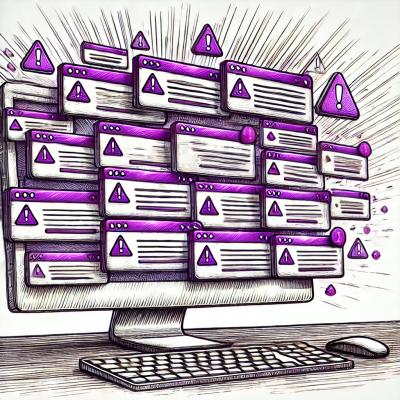
Security News
Combatting Alert Fatigue by Prioritizing Malicious Intent
In 2023, data breaches surged 78% from zero-day and supply chain attacks, but developers are still buried under alerts that are unable to prevent these threats.
filestack-js
Advanced tools
Official browser client to the Filestack API's. Available via NPM and Filestack CDN.
Filestack documentation can be found here: https://filestack.com/docs/
This library is shipped as both a UMD module and a ES2015 module for modern Javascript bundlers. There is also a separate minified UMD module available on the Filestack CDN.
npm install --save filestack-js
Via ES6 modules:
import filestack from 'filestack-js';
const apikey = 'abc';
const client = filestack.init(apikey);
Via script tag:
(Note http://static.filestackapi.com/v3/filestack.js will always point to the latest version. This is not recommended for production.)
<script src="http://static.filestackapi.com/v3/filestack-0.6.2.js"></script>
<script>
const apikey = 'abc';
const client = filestack.init(apikey);
</script>
object
object
object
Promise
Promise
Promise
Promise
Promise
string
Promise
string
object
Initializes the client.
Returns: object
- Object containing client methods.
Params
string
- Filestack API key. Get a free key here.object
- Read about security policies.
string
- Filestack security policy encoded in base64.string
- HMAC-SHA256 sIgnature for the security policy.string
Gets current version.
Example
import filestack from 'filestack-js';
console.log(filestack.version);
object
Get current security parameters.
Returns: object
- Object containing current security parameters
object
Set security parameters -- useful for changing policy on instantiated client.
Note: Does not currently work with pick
. You will need to init a new client if you want to propagate new security parameters to the picker.
Returns: object
- Object containing current session parameters
Params
object
- Read about security policies.
string
- Filestack security policy encoded in base64.string
- HMAC-SHA256 sIgnature for the security policy.Example
client.setSecurity({ policy: 'policy', signature: 'signature' });
Promise
Opens the picker UI.
Resolve: object
- Object contains keys filesUploaded
and filesFailed
which are both arrays of file metadata.
Params
object
Array.<string>
- Valid sources are:
local_file_system
- Defaultimagesearch
- Defaultfacebook
- Defaultinstagram
- Defaultgoogledrive
- Defaultdropbox
- Defaultwebcam
- Desktop only. Please use local_file_system
for mobile. Not currently supported in Safari or IE.evernote
flickr
box
github
gmail
picasa
onedrive
clouddrive
string
| Array.<string>
- Restrict file types that are allowed to be picked. Formats accepted:
.pdf
<- any file extensionimage/jpeg
<- any mime type commonly known by browsersimage/*
<- accept all types of imagesvideo/*
<- accept all types of video filesaudio/*
<- accept all types of audio filesapplication/*
<- accept all types of application filestext/*
<- accept all types of text filesboolean
= false
- For cloud sources whether to link or store files.string
= "en"
- Sets locale. Accepts: da
, de
, en
, es
, fr
, it
, ja
, nl
, pl
, pt
, ru
, zh
.number
= 1
- Minimum number of files required to start uploading.number
= 1
- Maximum number of files allowed to upload.number
- Restrict selected files to a maximum number of bytes. (e.g. 10 * 1024 * 1024
for 1MB limit).boolean
= false
- Whether to start uploading automatically when maxFiles is hit.boolean
= false
- Hide the picker UI once uploading begins.boolean
= true
- Start uploading immediately on file selection.boolean
= false
- When true removes ability to edit images.object
- Specify transforms for images passed to the transformations UI.
