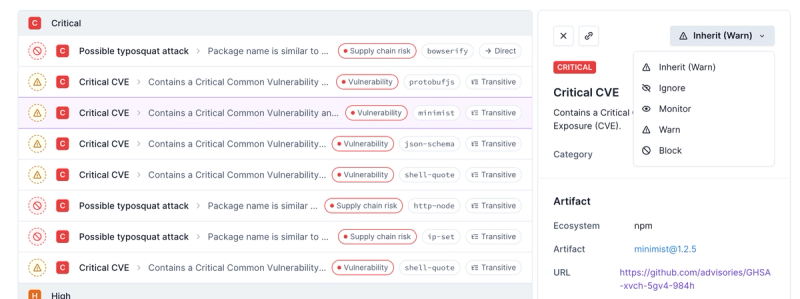
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
feathers-knex-modeler
Advanced tools
This package allows you to easily extend a table while you are developing it without requiring you to drop tables.
Readme
This package allows you to easily extend a table while you are developing it without requiring you to drop tables.
The below contain the contents of two files the model file and the service file.
// test.model.js - A KnexJS
//
// See http://knexjs.org/
// for more of what you can do here.
module.exports = function (app) {
const tableName = 'users'
const db = app.get('knexClient')
const Modeler = require('feathers-knex-modeler')
const modeler = new Modeler({
name: tableName,
depends: [],
columns: [
{ name: 'id', type: 'increments' },
{ name: 'name', type: 'text', options: [{ type: 'notNullable' }] },
{ name: 'schema_type', type: 'text', options: [{ type: 'notNullable' }] },
{ name: 'status', type: 'text', options: [{ type: 'notNullable' }] },
{ name: 'shared', type: 'bool', options: [{ type: 'notNullable' }] }
],
db
})
modeler.init()
return db
}
// test.service.js - A KnexJS
//
// Initializes the `fields` service on path `/test`
const createService = require('feathers-knex')
const createModel = require('../../models/test.model.js')
const hooks = require('./test.hooks')
module.exports = function (app) {
const Model = createModel(app)
const paginate = app.get('paginate')
const options = {
name: 'fields',
Model,
paginate
}
// Initialize our service with any options it requires
app.use('/fields', createService(options))
// Get our initialized service so that we can register hooks and filters
const service = app.service('fields')
service.hooks(hooks)
}
The below contain the contents of two files the model file and the service file.
'use strict'
const knex = require('knex')
const Modeler = require('feathers-knex-modeler')
const db = knex({
client: 'pg',
connection: {
host: '127.0.0.1',
database: 'myapp_test'
}
})
const testModel = new Modeler({
name: 'test',
depends: [],
columns: [
{ name: 'id', type: 'increments' },
{ name: 'name', type: 'text', options: [{ type: 'notNullable' }] },
{ name: 'schema_type', type: 'text', options: [{ type: 'notNullable' }] },
{ name: 'status', type: 'text', options: [{ type: 'notNullable' }] },
{ name: 'shared', type: 'bool', options: [{ type: 'notNullable' }] }
],
db
})
testModel.init()
FAQs
This package allows you to easily extend a table while you are developing it without requiring you to drop tables.
The npm package feathers-knex-modeler receives a total of 0 weekly downloads. As such, feathers-knex-modeler popularity was classified as not popular.
We found that feathers-knex-modeler demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.