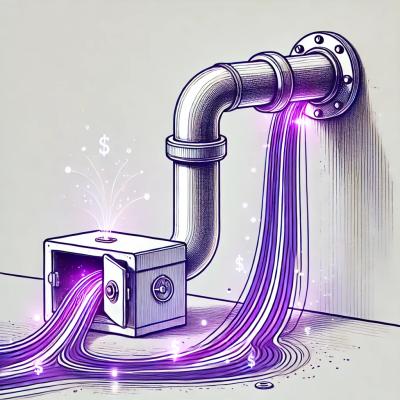
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
A powerful and flexible HTTP client for TypeScript/JavaScript applications, built on top of the Fetch API with additional features like request/response interceptors, automatic retries, timeout handling, and type safety.
npm install fetcherize
import { HttpClient } from "fetcherize";
// Create a new instance
const client = new HttpClient("https://api.example.com", {
timeout: 5000,
retries: 3,
retryDelay: 1000,
});
// Make requests
try {
const response = await client.get<User>("/users/1");
console.log(response.data); // Type-safe user data
} catch (error) {
console.error("Request failed:", error);
}
const client = new HttpClient(baseURL?: string, config?: HttpClientConfig);
Configuration options:
interface HttpClientConfig {
retries?: number; // Number of retry attempts (default: 1)
retryDelay?: number; // Delay between retries in ms (default: 1000)
timeout?: number; // Request timeout in ms (default: 10000)
headers?: Record<string, string>; // Default headers
}
The client provides method shortcuts for common HTTP methods:
// GET request
const response = await client.get<T>(url, config?);
// POST request
const response = await client.post<T>(url, data?, config?);
// PUT request
const response = await client.put<T>(url, data?, config?);
// PATCH request
const response = await client.patch<T>(url, data?, config?);
// DELETE request
const response = await client.delete<T>(url, config?);
interface RequestConfig extends RequestInit {
headers?: Record<string, string>;
timeout?: number;
signal?: AbortSignal;
retries?: number;
retryDelay?: number;
}
interface HttpResponse<T = unknown> {
data: T; // Response data
status: number; // HTTP status code
headers: Headers; // Response headers
config: RequestConfig; // Request configuration
}
Add request interceptors:
const removeInterceptor = client.addRequestInterceptor(async (config) => {
// Modify config before request
config.headers = {
...config.headers,
Authorization: "Bearer token",
};
return config;
});
Add response interceptors:
const removeInterceptor = client.addResponseInterceptor(async (response) => {
// Process response before returning
console.log("Response received:", response.status);
return response;
});
The client throws HttpError
for failed requests:
try {
const response = await client.get("/api/data");
} catch (error) {
if (error instanceof HttpError) {
console.error("Status:", error.response?.status);
console.error("Message:", error.message);
console.error("Config:", error.config);
}
}
The client automatically handles FormData:
const formData = new FormData();
formData.append("file", fileInput.files[0]);
formData.append("name", "example");
const response = await client.post("/upload", formData);
const client = new HttpClient("https://api.example.com", {
timeout: 5000,
retries: 3,
retryDelay: 1000,
headers: {
Accept: "application/json",
"X-Custom-Header": "value",
},
});
interface User {
id: number;
name: string;
email: string;
}
const response = await client.get<User>("/user/1");
// response.data is typed as User
console.log(response.data.name);
client.addRequestInterceptor(async (config) => {
const token = await getAuthToken();
config.headers = {
...config.headers,
Authorization: `Bearer ${token}`,
};
return config;
});
client.addResponseInterceptor(async (response) => {
if (response.status === 401) {
// Handle token refresh
await refreshToken();
// Retry the request
return client.request(response.config);
}
return response;
});
FAQs
A simple library used for making HTTP requests.
The npm package fetcherize receives a total of 2 weekly downloads. As such, fetcherize popularity was classified as not popular.
We found that fetcherize demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.