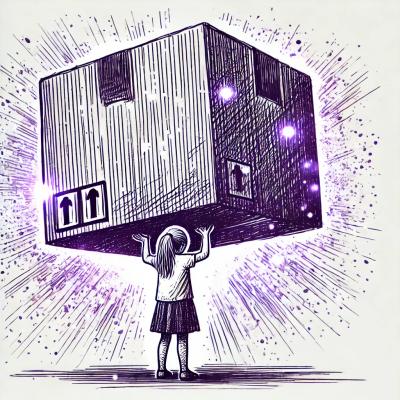
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
flat-embed
Advanced tools
Use this JavaScript Client to interact and receive events from our Sheet Music Embed.
This API requires an API Key, please contact us for more information.
You can install our Embed Client using npm or bower:
npm install flat-embed
or
bower install flat-embed
or use the latest version hosted on jsDelivr:
<script src="https://cdn.jsdelivr.net/flat-embed/0.3.0/embed.min.js"></script>
The simplest way to get started is the pass a DOM element to our embed that will be used as container. By default, this one will completely fit its container:
<div id="embed-container"></div>
<script src="https://cdn.jsdelivr.net/flat-embed/0.3.0/embed.min.js"></script>
<script>
var container = document.getElementById('embed-container');
var embed = new Flat.Embed(container, {
score: '<score-id-you-want-to-load>',
embedParams: {
appId: '<your-app-id>',
controlsFloating: false
}
});
</script>
When instantiating Flat.Embed
, the first argument will always refer to a DOM element. It can take:
document.getElementById('embed-container')
)."embed-container"
).$('#embed-container')
). If multiple elements match the selection, the client will take the first one selected.jsapi=true
.If you instance different Flat.Embed
for the same element, you will always get the same instance of the object.
When instantiating Flat.Embed
, you can pass options in the second parameter. In order to use the different methods available and events subscriptions, you will need to pass at least embedParams.appId
.
Option | Description | Values | Default |
---|---|---|---|
score | The score identifier that will load initially | Unique score id | blank |
width | The width of your embed | A width of the embed | 100% |
height | The height of your embed | A height of the embed | 100% |
embedParams | Object containing the loading options for the embed | Any URL parameters | {} |
ready
: Wait until the JavaScript is readyon
: Subscribe to eventsoff
: Unsubscribe from eventsgetEmbedConfig
: Get the global config of the embedloadFlatScore
: Load a score hosted on FlatloadMusicXML
: Load MusicXML file (compressed or not)loadJSON
: Load Flat JSON filegetMusicXML
: Get the score in MusicXML (compressed or not)getJSON
: Get the score data in Flat JSON formatgetScoreMeta
: Get the metadata from the current score (for hosted scores)fullscreen
: Toggle fullscreen modeplay
: Start playbackpause
: Pause playbackstop
: Stop playbackprint
: Print the scoregetZoom
: Get the current display zoom ratiosetZoom
: Change the display zoom ratiogetAutoZoom
: Get the state of the auto-zoom modesetAutoZoom
: Enable or disable the auto-zoom modesetEditorConfig
: Set the config of the editoredit
: Make a modification to the documentscoreLoaded
: A new score has been loadedcursorPosition
: The cursor position changedrangeSelection
: The range selected changedfullscreen
: The fullscreen state changedprint
: The score was printedplay
: The score playback startedpause
: The score playback pausedstop
: The score playback stoppedplaybackPosition
: The playback slider position changededit
: An edition has been made to the documentYou can call the methods using any Flat.Embed
object. By default, the methods (except on
and off
) return a Promise that will be resolved once the method is called, the value is set or get:
var embed = new Flat.Embed('container');
embed.loadFlatScore('12234').then(function () {
console.log('Score loaded');
}).catch(function (err) {
console.log('Error', err);
});
ready(): Promise<void, Error>
Promises resolved when the embed is loaded and the JavaScript API is ready to use. All the methods will implicitly use this method, so you don't have to worry about waiting the loading before calling the different methods.
embed.ready().then(function() {
// You can use the embed
});
on(event: string, callback: function): void
Add an event listener for the specified event. When receiving the event, the client will call the specified function with zero or one parameters depending of the event received.
