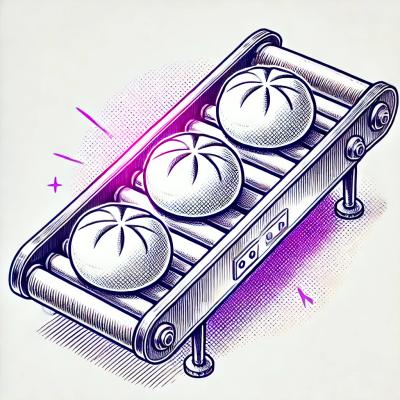
Security News
Bun 1.2 Released with 90% Node.js Compatibility and Built-in S3 Object Support
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.
ffmpeg-kit-react-native
Advanced tools
Includes both FFmpeg
and FFprobe
Supports
Both Android
and iOS
FFmpeg v6.0
arm-v7a
, arm-v7a-neon
, arm64-v8a
, x86
and x86_64
architectures on Android
Android API Level 24
or later
API Level 16
on LTS releasesarmv7
, armv7s
, arm64
, arm64-simulator
, i386
, x86_64
, x86_64-mac-catalyst
and arm64-mac-catalyst
architectures on iOS
iOS SDK 12.1
or later
iOS SDK 10
on LTS releasesCan process Storage Access Framework (SAF) Uris on Android
25 external libraries
dav1d
, fontconfig
, freetype
, fribidi
, gmp
, gnutls
, kvazaar
, lame
, libass
, libiconv
, libilbc
, libtheora
, libvorbis
, libvpx
, libwebp
, libxml2
, opencore-amr
, opus
, shine
, snappy
, soxr
, speex
, twolame
, vo-amrwbenc
, zimg
4 external libraries with GPL license
vid.stab
, x264
, x265
, xvidcore
zlib
and MediaCodec
Android system libraries
bzip2
, iconv
, libuuid
, zlib
system libraries and AudioToolbox
, VideoToolbox
, AVFoundation
system frameworks on iOS
Includes Typescript definitions
Licensed under LGPL 3.0
by default, some packages licensed by GPL v3.0
effectively
yarn add ffmpeg-kit-react-native
FFmpeg
includes built-in encoders for some popular formats. However, there are certain external libraries that needs
to be enabled in order to encode specific formats/codecs. For example, to encode an mp3
file you need lame
or
shine
library enabled. You have to install a ffmpeg-kit-react-native
package that has at least one of them inside.
To encode an h264
video, you need to install a package with x264
inside. To encode vp8
or vp9
videos, you need
a ffmpeg-kit-react-native
package with libvpx
inside.
ffmpeg-kit
provides eight packages that include different sets of external libraries. These packages are named
according to the external libraries included. Refer to the
Packages wiki page to see the names of those
packages and external libraries included in each one of them.
The following table shows all package names and their respective API levels, iOS deployment targets defined in
ffmpeg-kit-react-native
.
Package | Main Release | LTS Release | ||||
---|---|---|---|---|---|---|
Name | Android API Level | iOS Minimum Deployment Target | Name | Android API Level | iOS Minimum Deployment Target | |
min | min | 24 | 12.1 | min-lts | 16 | 10 |
min-gpl | min-gpl | 24 | 12.1 | min-gpl-lts | 16 | 10 |
https | (*) https | 24 | 12.1 | https-lts | 16 | 10 |
https-gpl | https-gpl | 24 | 12.1 | https-gpl-lts | 16 | 10 |
audio | audio | 24 | 12.1 | audio-lts | 16 | 10 |
video | video | 24 | 12.1 | video-lts | 16 | 10 |
full | full | 24 | 12.1 | full-lts | 16 | 10 |
full-gpl | full-gpl | 24 | 12.1 | full-gpl-lts | 16 | 10 |
(*) - Main https
package is the default package
Installing ffmpeg-kit-react-native
enables the https
package by default. It is possible to enable other
packages using the instructions below.
Edit android/build.gradle
file and add the package name in ext.ffmpegKitPackage
variable.
ext {
ffmpegKitPackage = "<package name>"
}
Edit ios/Podfile
file and add the package name as subspec
. After that run pod install
again.
pod 'ffmpeg-kit-react-native', :subspecs => ['<package name>'], :podspec => '../node_modules/ffmpeg-kit-react-native/ffmpeg-kit-react-native.podspec'
Note that if you have use_native_modules!
in your Podfile
, specifying a subspec
may cause the following error.
You can fix it by defining ffmpeg-kit-react-native
dependency before use_native_modules!
in your Podfile
.
[!] There are multiple dependencies with different sources for `ffmpeg-kit-react-native` in `Podfile`:
- ffmpeg-kit-react-native (from `../node_modules/ffmpeg-kit-react-native`)
- ffmpeg-kit-react-native/video (from `../node_modules/ffmpeg-kit-react-native/ffmpeg-kit-react-native.podspec`)
In order to install the LTS
variant, install the https-lts
package using instructions in 2.2
or append -lts
to
the package name you are using.
ffmpeg-kit-react-native
is published in two variants: Main Release
and LTS Release
. Both releases share the
same source code but is built with different settings (Architectures, API Level, iOS Min SDK, etc.). Refer to the
LTS Releases wiki page to see how they differ from each
other.
