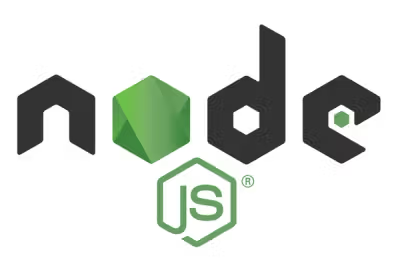
Security News
require(esm) Backported to Node.js 20, Paving the Way for ESM-Only Packages
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Fluorite is a lightweight ORM based on Knex.js query builder. It features promise based interface, provides transactions support, bulk updating and deleting, and support for one-to-one, one-to-many and many-to-many relations.
node 8+
npm install fluorite
First of all you'll need a copy of knex.js
query builder to be configured.
Next, you'll need to create a database representing your domain model, and
then create models.
const knex = require('knex')({
client: 'sqlite3',
connection: {
filename: './db.sqlite3',
},
});
await knex.schema.createTable('users', (table) => {
table.increments();
table.string('name');
table.number('age').unsigned();
});
await knex.schema.createTable('posts', (table) => {
table.increments();
table.string('content');
table.integer('user_id').unsigned().references('users.id');
});
const fluorite = require('fluorite')(knex);
class User extends fluorite.Model {
static table = 'users';
posts() {
return this.hasMany(Post);
}
}
class Post extends fluorite.Model {
static table = 'posts';
author() {
return this.belongsTo(User);
}
}
You should use the fluorite
instance returned throughout your library
because it creates a connection pool for the current database.
To create an model object, instantiate it with object representing attributes and then call save()
.
const user = new User({ name: 'John Doe', age: 28 });
await user.save();
To save changes to an object that is already in the database, call object's method save()
.
user.set('age', 29);
await user.save();
You also can also pass an object with attributes to set
method:
user.set({ age: 29, name: 'Bob Doe' });
await user.save();
Or shorthand 'set and update':
await user.save({ age: 29, name: 'Bob Doe' });
To delete object from database use method remove()
.
await user.remove();
Each Model has objects
property that by default returns new MultipleRowsQuery
object that
can be used to retrieve or bulk update group of objects.
To retrieve all objects use async/await
or then
promise syntax on the query:
const users = await User.objects();
// or
User.objects().then(users => console.log(users));
You can also use experimental asyncInterator
syntax to iterate over database rows:
for await (const user of User.objects()) {
console.log(user.get('name'));
}
To filter query result use method filter()
passing to it object with attributes for refining.
const men = await User.objects().filter({ gender: 'male' });
By default used =
operator for comparing. But you alter this behavior.
Just add double underscore and operator name after property name (example: age__gt
).
eq
evaluates to =ne
evaluates to !=gt
evaluates to >gte
evaluates to >=lt
evaluates to <lte
evaluates to <=in
evaluates to INlike
evaluates to LIKEconst adults = await User.objects().filter({ age__gte: 18 });
const users = await User.objects().filter({ id__in: [1, 2, 3] });
const irish = await User.objects().filter({ name__like: 'Mac%' });
const adultFemales = await User.objects
.filter({ age__gte: 18 })
.filter({ gender: 'female' });
All filters are immutable. Each time you refine your criteria you get new copy of query.
All filters are lazy. It means that query will run only when you call then
or iterate
over query.
To limit amount of objects to be returned use limit()
.
You could also use offset()
to specify offset for objects query.
const firstFiveUsers = await User.objects().limit(5);
const nextFiveUsers = await User.objects().limit(5).offset(5);
There are three different ways to retrieve single object from database.
const user = await User.find(5);
const user = await User.objects().single({ name: 'John Doe' });
It will fail with User.IntegrityError
if SQL statement returned more than one row.
const user = await User.objects().first({ name: 'John Doe' });
If object matching your criteria does not exist Model.NotFoundError
will be thrown.
Use of transactions is very simple:
import { fluorite } from 'fluorite';
await fluorite.transaction(async () => {
const user = await User.find(10);
await user.save({ name: 'John Doe' });
});
You can nest transactions as many as your database supports.
FAQs
Lightweight ORM based on Knex.js query builder.
The npm package fluorite receives a total of 2 weekly downloads. As such, fluorite popularity was classified as not popular.
We found that fluorite demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.