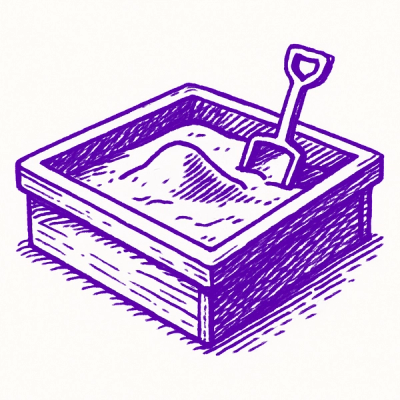
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
fmrs
is a collection of utility functions that filter/find, map, reduce,
and sort JavaScript primitives and built-ins between each other.
For example:
true
to a string would be 'true'
.[['key', 'value']]
to a record would be
{ key: 'value' }
.1
and 2
would be -1
.value is boolean
)
arr.every(isBoolean)
arr.filter(filterByBoolean)
arr.find(findBoolean)
arr.every(isDefined)
arr.filter(filterByDefined)
arr.find(findDefined)
value is number
)
arr.every(isNumber)
arr.filter(filterByNumber)
arr.find(findNumber)
value is object
)
arr.every(isObject)
arr.filter(filterByObject)
arr.find(findObject)
value is Record<number | string | symbol, unknown>
)
arr.every(isRecord)
arr.filter(filterByRecord)
arr.find(findRecord)
arr.every(isString)
arr.filter(filterByString)
arr.find(findString)
value is undefined
)
arr.every(isUndefined)
arr.filter(filterByUndefined)
arr.find(findUndefined)
from | to | example |
---|---|---|
boolean | number | [false, true].map(mapBooleanToNumber) |
entries | Record ({} ) | mapEntriesToRecord([['key', 'value']]) |
entry | key | mapEntryToKey(['key', 'value']) |
entry | value | mapEntryToValue(['key', 'value']) |
Map | entries | mapMapToEntries(new Map()) |
Map | Record ({} ) | mapMapToRecord(new Map()) |
unknown | Error | arr.map(mapToError) |
unknown | index | arr.map(mapToIndex) |
unknown | string | arr.map(mapToString) |
from | to | example |
---|---|---|
entries | Record ({} ) | Object.entries(record).reduce(reduceEntriesToRecord, {}) |
items | example |
---|---|
Array | arrOfArrs.sort(sortByIndex(0)) |
number | arr.sort(sortNumbers) |
string | arr.sort(sortStrings) |
unknown | arr.sort(sort) |
function | example |
---|---|
is | ['a', 'b'].filter(is('a')) |
isNot | ['a', 'b'].filter(isNot('a')) |
not | ['a', 'b'].filter(not(is('a'))) |
FAQs
filters/finds, maps, reduces, and sorts
The npm package fmrs receives a total of 0 weekly downloads. As such, fmrs popularity was classified as not popular.
We found that fmrs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.