.validate({ mac: "01c8.954b.65fe", schema);
v.validate({ mac: "01-C8-95-4B-65-FE" }, schema);
v.validate({ mac: "01-c8-95-4b-65-fe" }, schema);
v.validate({ mac: "01C8954B65FE" }, schema);
multi
This is a multiple definitions validator.
const schema = {
status: { type: "multi", rules: [
{ type: "boolean" },
{ type: "number" }
], default: true }
}
v.validate({ status: true }, schema);
v.validate({ status: false }, schema);
v.validate({ status: 1 }, schema);
v.validate({ status: 0 }, schema);
v.validate({ status: "yes" }, schema);
Shorthand multiple definitions:
const schema = {
status: [
"boolean",
"number"
]
}
v.validate({ status: true }, schema);
v.validate({ status: false }, schema);
v.validate({ status: 1 }, schema);
v.validate({ status: 0 }, schema);
v.validate({ status: "yes" }, schema);
number
This is a Number
validator.
const schema = {
age: { type: "number" }
}
v.validate({ age: 123 }, schema);
v.validate({ age: 5.65 }, schema);
v.validate({ age: "100" }, schema);
Properties
Property | Default | Description |
---|
min | null | Minimum value. |
max | null | Maximum value. |
equal | null | Fixed value. |
notEqual | null | Can't be equal to this value. |
integer | false | The value must be a non-decimal value. |
positive | false | The value must be greater than zero. |
negative | false | The value must be less than zero. |
convert | false | if true and the type is not Number , it's converted with Number() . It's a sanitizer, it will change the value in the original object. |
object
This is a nested object validator.
const schema = {
address: { type: "object", strict: true, props: {
country: { type: "string" },
city: "string",
zip: "number"
} }
}
v.validate({
address: {
country: "Italy",
city: "Rome",
zip: 12345
}
}, schema);
v.validate({
address: {
country: "Italy",
city: "Rome"
}
}, schema);
v.validate({
address: {
country: "Italy",
city: "Rome",
zip: 12345,
state: "IT"
}
}, schema);
Properties
Property | Default | Description |
---|
strict | false | If true any properties which are not defined on the schema will throw an error. If remove all additional properties will be removed from the original object. It's a sanitizer, it will change the original object. |
minProps | null | If set to a number N, will throw an error if the object has fewer than N properties. |
maxProps | null | If set to a number N, will throw an error if the object has more than N properties. |
let schema = {
address: { type: "object", strict: "remove", props: {
country: { type: "string" },
city: "string",
zip: "number"
} }
}
let obj = {
address: {
country: "Italy",
city: "Rome",
zip: 12345,
state: "IT"
}
};
v.validate(obj, schema);
console.log(obj);
schema = {
address: {
type: "object",
minProps: 2,
props: {
country: { type: "string" },
city: { type: "string", optional: true },
zip: { type: "number", optional: true }
}
}
}
obj = {
address: {
country: "Italy",
city: "Rome",
zip: 12345,
state: "IT"
}
}
v.validate(obj, schema);
obj = {
address: {
country: "Italy",
}
}
v.validate(obj, schema);
string
This is a String
validator.
const schema = {
name: { type: "string" }
}
v.validate({ name: "John" }, schema);
v.validate({ name: "" }, schema);
v.validate({ name: 123 }, schema);
Properties
Property | Default | Description |
---|
empty | true | If true , the validator accepts an empty string "" . |
min | null | Minimum value length. |
max | null | Maximum value length. |
length | null | Fixed value length. |
pattern | null | Regex pattern. |
contains | null | The value must contain this text. |
enum | null | The value must be an element of the enum array. |
alpha | null | The value must be an alphabetic string. |
numeric | null | The value must be a numeric string. |
alphanum | null | The value must be an alphanumeric string. |
alphadash | null | The value must be an alphabetic string that contains dashes. |
hex | null | The value must be a hex string. |
singleLine | null | The value must be a single line string. |
base64 | null | The value must be a base64 string. |
trim | null | If true , the value will be trimmed. It's a sanitizer, it will change the value in the original object. |
trimLeft | null | If true , the value will be left trimmed. It's a sanitizer, it will change the value in the original object. |
trimRight | null | If true , the value will be right trimmed. It's a sanitizer, it will change the value in the original object. |
padStart | null | If it's a number, the value will be left padded. It's a sanitizer, it will change the value in the original object. |
padEnd | null | If it's a number, the value will be right padded. It's a sanitizer, it will change the value in the original object. |
padChar | " " | The padding character for the padStart and padEnd . |
lowercase | null | If true , the value will be lower-cased. It's a sanitizer, it will change the value in the original object. |
uppercase | null | If true , the value will be upper-cased. It's a sanitizer, it will change the value in the original object. |
localeLowercase | null | If true , the value will be locale lower-cased. It's a sanitizer, it will change the value in the original object. |
localeUppercase | null | If true , the value will be locale upper-cased. It's a sanitizer, it will change the value in the original object. |
convert | false | if true and the type is not a String , it's converted with String() . It's a sanitizer, it will change the value in the original object. |
Sanitization example
const schema = {
username: { type: "string", min: 3, trim: true, lowercase: true}
}
const obj = {
username: " Icebob "
};
v.validate(obj, schema);
console.log(obj);
tuple
This validator checks if a value is an Array
with the elements order as described by the schema.
