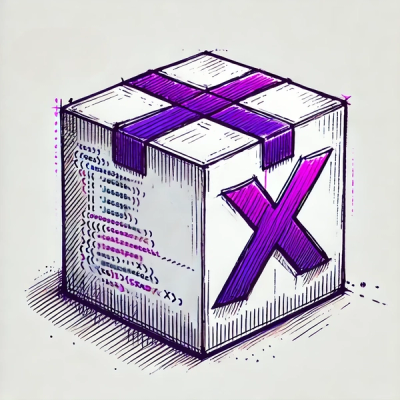
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
This project is under heavy development, and the API has not solidfied yet. Don't use this for anything important...yet.
Bind data to DOM with zero configuration. Just call .fill(data)
.
<div id="template">
<h2 class="project"></h2>
<div data-bind="details">
<a name="githubLink"></a>
<ul class="features">
<li></li>
</ul>
</div>
</div>
$('#template').fill({
project: 'Fill',
details: {
githubLink: { _text: 'Fill', _href: 'https://github.com/profit-strategies/fill' },
features: {
_style: 'color:green'
li: [
'Uses plain HTML with no extra templating markup',
'Runs on the client or server (with jsdom)',
'Render arrays without loops or partials',
'Nested objects and collections',
'Compatible (tested on IE6+, Chrome and Firefox)',
]
}
});
<div id="template">
<h2 class="project">Fill</h2>
<div data-bind="details">
<a name="githubLink" href="https://github.com/profit-strategies/fill">Fill</a>
<ul class="features" style="color:green">
<li>Uses plain HTML with no extra templating markup</li>
<li>Runs on the client or server (with jsdom)</li>
<li>Render arrays without loops or partials</li>
<li>Nested objects and collections</li>
<li>Compatible (tested on IE6+, Chrome and Firefox)</li>
</ul>
</div>
</div>
Install with npm install fill
or get the
compiled and minified version
and include it to your application. jQuery is optional, but if you happen to
use it, fill registers itself as a plugin.
<script src="js/jquery-1.7.1.min.js"></script>
<script src="js/fill.min.js"></script>
For server-side use, see spec
folder and the awesome
jsdom for the details.
fill binds JavaScript objects to DOM a element by id
, class
,element name
,
name
attribute and data-bind
attribute. Values are escaped before rendering.
Any keys that are prefixed with an underscore are treated as attributes (with
the exception of _text and _html).
Template:
<div id="container">
<div id="hello"></div>
<div class="goodbye"></div>
<span></span>
<input type="text" name="greeting" />
<button class="hi-button" data-bind="hi-label"></button>
</div>
Javascript:
var hello = {
_class: 'message',
hello: 'Hello',
goodbye: 'Goodbye!',
span: '<i>See Ya!</i>',
greeting: 'Howdy!',
'hi-label': 'Terve!' // Finnish i18n
};
// with jQuery
$('#container').fill(hello);
// ..or without
fill(document.getElementById('container'), hello);
Result:
<div id="container" class="message">
<div id="hello">Hello</div>
<div class="goodbye">Goodbye!</div>
<span><i>See Ya!</i></span>
<input type="text" name="greeting" value="Howdy!" />
<button class="hi-button" data-bind="hi-label">Terve!</button>
</div>
Template:
<ul id="activities">
<li class="activity"></li>
</ul>
Javascript:
var activities = {
activity: [
'Jogging',
'Gym',
'Sky Diving'
]
};
$('#activities').fill(activities);
// or
fill(document.getElementById('activities'), activities);
Result:
<ul id="activities">
<li class="activity">Jogging</li>
<li class="activity">Gym</li>
<li class="activity">Sky Diving</li>
</ul>
Template:
<div>
<div class="comments">
<div class="comment">
<label>comment</label><span class="body"></span>
</div>
</div>
</div>
Javascript:
var comments = [
{body: "That rules"},
{body: "Great post!"}
]
$('.comment').fill(comments);
Result:
<div>
<div class="comments">
<div class="comment">
<label>comment</label><span class="body">That rules</span>
</div>
<div class="comment">
<label>comment</label><span class="body">Great post!</span>
</div>
</div>
</div>
Template:
<div class="container">
<h1 class="title"></h1>
<p class="post"></p>
<div class="comments">
<div class="comment">
<span class="name"></span>
<span class="text"></span>
</div>
</div>
</div>
Javascript:
var post = {
title: 'Hello World',
post: 'Hi there it is me',
comment: [ {
name: 'John',
text: 'That rules'
}, {
name: 'Arnold',
text: 'Great post!'
}
]
};
$('.container').fill(post);
Result:
<div class="container">
<h1 class="title">Hello World</h1>
<p class="post">Hi there it is me</p>
<div class="comments">
<div class="comment">
<span class="name">John</span>
<span class="text">That rules</span>
</div>
<div class="comment">
<span class="name">Arnold</span>
<span class="text">Great post!</span>
</div>
</div>
</div>
Template:
<div class="person">
<div class="firstname"></div>
<div class="lastname"></div>
<div class="address">
<div class="street"></div>
<div class="zip"><span class="city"></span></div>
</div>
</div>
Javascript:
var person = {
firstname: 'John',
lastname: 'Wayne',
address: {
street: '4th Street',
city: 'San Francisco',
zip: '94199'
}
};
$('.person').fill(person);
Result:
<div class="container">
<div class="firstname">John</div>
<div class="lastname">Wayne</div>
<div class="address">
<div class="street">4th Street</div>
<div class="zip">94199<span class="city">San Francisco</span></div>
</div>
</div>
Template:
<div class="container">
<div>
<span class="hello"></span>
</div>
<div>
<span class="hello"></span>
</div>
<span class="hello"></span>
</div>
Javascript:
// prefix with a dollar sign to find all matches
// otherwise it will only find the first one
var post = {
$hello: 'hi'
};
$('.container').fill(post);
Result:
<div class="container">
<div>
<span class="hello">hi</span>
</div>
<div>
<span class="hello">hi</span>
</div>
<span class="hello">hi</span>
</div>
You need node.js 0.6.x and npm.
Install dependencies:
npm install
npm install -g uglify-js
Run tests
npm test
Run tests during development for more verbose assertion output
node_modules/jasmine-node/bin/jasmine-node --verbose spec
Generate Javascript libs
node build
All the following are appreciated, in an asceding order of preference
In case the contribution is going to change fill API, please create a ticket first in order to discuss and agree on design.
This project was forked from the very impressive Transparency project to attempt the following:
FAQs
Templating with nothing but javascript objects and HTML.
We found that fill demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.