What is fastseries?
fastseries is a lightweight and fast utility for running a series of asynchronous functions in Node.js. It is designed to handle a series of tasks in a sequential manner, ensuring that each task completes before the next one starts.
What are fastseries's main functionalities?
Running a series of asynchronous functions
This feature allows you to run a series of asynchronous functions in sequence. Each function is executed one after the other, and the final callback is called once all functions have completed.
const series = require('fastseries')();
function task1(cb) {
setTimeout(() => {
console.log('Task 1 complete');
cb(null, 'result1');
}, 1000);
}
function task2(cb) {
setTimeout(() => {
console.log('Task 2 complete');
cb(null, 'result2');
}, 500);
}
function task3(cb) {
setTimeout(() => {
console.log('Task 3 complete');
cb(null, 'result3');
}, 200);
}
series(null, [task1, task2, task3], (err, results) => {
if (err) throw err;
console.log('All tasks complete', results);
});
Other packages similar to fastseries
async
The async package provides a comprehensive set of utilities for working with asynchronous JavaScript. It includes methods for running tasks in series, parallel, and other control flow patterns. Compared to fastseries, async is more feature-rich and versatile but may be heavier in terms of performance and size.
neo-async
neo-async is a drop-in replacement for async with a focus on performance. It offers similar functionalities to async but is optimized for speed. Like fastseries, it is designed to handle asynchronous control flow, but it provides a broader range of utilities and optimizations.
run-series
run-series is a minimalistic utility for running an array of asynchronous functions in series. It is similar to fastseries in terms of simplicity and performance but offers fewer features and customization options.
fastseries

Zero-overhead series function call for node.js.
Also supports each
and map
!
Benchmark for doing 3 calls setImmediate
1 million times:
- non-reusable
setImmediate
: 3887ms async.series
: 5981msasync.eachSeries
: 5087msasync.mapSeries
: 5540msneoAsync.series
: 4338msneoAsync.eachSeries
: 4195msneoAsync.mapSeries
: 4237mstiny-each-async
: 4575msfastseries
with results: 4096msfastseries
without results: 4063msfastseries
map: 4032msfastseries
each: 4168ms
These benchmarks where taken via bench.js
on node 4.2.2, on a MacBook
Pro Retina 2014.
If you need zero-overhead parallel function call, check out
fastparallel.
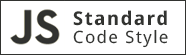
Example for series call
var series = require('fastseries')({
released: completed,
results: true
})
series(
{},
[something, something, something],
42,
done
)
function late (arg, cb) {
console.log('finishing', arg)
cb(null, 'myresult-' + arg)
}
function something (arg, cb) {
setTimeout(late, 1000, arg, cb)
}
function done (err, results) {
console.log('series completed, results:', results)
}
function completed () {
console.log('series completed!')
}
Example for each and map calls
var series = require('fastseries')({
released: completed,
results: true
})
series(
{},
something,
[1, 2, 3],
done
)
function late (arg, cb) {
console.log('finishing', arg)
cb(null, 'myresult-' + arg)
}
function something (arg, cb) {
setTimeout(late, 1000, arg, cb)
}
function done (err, results) {
console.log('series completed, results:', results)
}
function completed () {
console.log('series completed!')
}
Caveats
The done
function will be called only once, even if more than one error happen.
This library works by caching the latest used function, so that running a new series
does not cause any memory allocations.
License
ISC