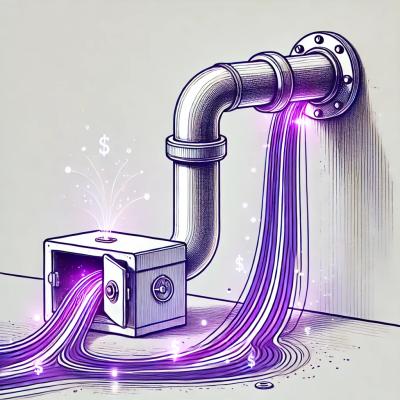
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Route authorization module for Node.js Express applications.
npm install --save fi-auth
var auth = require('fi-auth');
You must call it with your Express' app instance, to attach the routes, and a configuration object. It's important to initialize the Express' session before you configure Fi Auth:
var session = require('express-session');
var express = require('express');
var auth = require('fi-auth');
var app = express();
app.use(session());
auth(app, config);
/* And now your routes... */
app.get('/', function (req, res, next) {
//...
});
The configuration Object
must have an authorizer function and a route array. The debug
parameter is optional but recommended.
IMPORTANT: All routes are allowed by default!
debug: This option can be a Function
to log with or a Boolean
. If true
it'll use console.log
.
authorizer: This is required and must be a Function
. This Function
runs on each request and should return the String
or Number
that will be compared against the allows
parameter value inside each route definition. The authorizer Function
return value will be attached to req.session.authorized
.
routes: An Array
with the routes to authorize:
String
or an Array
of HTTP request method(s) to filter. If no method is specified it defaults to all.String
or an Array
of strings with the route(s) path(s) to filter.String
or an Array
of authorization value(s) to compare with the authorizer method returned value.{
debug: require('debug')('app:auth'),
authorizer: function (req) {
/* IMPORTANT: This is just a simple example */
/* Check if there's a user in session */
if (req.session.user) {
/* Check whether the user has 'admin' role */
return req.session.user.admin && 'admin' || 'user';
}
/* There's no user in session */
return null;
},
/* Routes authorization definition */
routes: [{
/* All request methods are filtered */
path: '/api/users/count', /* On this route path only */
allows: 'admin' /* And allows 'admin' only */
}, {
method: 'GET', /* Only GET requests are filtered */
path: '/api/users', /* On this route path only */
allows: 'admin' /* And allows 'admin' only */
}, {
method: ['POST', 'PUT', 'DELETE'], /* Only POST, PUT and DELETE requests are filtered */
path: ['/api/users', '/api/stuff'], /* On this route paths only */
allows: 'admin' /* And allows 'admin' only */
}, {
method: ['POST', 'DELETE'], /* Only POST, PUT and DELETE requests are filtered */
path: '/api/content', /* On this route path only */
allows: ['user', 'admin'] /* And allows both 'user' and 'admin' */
}]
}
FAQs
Route authorization module for Node.js Express apps
The npm package fi-auth receives a total of 0 weekly downloads. As such, fi-auth popularity was classified as not popular.
We found that fi-auth demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.