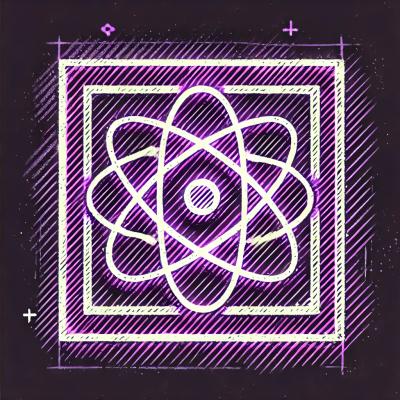
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
first-chunk-stream
Advanced tools
The 'first-chunk-stream' npm package is a Node.js module that allows you to transform the first chunk of a stream. This can be particularly useful for tasks that require modifying or inspecting the beginning of a stream before processing the rest, such as adjusting headers, parsing initial metadata, or prepending data to a stream.
Modify the first chunk of a stream
This code sample demonstrates how to use first-chunk-stream to modify the first 10 bytes of data from a file stream. It prepends the text 'Modified: ' to the beginning of the first chunk.
const firstChunkStream = require('first-chunk-stream');
const fs = require('fs');
const modifyFirstChunk = firstChunkStream({ chunkSize: 10 }, (chunk, enc, callback) => {
const modifiedChunk = Buffer.from(`Modified: ${chunk.toString()}`);
callback(null, modifiedChunk);
});
fs.createReadStream('example.txt')
.pipe(modifyFirstChunk)
.pipe(fs.createWriteStream('modifiedExample.txt'));
Inspect and conditionally modify the first chunk
This example shows how to inspect the first 15 bytes of a stream and modify it only if it contains the word 'Important'. If the condition is met, it prepends 'Alert: ' to the chunk.
const firstChunkStream = require('first-chunk-stream');
const fs = require('fs');
const inspectFirstChunk = firstChunkStream({ chunkSize: 15 }, (chunk, enc, callback) => {
if (chunk.toString().includes('Important')) {
callback(null, Buffer.from(`Alert: ${chunk.toString()}`));
} else {
callback(null, chunk);
}
});
fs.createReadStream('example.txt')
.pipe(inspectFirstChunk)
.pipe(fs.createWriteStream('inspectedExample.txt'));
Through2 is a tiny wrapper around Node streams.Transform, making it easier to create transform streams. It is similar to first-chunk-stream but offers more general functionality for transforming streams, not limited to just the first chunk.
Peek-stream allows you to peek at the first few bytes of a stream to determine how to parse it, then lets the stream continue unmodified or modified. It is similar to first-chunk-stream but focuses on peeking rather than modifying.
Buffer and transform the n first bytes of a stream
npm install first-chunk-stream
import fs from 'node:fs';
import getStream from 'get-stream';
import FirstChunkStream from 'first-chunk-stream';
import {uint8ArrayToString} from 'uint8array-extras';
// unicorn.txt => unicorn rainbow
const stream = fs.createReadStream('unicorn.txt')
.pipe(new FirstChunkStream({chunkSize: 7}, async (chunk, encoding) => {
return uint8ArrayToString(chunk).toUpperCase();
}));
const data = await getStream(stream);
if (data.length < 7) {
throw new Error('Couldn\'t get the minimum required first chunk length');
}
console.log(data);
//=> 'UNICORN rainbow'
FirstChunkStream
constructor.
Type: Function
Async function that receives the required options.chunkSize
bytes.
Expected to return an buffer-like object or string
or object of form {buffer: Uint8Array
, encoding: string
} to send to stream or firstChunkStream.stop
to end stream right away.
An error thrown from this function will be emitted as stream errors.
Note that the buffer can have a smaller length than the required one. In that case, it will be due to the fact that the complete stream contents has a length less than the options.chunkSize
value. You should check for this yourself if you strictly depend on the length.
import FirstChunkStream from 'first-chunk-stream';
new FirstChunkStream({chunkSize: 7}, async (chunk, encoding) => {
return chunk.toString(encoding).toUpperCase(); // Send string to stream
});
new FirstChunkStream({chunkSize: 7}, async (chunk, encoding) => {
return chunk; // Send buffer to stream
});
new FirstChunkStream({chunkSize: 7}, async (chunk, encoding) => {
return {
buffer: chunk,
encoding: encoding,
}; // Send buffer with encoding to stream
});
new FirstChunkStream({chunkSize: 7}, async (chunk, encoding) => {
return FirstChunkStream.stop; // End the stream early
});
new FirstChunkStream({chunkSize: 7}, async (chunk, encoding) => {
throw new Error('Unconditional error'); // Emit stream error
});
Type: object
The options object is passed to the Duplex
stream constructor allowing you to customize your stream behavior. In addition, you can specify the following option:
Type: number
The number of bytes to buffer.
FAQs
Buffer and transform the n first bytes of a stream
The npm package first-chunk-stream receives a total of 0 weekly downloads. As such, first-chunk-stream popularity was classified as not popular.
We found that first-chunk-stream demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.