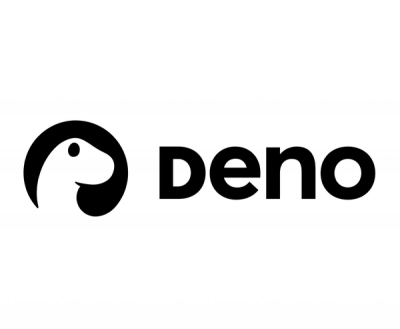
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
femonitor-web
Advanced tools
A SDK for web error and performance monitor using event subscription
Online example: http://monitor.huzerui.com
Open chrome developer tool to see console information
npm run watch // Watch tsfile change and compile by rollup
npm run server // Start a nodejs test server
Then visit localhost:3000
for example test
npm run build
npm run test
<script src="https://cdn.jsdelivr.net/npm/femonitor-web@latest/dist/index.min.js"></script>
npm i femonitor-web -S
import { Monitor } from "femonitor-web";
const monitor = Monitor.init();
/* Listen single event */
monitor.on([event], (emitData) => {});
/* Or listen some events by pass Array */
monitor.on(["jsError", "unhandleRejection"], (eventName, emitData) => {});
/* Or Listen all events */
monitor.on("event", (eventName, emitData) => {});
// Default full options
export const defaultTrackerOptions = {
env: "dev",
reportUrl: "",
data: {},
error: {
watch: true, // If listen all error
random: 1, // Sampling rate from 0 to 1, 1 means emit all error
repeat: 5, // Don't emit sample error events when exceed 5 times
delay: 1000 // Delay emit event after 1000 ms
},
performance: false, // If want to collect performance data
http: {
fetch: true, // If listen request use fetch interface
ajax: true // If listen ajax request
},
behavior: {
watch: false,
console: [ConsoleType.error],
click: true, // If set to true will listen all dom click event
queueLimit: 20 // Limit behavior queue to 20
},
/**
* rrweb use mutation observer api, for compatibility see:
* https://caniuse.com/mutationobserver
*/
rrweb: {
watch: false,
queueLimit: 50, // Limit rrweb queue to 20
delay: 1000 // Emit event after 1000 ms
},
isSpa: true // If watch is true, globalData can get _spaUrl for report when route change
};
const monitor = Monitor.init(defaultTrackerOptions);
Sdk support Vue.config.errorHandler
to handle error for get detail component info. You just need to call useVueErrorListener
before create Vue instance.
monitor.useVueErrorListener(Vue)
React supply a hook called componentDidCatch
for error listen and concept called ErrorBoundary which is enabled to catch errors at top and prevent app to shutdown. You can report it by yourself like below.
class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
componentDidCatch(error, info) {
this.setState({ hasError: true });
reportError(error, info);
}
render() {
if (this.state.hasError) {
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
EventName | Description |
---|---|
jsError | winodw.onerror |
vuejsError | Vue.config.errorHandler |
unhandleRejection | window.onunhandledrejection |
resourceError | Resource request error |
batchErrors | Batch collection of error events, trigger every specified time interval |
reqError | Network request error |
reqStart | Network request start |
reqEnd | Network request end |
performanceInfoReady | Performance data is ready |
event | Includes all events above |
FAQs
A web SDK for frontend error and performance monnitor
We found that femonitor-web demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.