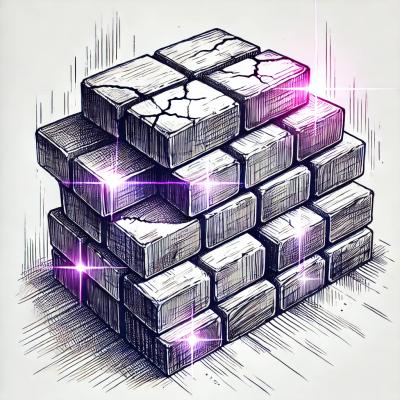
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
`ferrydb` is a lightweight, event-driven, and type-safe database ORM built on top of SQLite using TypeScript. It simplifies database operations by providing a schema-based model system with built-in validation and type inference.
ferrydb
is a lightweight, event-driven, and type-safe database ORM built on top of SQLite using TypeScript. It simplifies database operations by providing a schema-based model system with built-in validation and type inference.
To install ferrydb
, you need to have Node.js installed. You can then use npm to install the library:
npm install ferrydb better-sqlite3
First, define the schema for your data model. The schema specifies the structure and validation rules for your data.
import { Schema } from "ferrydb";
const userSchema = new Schema({
name: { type: String, required: true },
email: { type: String },
age: { type: Number },
});
Next, create a model using the defined schema. The model represents the database table and provides methods for interacting with the data.
import { model } from "ferrydb";
const User = model('User', userSchema);
You can now use the model to perform CRUD (Create, Read, Update, Delete) operations.
// Create a new user
const newUser = User.create({
name: "John",
email: "john@example.com",
age: 30,
});
// Find a user by name
const user = User.findOne({ name: "John" });
console.log(user);
// Update a user's age
if (user) {
user.age = 31;
user.save();
}
// Delete a user
if (user) {
user.delete();
}
new Schema(definition: SchemaDefinition)
Creates a new schema with the given definition.
definition
: An object defining the schema. Each key represents a field, and the value specifies the field's type and validation rules.model(name: string, schema: Schema)
Creates a new model with the specified name and schema.
name
: The name of the model, which corresponds to the table name in the database.schema
: The schema defining the structure and validation rules for the model.An instance of a model represents a single record in the database.
save()
Saves the current instance to the database. If the instance already exists, it updates the record.
delete()
Deletes the current instance from the database.
Here's a complete example demonstrating how to use ferrydb
:
import { Schema, model } from "ferrydb";
// Define a schema
const userSchema = new Schema({
name: { type: String, required: true },
email: { type: String },
age: { type: Number },
});
// Create a model
const User = model('User', userSchema);
// Create a new user
const newUser = User.create({
name: "John",
email: "john@example.com",
age: 30,
});
// Find a user by name
const user = User.findOne({ name: "John" });
console.log(user);
// Update a user's age
if (user) {
user.age = 31;
user.save();
}
// Delete a user
if (user) {
user.delete();
}
This project is licensed under the MIT License.
FAQs
FerryDB is a simple, flexible, and powerful SQLite-based ORM for Node.js. It allows you to define schemas, validate data, and perform CRUD operations with ease.
The npm package ferrydb receives a total of 9 weekly downloads. As such, ferrydb popularity was classified as not popular.
We found that ferrydb demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.