embed.on('playbackPosition', function (position) {
console.log(position);
});
off(event: string, callback?: function): void
Remove an event listener for the specified event. If no callback
is specified, all the listener for the event will be removed.
function positionChanged(position) {
// Print position
console.log(position);
// You can remove the listener later (e.g. here, once the first event is received)
embed.off('play', positionChanged);
// Alternatively, you can remove all the listeners for the event:
embed.off('play');
};
// Subscribe to the event
embed.on('positionChanged', positionChanged);
getEmbedConfig(): Promise<object, Error>
Fetch the global config of the embed. This will include the URL parameters, the editor config and the default config set by the embed.
embed.getEmbedConfig().then(function (config) {
// The config of the embed
console.log(config);
});
loadFlatScore(id: string): Promise<void, ApiError>
Load a score hosted on Flat using its identifier. For example to load https://flat.io/score/56ae21579a127715a02901a6-house-of-the-rising-sun
, you can call:
embed.loadFlatScore('56ae21579a127715a02901a6').then(function () {
// Score loaded in the embed
}).catch(function (error) {
// Unable to load the score
});
loadMusicXML(score: mixed): Promise<void, Error>
Load a MusicXML score, compressed (MXL) or not (plain XML):
fetch('https://api.flat.io/v2/scores/56ae21579a127715a02901a6/revisions/last/mxl')
.then(function (response) {
return response.arrayBuffer();
})
.then(function (mxl) {
return embed.loadMusicXML(mxl);
})
.then(function () {
// Score loaded in the embed
})
.catch(function (error) {
// Unable to load the score
});
loadJSON(score: object): Promise<void, Error>
Load a score using Flat's JSON Format
fetch('https://api.flat.io/v2/scores/56ae21579a127715a02901a6/revisions/last/json')
.then(function (response) {
return response.json();
})
.then(function (json) {
return embed.loadJSON(json);
})
.then(function () {
// Score loaded in the embed
})
.catch(function (error) {
// Unable to load the score
});
getMusicXML(options?: object): Promise<string|Uint8Array, Error>
Convert the current displayed score into a MusicXML file, compressed (.mxl
) or not (.xml
).
// Uncompressed MusicXML
embed.getMusicXML().then(function (xml) {
// Plain XML file (string)
console.log(xml);
});
Example: Retrieve the score as a compressed MusicXML, then convert it to a Blob and download it:
// Uncompressed MusicXML
embed.getMusicXML({ compressed: true }).then(function (buffer) {
// Create a Blob from a compressed MusicXML file (Uint8Array)
var blobUrl = window.URL.createObjectURL(new Blob([buffer], {
type: 'application/vnd.recordare.musicxml+xml'
}));
// Create a hidden link to download the blob
var a = document.createElement('a');
a.href = blobUrl;
a.download = 'My Music Score.mxl';
document.body.appendChild(a);
a.style = 'display: none';
a.click();
a.remove();
});
getJSON(): object
Get the data of the score in the "Flat JSON" format (a MusicXML-like as a JavaScript object).
embed.getJSON().then(function (data) {
console.log(data);
}).catch(function (error) {
// Unable to get the data
});
getScoreMeta(): object
Get the score metadata of the hosted score. The object will have the same format that the one returned by our API GET /v2/scores/{score}
.
embed.getScoreMeta().then(function (metadata) {
console.log(metadata);
}).catch(function (error) {
// Unable to get the metadata
});
fullscreen(state: bool): Promise<void, Error>
Display the embed in fullscreen (state = true
) or return to the regular display (state = false
).
embed.fullscreen(true).then(function () {
// The score is now displayed in fullscreen
});
play(): Promise<void, Error>
Load the playback and play the score.
embed.play().then(function () {
// The score is playing
});
pause(): Promise<void, Error>
Pause the playback
embed.pause().then(function () {
// The playback is paused
});
stop(): Promise<void, Error>
Stop the playback
embed.stop().then(function () {
// The playback is stopped
});
print(): Promise<void, Error>
Print the score
embed.print().then(function () {
// The score is being printed (the browser opens the print prompt)
}).catch(function (error) {
// Error when printing
});
getZoom(): Promise<number, Error>
Get the current zoom ration applied for the score (between 0.5 and 3).
embed.getZoom().then(function (zoom) {
// The zoom value
console.log(zoom);
});
setZoom(number): Promise<number, Error>
Set a new zoom ration for the display (between 0.5 and 3).
embed.setZoom(2).then(function (zoom) {
// The zoom value applied
console.log(zoom);
});
getAutoZoom(): Promise(<boolean, Error>)
Get the state of the auto-zoom mode. Auto-zoom is enabled by default for page mode or when the URL parameter zoom
is set to auto
.