Simple example:
const schema = { list: "tuple" };
v.validate({ list: [] }, schema);
v.validate({ list: [1, 2] }, schema);
v.validate({ list: ["RON", 100, true] }, schema);
v.validate({ list: 94 }, schema);
Example with items:
const schema = {
grade: { type: "tuple", items: ["string", "number"] }
}
v.validate({ grade: ["David", 85] }, schema);
v.validate({ grade: [85, "David"] }, schema);
v.validate({ grade: ["Cami"] }, schema);
Example with a more detailed schema:
const schema = {
location: { type: "tuple", items: [
"string",
{ type: "tuple", empty: false, items: [
{ type: "number", min: 35, max: 45 },
{ type: "number", min: -75, max: -65 }
] }
] }
}
v.validate({ location: ['New York', [40.7127281, -74.0060152]] }, schema);
v.validate({ location: ['New York', [50.0000000, -74.0060152]] }, schema);
v.validate({ location: ['New York', []] }, schema);
Properties
Property | Default | Description |
---|
empty | true | If true , the validator accepts an empty array [] . |
items | undefined | Exact schema of the value items |
url
This is an URL validator.
const schema = {
url: { type: "url" }
}
v.validate({ url: "http://google.com" }, schema);
v.validate({ url: "https://github.com/icebob" }, schema);
v.validate({ url: "www.facebook.com" }, schema);
Properties
Property | Default | Description |
---|
empty | false | If true , the validator accepts an empty string "" . |
uuid
This is an UUID validator.
const schema = {
uuid: { type: "uuid" }
}
v.validate({ uuid: "00000000-0000-0000-0000-000000000000" }, schema);
v.validate({ uuid: "10ba038e-48da-487b-96e8-8d3b99b6d18a" }, schema);
v.validate({ uuid: "9a7b330a-a736-51e5-af7f-feaf819cdc9f" }, schema);
v.validate({ uuid: "10ba038e-48da-487b-96e8-8d3b99b6d18a", version: 5 }, schema);
Properties
Property | Default | Description |
---|
version | 4 | UUID version in range 0-6. |
objectID
You can validate BSON/MongoDB ObjectID's
const { ObjectID } = require("mongodb")
const schema = {
id: {
type: "objectID",
ObjectID
}
}
const check = v.compile(schema);
check({ id: "5f082780b00cc7401fb8e8fc" })
check({ id: new ObjectID() })
check({ id: "5f082780b00cc7401fb8e8" })
Pro tip: By using defaults props for objectID rule, No longer needed to pass ObjectID
class in validation schema:
const { ObjectID } = require("mongodb")
const v = new Validator({
defaults: {
objectID: {
ObjectID
}
}
})
const schema = {
id: "objectID"
}
Properties
Property | Default | Description |
---|
convert | false | If true , the validator converts ObjectID HexString representation to ObjectID instance , if hexString the validator converts to HexString |
Custom validator
You can also create your custom validator.
const v = new Validator({
messages: {
evenNumber: "The '{field}' field must be an even number! Actual: {actual}"
}
});
v.add("even", function({ schema, messages }, path, context) {
return {
source: `
if (value % 2 != 0)
${this.makeError({ type: "evenNumber", actual: "value", messages })}
return value;
`
};
});
const schema = {
name: { type: "string", min: 3, max: 255 },
age: { type: "even" }
};
console.log(v.validate({ name: "John", age: 20 }, schema));
console.log(v.validate({ name: "John", age: 19 }, schema));
Or you can use the custom
type with an inline checker function:
const v = new Validator({
useNewCustomCheckerFunction: true,
messages: {
weightMin: "The weight must be greater than {expected}! Actual: {actual}"
}
});
const schema = {
name: { type: "string", min: 3, max: 255 },
weight: {
type: "custom",
minWeight: 10,
check(value, errors, schema) {
if (value < minWeight) errors.push({ type: "weightMin", expected: schema.minWeight, actual: value });
if (value > 100) value = 100
return value
}
}
};
console.log(v.validate({ name: "John", weight: 50 }, schema));
console.log(v.validate({ name: "John", weight: 8 }, schema));
const o = { name: "John", weight: 110 }
console.log(v.validate(o, schema));
Please note: the custom function must return the value
. It means you can also sanitize it.