boolean
| object
= true
- Enable crop.
number
- Maintain aspect ratio for crop selection. (e.g. 16/9 or 4/3)boolean
= true
- Enable circle crop. Disabled if aspectRatio
is defined and not 1.array
- Minimum dimensions for picked image. Image will be upscaled if smaller. (e.g. [200, 300])array
- Maximum dimensions for picked image. Image will be downscaled if smaller. (e.g. [200, 300])Array.<string>
- Enable image filters. Pick from: sepia
, monochrome
. All enabled by default.object
- Options for file storage.
string
= "s3"
- One of s3
, gcs
, rackspace
, azure
, dropbox
.string
- Valid S3 region for the selected S3 bucket. S3 only.string
string
string
- One of public
or private
.onFileSelected
- Called whenever user selects a file.onFileUploadStarted
- Called when a file begins uploading.onFileUploadProgress
- Called during multi-part upload progress events. Local files only.onFileUploadFinished
- Called when a file is done uploading.onFileUploadFailed
- Called when uploading a file fails.function
- Called when the UI is exited.Example
client.pick({
maxFiles: 20,
fromSources: ['local_file_system', 'facebook'],
}).then(res => console.log(res));
function
Params
object
- File metadata.Example
// Using to veto file selection
// If you throw any error in this function it will reject the file selection.
// The error message will be displayed to the user as an alert.
onFileSelected(file) {
if (file.size > 1000 * 1000) {
throw new Error('File too big, select something smaller than 1MB');
}
}
// Using to change selected file name
onFileSelected(file) {
file.name = 'foo';
// It's important to return altered file by the end of this function.
return file;
}
function
Params
object
- File metadata.function
Params
object
- File metadata.function
Params
object
- File metadata.error
- Error instance for this upload.function
Params
object
- File metadata.object
- Progress event.
number
- Percent of file uploaded.number
- Total number of bytes uploaded for this file.Promise
Interface to the Filestack Store API. Used for storing from a URL.
Resolve: object
- Metadata of stored file.
Reject: error
- A Superagent error object.
Params
string
- Valid URL to a file.object
string
string
= "s3"
- One of s3
, gcs
, rackspace
, azure
, dropbox
.string
string
string
string
- Valid S3 region for the selected container (S3 only).string
- One of public
or private
.Example
client
.storeURL('https://d1wtqaffaaj63z.cloudfront.net/images/NY_199_E_of_Hammertown_2014.jpg')
.then(res => console.log(res));
Promise
Interface to the Filestack Retrieve API. Used for accessing files via Filestack handles.
Resolve: object
- Metadata of stored file or stored file, depending on metadata / head option.
Reject: error
- A Superagent error object.
Params
string
- Valid Filestack handle.object
boolean
- return json of file metadataboolean
- perform a 'head' request instead of a 'get'boolean
- X-File-Name will be returnedstring
- add extension to handleExample
client
.retrieve('DCL5K46FS3OIxb5iuKby')
.then((blob) => {
var urlCreator = window.URL || window.webkitURL;
var imageUrl = urlCreator.createObjectURL(blob);
document.querySelector('#myImage').src = imageUrl;
})
.catch((err) => {
console.log(err);
}));
Promise
Interface to the Filestack Remove API. Used for removing files, requires security to be enabled.
Resolve: object
- Result of remove.
Reject: error
- A Superagent error object.
Params
string
- Valid Filestack handle.Example
client
.remove('DCL5K46FS3OIxb5iuKby')
.then((res) => {
console.log(res);
})
.catch((err) => {
console.log(err);
}));
Promise
Interface to the Filestack Metadata API. Used for retrieving detailed data of stored files.
Resolve: object
- Result of metadata.
Reject: error
- A Superagent error object.
