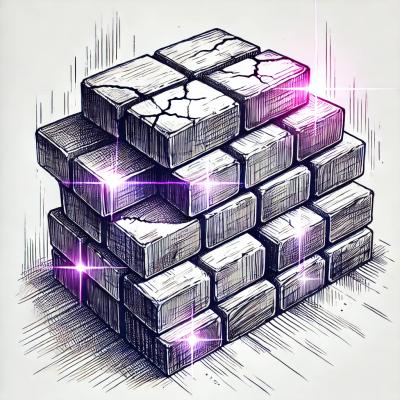
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Validate, sanitize and transform values with proper TypeScript types and with zero dependencies.
🔎 Validation: checks a value (example: check if value is string)
⚙ Sanitization: if a value is not valid, try to transform it (example: transform value to Date
)
🛠️ Transformation: transforms a value (example: parse JSON)
🔌 Everything is a function: functional approach makes it easy to extend – just plug in your own function anywhere!
npm install fefe
Validation only checks the provided value and returns it with proper types.
import { object, string } from 'fefe'
const validatePerson = object({ name: string() })
// result is of type { name: string }
const person = validatePerson({ name: 'Leia' })
// throws FefeError because 'foo' is not a valid property
validatePerson({ foo: 'bar' })
☝️ You can also use fefe
to define your types easily:
type Person = ReturnType<typeof validatePerson> // { name: string }
In this example a string
needs to be parsed as a Date
.
import { object, parseDate, string } from 'fefe'
const sanitizeMovie = object({
title: string(),
releasedAt: parseDate()
})
// { title: string, releasedAt: Date }
type Movie = ReturnType<typeof sanitizeMovie>
const movie: Movie = sanitizeMovie({
title: 'Star Wars',
releasedAt: '1977-05-25T12:00:00.000Z'
})
Then movie
equals { title: 'Star Wars', releasedAt: Date(1977-05-25T12:00:00.000Z) }
(releasedAt
now is a date).
Sometimes a value might already be of the right type. In the following example we use union()
to create a sanitizer that returns a provided value if it is a Date already and parse it otherwise. If it can't be parsed either the function will throw:
import { date, parseDate, union } from 'fefe'
const sanitizeDate = union(date(), parseDate())
This is a more complex example that can be applied to parsing environment variables or query string parameters. Note how easy it is to apply a chain of functions to validate and transform a value (here we use ramda
).
import { object, parseJson, string } from 'fefe'
import { pipe } from 'ramda'
const parseConfig = object({
gcloudCredentials: pipe(
parseJson(),
object({ secret: string() })
),
whitelist: pipe(string(), secret => str.split(','))
})
// { gcloudCredentials: { secret: string }, whitelist: string[] }
type Config = ReturnType<typeof parseConfig>
const config: Config = parseConfig({
gcloudCredentials: '{"secret":"foobar"}',
whitelist: 'alice,bob'
})
Then config
will equal { gcloudCredentials: { secret: 'foobar'}, whitelist: ['alice', 'bob'] }
.
FefeError
fefe
throws a FefeError
if a value can't be validated/transformed. A FefeError
has the following properties:
reason
: the reason for the error.value
: the value that was passed.path
: the path in value
to where the error occured.array(elementValidator, options?)
Returns a function (value: unknown) => T[]
that checks that the given value is an array and that runs elementValidator
on all elements. A new array with the results is returned.
Options:
elementValidator
: validator function (value: unknown) => T
that is applied to each element. The return values are returned as a new array.options.minLength?
, options.maxLength?
: restrict length of arrayboolean()
Returns a function (value: unknown) => boolean
that returns value
if it is a boolean and throws otherwise.
date(options?)
Returns a function (value: unknown) => Date
that returns value
if it is a Date and throws otherwise.
Options:
options.min?
, options.max?
: restrict dateenum(value1, value2, ...)
Returns a function (value: unknown) => value1 | value2 | ...
that returns value
if if equals one of the strings value1
, value2
, .... and throws otherwise.
number(options?)
Returns a function (value: unknown) => number
that returns value
if it is a number and throws otherwise.
Options:
options.min?
, options.max?
: restrict numberoptions.integer?
: require number to be an integer (default: false
)options.allowNaN?
, options.allowInfinity?
: allow NaN
or infinity
(default: false
)object(definition, options?)
Returns a function (value: unknown) => {...}
that returns value
if it is an object and all values pass the validation as specified in definition
, otherwise it throws. A new object is returned that has the results of the validator functions as values.
Options:
definition
: an object where each value is either:
(value: unknown) => T
orvalidate
: validator function (value: unknown) => T
optional?
: allow undefined values (default: false
)default?
: default value of type T
or function () => T
that returns a default valueallowExcessProperties?
: allow excess properties in value
(default: false
). Excess properties are not copied to the returned object.string(options?)
Returns a function (value: unknown) => string
that returns value
if it is a string and throws otherwise.
Options:
options.minLength?
, options.maxLength?
: restrict length of stringoptions.regex?
: require string to match regexunion(validator1, validator2, ...)
Returns a function (value: unknown) => return1 | return2 | ...
that returns the return value of the first validator called with value
that does not throw. The function throws if all validators throw.
parseBoolean()
Returns a function (value: string) => boolean
that parses a string as a boolean.
parseDate(options?)
Returns a function (value: string) => Date
that parses a string as a date.
Options:
options.iso?
: require value to be an ISO 8601 string.parseJson()
Returns a function (value: string) => any
that parses a JSON string.
parseNumber()
Returns a function (value: string) => number
that parses a number string.
FAQs
Validate, sanitize and transform values with proper types.
The npm package fefe receives a total of 44 weekly downloads. As such, fefe popularity was classified as not popular.
We found that fefe demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.