Custom validation for built-in rules
You can define a custom
function in the schema for built-in rules. With it you can extend any built-in rules.
const v = new Validator({
useNewCustomCheckerFunction: true,
messages: {
phoneNumber: "The phone number must be started with '+'!"
}
});
const schema = {
name: { type: "string", min: 3, max: 255 },
phone: { type: "string", length: 15, custom: (v, errors) => {
if (!v.startsWith("+")) errors.push({ type: "phoneNumber" })
return v.replace(/[^\d+]/g, "");
}
}
};
console.log(v.validate({ name: "John", phone: "+36-70-123-4567" }, schema));
console.log(v.validate({ name: "John", phone: "36-70-123-4567" }, schema));
Please note: the custom function must return the value
. It means you can also sanitize it.
Asynchronous custom validations
You can also use async custom validators. This can be useful if you need to check something in a database or in a remote location.
In this case you should use async/await
keywords, or return a Promise
in the custom validator functions.
This implementation uses async/await
keywords. So this feature works only on environments which supports async/await:
- Chrome > 55
- Firefox > 52
- Edge > 15
- NodeJS > 8.x (or 7.6 with harmony)
- Deno (all versions)
To enable async mode, you should set $$async: true
in the root of your schema.
Example with custom checker function
const v = new Validator({
useNewCustomCheckerFunction: true,
messages: {
unique: "The username is already exist"
}
});
const schema = {
$$async: true,
name: { type: "string" },
username: {
type: "string",
min: 2,
custom: async (v, errors) => {
const res = await DB.checkUsername(v);
if (!res)
errors.push({ type: "unique", actual: value });
return v;
}
}
};
const check = v.compile(schema);
const res = await check(user);
console.log("Result:", res);
The compiled check
function contains an async
property, so you can check if it returns a Promise
or not.
const check = v.compile(schema);
console.log("Is async?", check.async);
Meta information for custom validators
You can pass any extra meta information for the custom validators which is available via context.meta
.
const schema = {
name: { type: "string", custom: (value, errors, schema, name, parent, context) => {
return context.meta.a;
} },
};
const check = v.compile(schema);
const res = check(obj, {
meta: { a: "from-meta" }
});
Custom error messages (l10n)
You can set your custom messages in the validator constructor.
const Validator = require("fastest-validator");
const v = new Validator({
messages: {
stringMin: "A(z) '{field}' mező túl rövid. Minimum: {expected}, Jelenleg: {actual}",
stringMax: "A(z) '{field}' mező túl hosszú. Minimum: {expected}, Jelenleg: {actual}"
}
});
v.validate({ name: "John" }, { name: { type: "string", min: 6 }});
Personalised Messages
Sometimes the standard messages are too generic. You can customize messages per validation type per field:
const Validator = require("fastest-validator");
const v = new Validator();
const schema = {
firstname: {
type: "string",
min: 6,
messages: {
string: "Please check your firstname",
stringMin: "Your firstname is too short"
}
},
lastname: {
type: "string",
min: 6,
messages: {
string: "Please check your lastname",
stringMin: "Your lastname is too short"
}
}
}
v.validate({ firstname: "John", lastname: 23 }, schema );
Plugins
You can apply plugins:
function myPlugin(validator){
}
const v = new Validator();
v.plugin(myPlugin)
Message types
Name | Default text |
---|
required | The '{field}' field is required. |
string | The '{field}' field must be a string. |
stringEmpty | The '{field}' field must not be empty. |
stringMin | The '{field}' field length must be greater than or equal to {expected} characters long. |
stringMax | The '{field}' field length must be less than or equal to {expected} characters long. |
stringLength | The '{field}' field length must be {expected} characters long. |
stringPattern | The '{field}' field fails to match the required pattern. |
stringContains | The '{field}' field must contain the '{expected}' text. |
stringEnum | The '{field}' field does not match any of the allowed values. |
stringNumeric | The '{field}' field must be a numeric string. |
stringAlpha | The '{field}' field must be an alphabetic string. |
stringAlphanum | The '{field}' field must be an alphanumeric string. |
stringAlphadash | The '{field}' field must be an alphadash string. |
stringHex | The '{field}' field must be a hex string. |
stringSingleLine | The '{field}' field must be a single line string. |
stringBase64 | The '{field}' field must be a base64 string. |