Execute FFmpeg commands.
import { FFmpegKit } from 'ffmpeg-kit-react-native';
FFmpegKit.execute('-i file1.mp4 -c:v mpeg4 file2.mp4').then(async (session) => {
const returnCode = await session.getReturnCode();
if (ReturnCode.isSuccess(returnCode)) {
// SUCCESS
} else if (ReturnCode.isCancel(returnCode)) {
// CANCEL
} else {
// ERROR
}
});
Each execute
call creates a new session. Access every detail about your execution from the
session created.
FFmpegKit.execute('-i file1.mp4 -c:v mpeg4 file2.mp4').then(async (session) => {
// Unique session id created for this execution
const sessionId = session.getSessionId();
// Command arguments as a single string
const command = session.getCommand();
// Command arguments
const commandArguments = session.getArguments();
// State of the execution. Shows whether it is still running or completed
const state = await session.getState();
// Return code for completed sessions. Will be undefined if session is still running or FFmpegKit fails to run it
const returnCode = await session.getReturnCode()
const startTime = session.getStartTime();
const endTime = await session.getEndTime();
const duration = await session.getDuration();
// Console output generated for this execution
const output = await session.getOutput();
// The stack trace if FFmpegKit fails to run a command
const failStackTrace = await session.getFailStackTrace()
// The list of logs generated for this execution
const logs = await session.getLogs();
// The list of statistics generated for this execution (only available on FFmpegSession)
const statistics = await session.getStatistics();
});
Execute FFmpeg
commands by providing session specific execute
/log
/session
callbacks.
FFmpegKit.executeAsync('-i file1.mp4 -c:v mpeg4 file2.mp4', session => {
// CALLED WHEN SESSION IS EXECUTED
}, log => {
// CALLED WHEN SESSION PRINTS LOGS
}, statistics => {
// CALLED WHEN SESSION GENERATES STATISTICS
});
Execute FFprobe
commands.
FFprobeKit.execute(ffprobeCommand).then(async (session) => {
// CALLED WHEN SESSION IS EXECUTED
});
Get media information for a file/url.
FFprobeKit.getMediaInformation(testUrl).then(async (session) => {
const information = await session.getMediaInformation();
if (information === undefined) {
// CHECK THE FOLLOWING ATTRIBUTES ON ERROR
const state = FFmpegKitConfig.sessionStateToString(await session.getState());
const returnCode = await session.getReturnCode();
const failStackTrace = await session.getFailStackTrace();
const duration = await session.getDuration();
const output = await session.getOutput();
}
});
Stop ongoing FFmpeg operations.
FFmpegKit.cancel();
FFmpegKit.cancel(sessionId);
FFmpegKit
and FFprobeKit
.Reading a file:
FFmpegKitConfig.selectDocumentForRead('*/*').then(uri => {
FFmpegKitConfig.getSafParameterForRead(uri).then(safUrl => {
FFmpegKit.executeAsync(`-i ${safUrl} -c:v mpeg4 file2.mp4`);
});
});
Writing to a file:
FFmpegKitConfig.selectDocumentForWrite('video.mp4', 'video/*').then(uri => {
FFmpegKitConfig.getSafParameterForWrite(uri).then(safUrl => {
FFmpegKit.executeAsync(`-i file1.mp4 -c:v mpeg4 ${safUrl}`);
});
});
Get previous FFmpeg
, FFprobe
and MediaInformation
sessions from the session history.
FFmpegKit.listSessions().then(sessionList => {
sessionList.forEach(async session => {
const sessionId = session.getSessionId();
});
});
FFprobeKit.listFFprobeSessions().then(sessionList => {
sessionList.forEach(async session => {
const sessionId = session.getSessionId();
});
});
FFprobeKit.listMediaInformationSessions().then(sessionList => {
sessionList.forEach(async session => {
const sessionId = session.getSessionId();
});
});
Enable global callbacks.
Session type specific Complete Callbacks, called when an async session has been completed
FFmpegKitConfig.enableFFmpegSessionCompleteCallback(session => {
const sessionId = session.getSessionId();
});
FFmpegKitConfig.enableFFprobeSessionCompleteCallback(session => {
const sessionId = session.getSessionId();
});
FFmpegKitConfig.enableMediaInformationSessionCompleteCallback(session => {
const sessionId = session.getSessionId();
});
Log Callback, called when a session generates logs
FFmpegKitConfig.enableLogCallback(log => {
const message = log.getMessage();
});
Statistics Callback, called when a session generates statistics
FFmpegKitConfig.enableStatisticsCallback(statistics => {
const size = statistics.getSize();
});
Register system fonts and custom font directories.
FFmpegKitConfig.setFontDirectoryList(["/system/fonts", "/System/Library/Fonts", "<folder with fonts>"]);
You can see how FFmpegKit
is used inside an application by running react-native
test applications developed under
the FFmpegKit Test project.
See Tips wiki page.
See License wiki page.
See Patents wiki page.
FAQs
FFmpeg Kit for React Native
The npm package ffmpeg-kit-react-native receives a total of 7,776 weekly downloads. As such, ffmpeg-kit-react-native popularity was classified as popular.
We found that ffmpeg-kit-react-native demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.
Security News
Biden's executive order pushes for AI-driven cybersecurity, software supply chain transparency, and stronger protections for federal and open source systems.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.