This getter will return true
if the auto-zoom is enabled, and false
when disabled. Setting a zoom value with setZoom
will disable this mode.
embed.getAutoZoom().then(function (state) {
// The auto-zoom state
console.log(state);
});
setAutoZoom(boolean): Promise(<boolean, Error>)
Enable (true
) or disable (false
) the auto-zoom. Auto-zoom is enabled by default for page mode or when the URL parameter zoom
is set to auto
. Setting a zoom value with setZoom
will disable this mode.
embed.setAutoZoom(false).then(function (state) {
// Auto-zoom mode is disabled
console.log(state);
});
You can enable the editor mode by setting the mode
to edit
when creating the embed:
var embed = new Flat.Embed(container, {
embedParams: {
appId: '<your-app-id>',
modeL 'edit'
}
});
setEditorConfig(config: object): Promise<object, Error>
Set a new config for the editor (e.g. the different tools available in the embed). This one will be used at the next loading score.
// For example: hide the Articulation mode, and only display the durations tools in the Note mode
embed.setEditorConfig({
noteMode: {
durations: true
},
articulationMode: false
}).then(function (config) {
// The config of the embed
console.log(config);
});
edit(operations: object): Promise<void, Error>
Process some edit operations using one of our internal editing method. Feel free to contact our developers support to get more information about the operations available.
embed.edit([
{ name: 'action.SetTitle', opts: { title: 'I <3 Flat'} }
]).then(function () {
// The actions have been executed
}).catch(function (error) {
// Error while executing the actions
});
Events are broadcasted following actions made by the end user or you with the JavaScript API. You can subscribe to an event using the method on
, and unsubscribe using off
. When an event includes some data, this data will be available in the first parameter of the listener callback.
scoreLoaded
This event is triggered once a new score has been loaded. This event doesn't include any data.
cursorPosition
This event is triggered when the position of the user's cursor changes.
{
"partIdx": 0,
"staffIdx": 1,
"voiceIdx": 0,
"measureIdx": 2,
"noteIdx": 1
}
rangeSelection
This event is triggered when a range of notes is selected or the selection changed.
{
"from": {
"partIdx": 0,
"measureIdx": 1,
"staffIdx": 0,
"voiceIdx": 0,
"noteIdx": 2
},
"to": {
"partIdx": 0,
"measureIdx": 3,
"staffIdx": 0,
"voiceIdx": 0,
"noteIdx": 5
}
}
fullscreen
This event is triggered when the state of the fullscreen changed. The callback will take a boolean as the first parameter that will be true
if the fullscreen mode is enabled, and false
is the display is back to normal (fullscreen exited).
print
This event is triggered when you or the end-user prints the score. This event doesn't include any data.
play
This event is triggered when you or the end-user starts the playback. This event doesn't include any data.
pause
This event is triggered when you or the end-user pauses the playback. This event doesn't include any data.
stop
This event is triggered when you or the end-user stops the playback. This event doesn't include any data.
playbackPosition
This event is triggered when the playback slider moves. It is usually triggered at the beginning of every measure and will contain an object with information regarding the position of the playback in the score:
{
"beat": "4",
"beatType": "4",
"tempo": 120,
"currentMeasure": 1,
"timePerMeasure": 2
}
edit
This event is triggered when one or multiple modifications ave been made to the document. This one will contain a list of operations made:
[
{
"name": "action.SetTempo",
"opts": {
"startMeasureIdx": 0,
"stopMeasureIdx": 1,
"tempo": {
"bpm": 142,
"qpm": 142,
"durationType": 3,
"nbDots": 0
}
}
}
]
2017-05-03: v0.3.0
getEmbedConfig
, setEditorConfig
, edit
edit
FAQs
Interact and get events from Flat's Sheet Music Embed
The npm package flat-embed receives a total of 53 weekly downloads. As such, flat-embed popularity was classified as not popular.
We found that flat-embed demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.