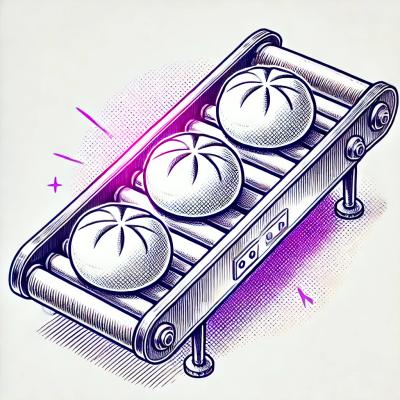
Security News
Bun 1.2 Released with 90% Node.js Compatibility and Built-in S3 Object Support
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.
A high-performance, thread-safe LRU cache implementation for fibjs with TTL support and resolver functionality
A high-performance, thread-safe LRU (Least Recently Used) cache implementation for fibjs with TTL support and resolver functionality.
fibjs --install fib-cache
const { LRU } = require('fib-cache');
// Create a cache with max 100 items and 5s TTL
const cache = new LRU({
max: 100,
ttl: 5000
});
// Basic operations
cache.set('key1', 'value1');
console.log(cache.get('key1')); // 'value1'
// The item will be automatically removed after 5 seconds
coroutine.sleep(5000);
console.log(cache.get('key1')); // undefined
const cache = new LRU({
max: 1000,
ttl: 3600000, // 1 hour
resolver: (key) => {
// Automatically fetch value if not in cache
const value = fetchDataFromDatabase(key);
return value;
}
});
// Will automatically fetch from database if not in cache
const value = cache.get('user:123');
// Example with sleep
const slowCache = new LRU({
max: 100,
resolver: (key) => {
// Simulate slow operation
coroutine.sleep(100);
return `computed_${key}`;
}
});
// This will wait for resolver
console.log(slowCache.get('test')); // 'computed_test'
const cache = new LRU({ max: 2 });
cache.set('a', 1);
cache.set('b', 2);
cache.set('c', 3); // 'a' will be evicted
console.log(cache.get('a')); // undefined
console.log(cache.get('b')); // 2
console.log(cache.get('c')); // 3
max
(number, optional): Maximum number of items in cache. Default: 0 (no limit)ttl
(number, optional): Time-to-live in milliseconds. Default: 0 (no expiration)resolver
(Function, optional): Function to resolve cache missesget(key, [resolver])
: Get value by key
key
: The key to look upresolver
(optional): A one-time resolver function for this specific get operationset(key, value)
: Set value for keydelete(key)
: Remove item by keyhas(key)
: Check if key existsclear()
: Remove all itemskeys()
: Get all keysvalues()
: Get all valuesentries()
: Get all key-value pairssize
: Get current cache sizeThe cache is designed to be thread-safe in fibjs environment:
const cache = new LRU({ max: 100 });
// Safe for concurrent access
coroutine.parallel([
() => cache.set('key1', 'value1'),
() => cache.get('key1'),
() => cache.delete('key1')
]);
const cache = new LRU({
max: 1000,
ttl: 3600000, // 1 hour
// Default resolver
resolver: (key) => {
return fetchFromMainDB(key);
}
});
// Using default resolver
const value1 = cache.get('user:123');
// Using one-time custom resolver
const value2 = cache.get('user:456', (key) => {
return fetchFromBackupDB(key);
});
// Example with different resolvers
const slowCache = new LRU({
max: 100,
// Default slow resolver
resolver: (key) => {
coroutine.sleep(100);
return `slow_${key}`;
}
});
// Using default slow resolver
console.log(slowCache.get('test')); // 'slow_test'
// Using fast one-time resolver
console.log(slowCache.get('test', key => `fast_${key}`)); // 'fast_test'
MIT License
Contributions are welcome! Please feel free to submit a Pull Request.
FAQs
A high-performance, thread-safe LRU cache implementation for fibjs with TTL support and resolver functionality
We found that fib-cache demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.
Security News
Biden's executive order pushes for AI-driven cybersecurity, software supply chain transparency, and stronger protections for federal and open source systems.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.