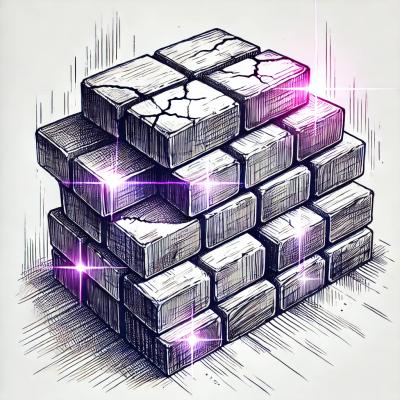
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
flixhq-core
Advanced tools
Nodejs library that provides an Api for obtaining the movies information from FlixHQ website.
Nodejs library that provides an Api for obtaining the movies information from FlixHQ website.
import { MOVIES } from 'flixhq-core'
const flixhq = new MOVIES.FlixHQ();
Install with npm
npm install flixhq-core
Fetch data of the FlixHQ homepage.
// Promise:
flixhq.home().then(data => console.log(data));
// Async/Await:
(async () => {
const data = await flixhq.home();
console.log(data);
})();
returns a promise which resolves into an object. (Promise<IHomeResult>
).
// Promise:
flixhq.fetchGenresList().then(data => console.log(data));
// Async/Await:
(async () => {
const data = await flixhq.fetchGenresList();
console.log(data);
})();
returns a promise which resolves into an array of genres. (Promise<IGenre[]>
).
// Promise:
flixhq.fetchCountriesList().then(data => console.log(data));
// Async/Await:
(async () => {
const data = await flixhq.fetchCountriesList();
console.log(data);
})();
returns a promise which resolves into an array of countries. (Promise<ICountry[]>
).
Parameter | Type | Description |
---|---|---|
filterBy | Filters | Accept: "GENRE" or "COUNTRY". |
query | string | query that depend on the filterBy parameter. (genre or country that can be found in the genres or countries list). |
page (optional) | number | page number (default: 1). |
// Promise:
flixhq.fetchMovieByCountryOrGenre(Filter.COUNTRY, "US").then(data => console.log(data));
// Async/Await:
(async () => {
const data = await flixhq.fetchMovieByCountryOrGenre(Filter.COUNTRY, "US");
console.log(data);
})();
returns a promise which resolves into an array of movies/tvseries. (Promise<ISearch<IMovieResult>>
).
Parameter | Type | Description |
---|---|---|
type | MovieType | Accept: "MOVIE" or "TVSERIES". |
page (optional) | number | page number (default: 1). |
// Promise:
flixhq.fetchMovieByType(MovieType.MOVIE).then(data => console.log(data));
// Async/Await:
(async () => {
const data = await flixhq.fetchMovieByType(MovieType.MOVIE);
console.log(data);
})();
returns a promise which resolves into an array of movies. (Promise<ISearch<IMovieResult>>
).
Parameter | Type | Description |
---|---|---|
type (optional) | MovieType | Accept: "MOVIE" or "TVSERIES" (default: "ALL"). |
page (optional) | number | page number (default: 1). |
// Promise:
flixhq.fetchMovieByTopIMDB().then(data => console.log(data));
// Async/Await:
(async () => {
const data = await flixhq.fetchMovieByTopIMDB();
console.log(data);
})();
returns a promise which resolves into an array of movies/tvseries. (Promise<ISearch<IMovieResult>>
).
Parameter | Type | Description |
---|---|---|
mediaId | string | (can be found in the media search results.). |
// Promise:
flixhq.fetchMovieInfo("movie/watch-m3gan-91330").then(data => console.log(data));
// Async/Await:
(async () => {
const data = await flixhq.fetchMovieInfo("movie/watch-m3gan-91330");
console.log(data);
})();
returns a promise which resolves into an object of movie info. (Promise<IMovieInfo>
).
Parameter | Type | Description |
---|---|---|
mediaId | string | (can be found in the media search results.). |
episodeId | string | (can be found in the media info results as shown on the above method). |
// Promise:
flixhq.fetchEpisodeServers("movie/watch-m3gan-91330", "91330").then(data => console.log(data));
// Async/Await:
(async () => {
const data = await flixhq.fetchEpisodeServers("movie/watch-m3gan-91330", "91330");
console.log(data);
})();
returns a promise which resolves into an array of the servers info. (Promise<IEpisodeServer>
).
Parameter | Type | Description |
---|---|---|
mediaId | string | (can be found in the media search results.). |
episodeId | string | (can be found in the media info results as shown on the above method). |
server (optional) | StreamingServers | Accept: "UpCloud" or "VidCloud" or "MixDrop" (default: "UpCloud"). |
// Promsie:
flixhq.fetchEpisodeSources("movie/watch-m3gan-91330", "91330").then(data => console.log(data));
// Async/Await:
(async () => {
const data = await flixhq.fetchEpisodeSources("movie/watch-m3gan-91330", "91330");
console.log(data);
})();
returns a promise which resolves into an object of media sources and subtitles.
Parameter | Type | Description |
---|---|---|
query | string | movie or tvseries name. |
page (optional) | number | page number (default: 1). |
// Promise:
flixhq.search("the last of us").then(data => console.log(data));
// Async/Await:
(async () => {
const data = await flixhq.search("the last of us");
console.log(data);
})();
returns a promise which resolves into an array of movies/tvseries. (Promise<ISearch<IMovieResult>>
).
// Promise:
flixhq. fetchFiltersList().then(data => console.log(data));
// Async/AwaitL
(async () => {
const data = await flixhq.fetchFiltersList();
console.log(data);
})();
returns a promise which resolves into an object of filters info. (Promise<IMovieFilter>
).
Parameter | Type | Description |
---|---|---|
options | IMovieFilter | (Includes: type, quality, released, genre, country. Can be found in the filters list as shown on the above method.) |
page (optional) | number | page number (default: 1). |
// Promise:
const options = { type: 'all', quality: 'all', released: 'all', genre: 'all', country: 'all' };
flixhq.filter(options).then(data => console.log(data));
// Async/Await:
(async () => {
const data = await flixhq.filter(options);
console.log(data);
})();
returns a promise which resolves into an array of movies/tvseries. (Promise<ISearch<IMovieResult>>
).
FAQs
Nodejs library that provides an Api for obtaining the movies information from FlixHQ website.
The npm package flixhq-core receives a total of 27 weekly downloads. As such, flixhq-core popularity was classified as not popular.
We found that flixhq-core demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.