number | The '{field}' field must be a number. |
numberMin | The '{field}' field must be greater than or equal to {expected}. |
numberMax | The '{field}' field must be less than or equal to {expected}. |
numberEqual | The '{field}' field must be equal to {expected}. |
numberNotEqual | The '{field}' field can't be equal to {expected}. |
numberInteger | The '{field}' field must be an integer. |
numberPositive | The '{field}' field must be a positive number. |
numberNegative | The '{field}' field must be a negative number. |
array | The '{field}' field must be an array. |
arrayEmpty | The '{field}' field must not be an empty array. |
arrayMin | The '{field}' field must contain at least {expected} items. |
arrayMax | The '{field}' field must contain less than or equal to {expected} items. |
arrayLength | The '{field}' field must contain {expected} items. |
arrayContains | The '{field}' field must contain the '{expected}' item. |
arrayUnique | The '{actual}' value in '{field}' field does not unique the '{expected}' values. |
arrayEnum | The '{actual}' value in '{field}' field does not match any of the '{expected}' values. |
tuple | The '{field}' field must be an array. |
tupleEmpty | The '{field}' field must not be an empty array. |
tupleLength | The '{field}' field must contain {expected} items. |
boolean | The '{field}' field must be a boolean. |
function | The '{field}' field must be a function. |
date | The '{field}' field must be a Date. |
dateMin | The '{field}' field must be greater than or equal to {expected}. |
dateMax | The '{field}' field must be less than or equal to {expected}. |
forbidden | The '{field}' field is forbidden. |
email | The '{field}' field must be a valid e-mail. |
emailEmpty | The '{field}' field must not be empty. |
emailMin | The '{field}' field length must be greater than or equal to {expected} characters long. |
emailMax | The '{field}' field length must be less than or equal to {expected} characters long. |
url | The '{field}' field must be a valid URL. |
enumValue | The '{field}' field value '{expected}' does not match any of the allowed values. |
equalValue | The '{field}' field value must be equal to '{expected}'. |
equalField | The '{field}' field value must be equal to '{expected}' field value. |
object | The '{field}' must be an Object. |
objectStrict | The object '{field}' contains forbidden keys: '{actual}'. |
objectMinProps | "The object '{field}' must contain at least {expected} properties. |
objectMaxProps | "The object '{field}' must contain {expected} properties at most. |
uuid | The '{field}' field must be a valid UUID. |
uuidVersion | The '{field}' field must be a valid UUID version provided. |
mac | The '{field}' field must be a valid MAC address. |
luhn | The '{field}' field must be a valid checksum luhn. |
Message fields
Name | Description |
---|
field | The field name |
expected | The expected value |
actual | The actual value |
Development
npm run dev
Test
npm test
Coverage report
-----------------|----------|----------|----------|----------|-------------------|
File | % Stmts | % Branch | % Funcs | % Lines | Uncovered Line #s |
-----------------|----------|----------|----------|----------|-------------------|
All files | 100 | 97.73 | 100 | 100 | |
lib | 100 | 100 | 100 | 100 | |
messages.js | 100 | 100 | 100 | 100 | |
validator.js | 100 | 100 | 100 | 100 | |
lib/helpers | 100 | 100 | 100 | 100 | |
deep-extend.js | 100 | 100 | 100 | 100 | |
flatten.js | 100 | 100 | 100 | 100 | |
lib/rules | 100 | 96.43 | 100 | 100 | |
any.js | 100 | 100 | 100 | 100 | |
array.js | 100 | 100 | 100 | 100 | |
boolean.js | 100 | 100 | 100 | 100 | |
custom.js | 100 | 50 | 100 | 100 | 6 |
date.js | 100 | 100 | 100 | 100 | |
email.js | 100 | 100 | 100 | 100 | |
enum.js | 100 | 50 | 100 | 100 | 6 |
equal.js | 100 | 100 | 100 | 100 | |
forbidden.js | 100 | 100 | 100 | 100 | |
function.js | 100 | 100 | 100 | 100 | |
luhn.js | 100 | 100 | 100 | 100 | |
mac.js | 100 | 100 | 100 | 100 | |
multi.js | 100 | 100 | 100 | 100 | |
number.js | 100 | 100 | 100 | 100 | |
object.js | 100 | 100 | 100 | 100 | |
string.js | 100 | 95.83 | 100 | 100 | 55,63 |
tuple.js | 100 | 100 | 100 | 100 | |
url.js | 100 | 100 | 100 | 100 | |
uuid.js | 100 | 100 | 100 | 100 | |
-----------------|----------|----------|----------|----------|-------------------|
Contribution
Please send pull requests improving the usage and fixing bugs, improving documentation and providing better examples, or providing some tests, because these things are important.
License
fastest-validator is available under the MIT license.
Contact
Copyright (C) 2019 Icebob
