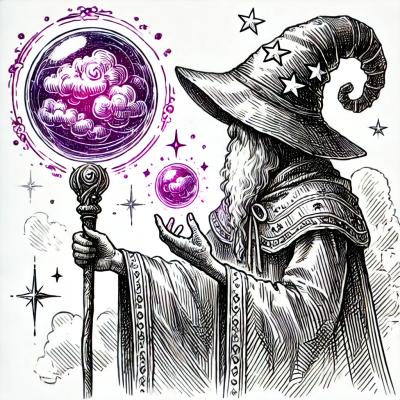
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
focus-trap
Advanced tools
The focus-trap npm package is designed to trap focus within a specified DOM element. This is particularly useful for accessibility in modal dialogs, ensuring that keyboard users do not accidentally move focus outside of the dialog, thereby improving the user experience and accessibility compliance.
Create and activate a focus trap
This code demonstrates how to create and activate a focus trap within a specified DOM element, typically a modal dialog. It ensures that all keyboard navigation remains within the 'modal' element.
const { createFocusTrap } = require('focus-trap');
const container = document.getElementById('modal');
const focusTrap = createFocusTrap(container);
focusTrap.activate();
Deactivate a focus trap
This code snippet shows how to deactivate a previously activated focus trap, allowing focus to move freely outside the trapped container once again.
focusTrap.deactivate();
Set return focus on deactivation
This feature allows setting a specific element to receive focus when the focus trap is deactivated. It enhances usability by returning focus to a logical location, such as a button that initially triggered the modal.
const focusTrap = createFocusTrap(container, {
onDeactivate: () => document.getElementById('triggerButton').focus()
});
focus-lock is similar to focus-trap in that it also provides functionality to trap focus within a DOM element. However, focus-lock offers additional features such as auto-focusing the first focusable element and integration with React components.
react-focus-lock is a React-specific implementation of focus trapping. It provides similar functionalities to focus-trap but is tailored specifically for use within React applications, making it easier to integrate with React component lifecycle.
Trap focus within a DOM node.
There may come a time when you find it important to trap focus within a DOM node — so that when a user hits Tab or Shift+Tab or clicks around, she can't escape a certain cycle of focusable elements.
You will definitely face this challenge when you are try to build an accessible modal or dropdown menu.
This module is a little vanilla JS solution to the problem.
If you are using React, check out focus-trap-react, a light wrapper around this library. If you are not a React user, consider creating light wrappers in your framework-of-choice!
When a focus trap is activated, this is what should happen:
When the focus trap is deactivated, this is what should happen:
npm install focus-trap
IE9+
Why?
Because this module uses EventTarget.addEventListener()
.
And its only dependency, tabbable, uses a couple of IE9+ functions.
The module exposes two functions.
Turn element
into a focus trap.
element
can be a DOM node (the focus trap itself) or a selector string
(which will be pass to document.querySelector()
to find the DOM node).
Options:
document.querySelector()
to find the DOM node).true
. If false
, the Escape key will not trigger deactivation of the focus trap. This can be useful if you want to force the user to make a decision instead of allowing an easy way out.false
. If true
, a click outside the focus trap will deactivate the focus trap and allow the click event to carry on.Simply deactivate any currently active focus trap.
Read code in demo/
(it's very simple), and see how it works.
Here's what happens in demo-one.js
:
var focusTrap = require('focus-trap');
var el = document.getElementById('demo-one');
document.getElementById('activate-one').addEventListener('click', function() {
focusTrap.activate(el, {
onDeactivate: removeActiveClass,
});
el.className = 'trap is-active';
});
document.getElementById('deactivate-one').addEventListener('click', function() {
focusTrap.deactivate();
removeActiveClass();
});
function removeActiveClass() {
el.className = 'trap';
}
Because of the nature of the functionality, involving keyboard and click and (especially) focus events, JavaScript unit tests didn't make sense. (If you disagree and can help out, please PR!) So the demo is also the test: run it in browsers and see how it works.
1.1.1
clickOutsideDeactivates
functionality.FAQs
Trap focus within a DOM node.
The npm package focus-trap receives a total of 1,402,543 weekly downloads. As such, focus-trap popularity was classified as popular.
We found that focus-trap demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.