Params
string
- Valid Filestack handle.object
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
boolean
Example
client
.metadata('DCL5K46FS3OIxb5iuKby')
.then((res) => {
console.log(res);
})
.catch((err) => {
console.log(err);
}));
string
Interface to the Filestack transformation engine.
Returns: string
- A new URL that points to the transformed resource.
Params
string
- Valid URL to an image.transformOptions
- Transformations are applied in the order specified by this object.Example
const transformedUrl = client.transform(url, {
crop: {
dim: {
x: 0,
y: 50,
width: 300,
height: 300,
},
},
vignette: {
blurmode: 'gaussian',
amount: 50,
},
flip: true,
};
// optionally store the new URL
client.storeURL(transformedUrl).then(res => console.log(res));
object
Params
object
- Crop options.
object
- Crop dimensions.
number
number
number
number
object
- Resize options. At least one option is required.
number
number
string
- One of clip
, crop
, scale
, max
.string
- One of center
, top
, bottom
, left
, right
, faces
, or align pair like ['top', 'left']
.object
- Rotate options. At least one option is required.
number
| string
- Can be number in range 0-359 or exif
.boolean
string
boolean
- Flip imageboolean
- Flop imageobject
- Rounded corners options.
number
| string
- Can be number in range 1-10000 or max
.number
string
object
- Vignette options.
number
string
- One of linear
or gaussian
.string
object
- Polaroid options.
string
number
string
object
- Torn edges options.
Array.<number>
- Range format [10, 50]
.string
object
- Shadow options.
number
number
Array.<number>
- Range format [25, 25]
.string
string
object
- Circle options.
string
object
- Border options.
number
number
number
boolean
- Monochrome.object
- Sepia.
number
Promise
Initiates a direct-to-S3 multi-part upload. Uses Filestack S3 by default. Read how to configure your own S3 buckets here.
Resolve: object
- Metadata of uploaded file.
Reject: error
- An Error object depending on where the flow halted.
Params
Blob
- must be a valid File or Blob.object
number
= 6 * 1024 * 1024
- Size of each uploaded part.number
= 5
- Max number of concurrent parts uploading.number
= 10
- Number of times to retry a failed part of the flow.number
= 1000
- Frequency (in milliseconds) at which progress events are dispatched.progressCallback
- Called regularly to give progress updates.retryCallback
- Called when a retry is initiated.object
- Configure where the file is stored.
string
- Define a custom filename for the Blob/File being uploaded.string
= "s3"
- Valid options are: s3
, gcs
, dropbox
, azure
, rackspace
.string
- Valid S3 region for the selected container (S3 only).string
- Name of the storage container.string
- Path where the file will be stored. A trailing slash will put the file in that folder path.string
- Valid options are private
or public
.object
- A control token that can be used to call cancel(), pause(), and resume().Example
const token = {};
const onRetry = (obj) => {
console.log(`Retrying ${obj.location} for ${obj.filename}. Attempt ${obj.attempt} of 10.`);
};
client.upload(file, { onRetry }, { filename: 'foobar.jpg' }, token)
.then(res => console.log(res));
token.pause(); // Pause flow
token.resume(); // Resume flow
token.cancel(); // Cancel flow (rejects)
function
Params
object
- Progress event.
number
- Percent of total file upload progress.number
- Total number of bytes uploaded thus far across all parts.function
Params
object
- Retry information object.
string
- Which part of the flow is being retried.Array.<object>
- Array of current parts at this point in the flow.string
- Name of the file being retried.number
- Current attempt.© 2017 Filestack.
0.6.2 (2017-05-26)
Picker changes
uploadInBackground: false
was not respecting storeTo
optionsFAQs
Official JavaScript library for Filestack
The npm package filestack-js receives a total of 76,917 weekly downloads. As such, filestack-js popularity was classified as popular.
We found that filestack-js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
In 2023, data breaches surged 78% from zero-day and supply chain attacks, but developers are still buried under alerts that are unable to prevent these threats.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.