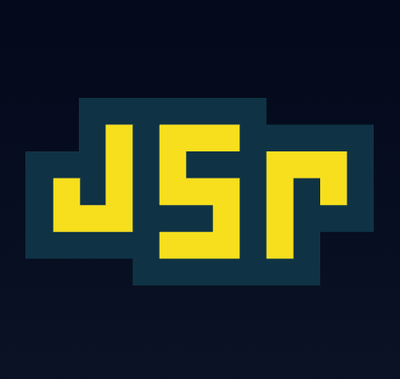
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
fork-ts-checker-webpack-plugin
Advanced tools
Runs typescript type checker and linter on separate process.
The fork-ts-checker-webpack-plugin is a plugin for webpack that runs TypeScript type checking on a separate process. This allows you to get type checking results while webpack compiles your code, improving build speed by utilizing multiple CPU cores. It also integrates with ESLint to provide linting for both TypeScript and JavaScript files.
TypeScript Type Checking
This feature runs TypeScript type checking in a separate process to speed up the webpack build.
const ForkTsCheckerWebpackPlugin = require('fork-ts-checker-webpack-plugin');
module.exports = {
plugins: [new ForkTsCheckerWebpackPlugin()],
};
ESLint Integration
This feature allows the plugin to run ESLint on your code in parallel with the TypeScript type checker.
const ForkTsCheckerWebpackPlugin = require('fork-ts-checker-webpack-plugin');
module.exports = {
plugins: [
new ForkTsCheckerWebpackPlugin({
eslint: {
files: './src/**/*.{ts,tsx,js,jsx}'
}
})
],
};
Asynchronous Reporting
This feature provides asynchronous reporting of type checking and linting results, so they do not block webpack's compilation process.
const ForkTsCheckerWebpackPlugin = require('fork-ts-checker-webpack-plugin');
const webpack = require('webpack');
const compiler = webpack({
// ...webpack configuration
plugins: [new ForkTsCheckerWebpackPlugin()],
});
compiler.run((error, stats) => {
// ...handle webpack build result
});
ts-loader is a TypeScript loader for webpack. It is responsible for compiling TypeScript files to JavaScript. Unlike fork-ts-checker-webpack-plugin, ts-loader does the type checking in the main compilation process, which can be slower for large projects.
awesome-typescript-loader is another TypeScript loader for webpack. Similar to ts-loader, it provides compilation of TypeScript files. It also offers features like Babel integration and can use a separate process for type checking, but it is not as actively maintained as fork-ts-checker-webpack-plugin.
eslint-webpack-plugin is a plugin for webpack that provides linting functionality. It integrates ESLint into the webpack build process. While it does not offer TypeScript type checking, it serves a similar purpose for linting as fork-ts-checker-webpack-plugin does when the ESLint integration is enabled.
Webpack plugin that runs TypeScript type checker on a separate process.
This plugin requires Node.js >=12.13.0+, Webpack ^5.11.0, TypeScript ^3.6.0
# with npm
npm install --save-dev fork-ts-checker-webpack-plugin
# with yarn
yarn add --dev fork-ts-checker-webpack-plugin
The minimal webpack config (with ts-loader)
// webpack.config.js
const ForkTsCheckerWebpackPlugin = require('fork-ts-checker-webpack-plugin');
module.exports = {
context: __dirname, // to automatically find tsconfig.json
entry: './src/index.ts',
resolve: {
extensions: [".ts", ".tsx", ".js"],
},
module: {
rules: [
{
test: /\.tsx?$/,
loader: 'ts-loader',
// add transpileOnly option if you use ts-loader < 9.3.0
// options: {
// transpileOnly: true
// }
}
]
},
plugins: [new ForkTsCheckerWebpackPlugin()],
watchOptions: {
// for some systems, watching many files can result in a lot of CPU or memory usage
// https://webpack.js.org/configuration/watch/#watchoptionsignored
// don't use this pattern, if you have a monorepo with linked packages
ignored: /node_modules/,
},
};
Examples how to configure it with babel-loader, ts-loader and Visual Studio Code are in the examples directory.
It's very important to be aware that this plugin uses TypeScript's, not
webpack's modules resolution. It means that you have to setup tsconfig.json
correctly.
It's because of the performance - with TypeScript's module resolution we don't have to wait for webpack to compile files.
To debug TypeScript's modules resolution, you can use
tsc --traceResolution
command.
This plugin uses cosmiconfig
. This means that besides the plugin constructor,
you can place your configuration in the:
"fork-ts-checker"
field in the package.json
.fork-ts-checkerrc
file in JSON or YAML formatfork-ts-checker.config.js
file exporting a JS objectOptions passed to the plugin constructor will overwrite options from the cosmiconfig (using deepmerge).
Name | Type | Default value | Description |
---|---|---|---|
async | boolean | compiler.options.mode === 'development' | If true , reports issues after webpack's compilation is done. Thanks to that it doesn't block the compilation. Used only in the watch mode. |
typescript | object | {} | See TypeScript options. |
issue | object | {} | See Issues options. |
formatter | string or object or function | codeframe | Available formatters are basic , codeframe and a custom function . To configure codeframe formatter, pass: { type: 'codeframe', options: { <coderame options> } } . To use absolute file path, pass: { type: 'codeframe', pathType: 'absolute' } . |
logger | { log: function, error: function } or webpack-infrastructure | console | Console-like object to print issues in async mode. |
devServer | boolean | true | If set to false , errors will not be reported to Webpack Dev Server. |
Options for the TypeScript checker (typescript
option object).
Name | Type | Default value | Description |
---|---|---|---|
memoryLimit | number | 2048 | Memory limit for the checker process in MB. If the process exits with the allocation failed error, try to increase this number. |
configFile | string | 'tsconfig.json' | Path to the tsconfig.json file (path relative to the compiler.options.context or absolute path) |
configOverwrite | object | { compilerOptions: { skipLibCheck: true, sourceMap: false, inlineSourceMap: false, declarationMap: false } } | This configuration will overwrite configuration from the tsconfig.json file. Supported fields are: extends , compilerOptions , include , exclude , files , and references . |
context | string | dirname(configuration.configFile) | The base path for finding files specified in the tsconfig.json . Same as the context option from the ts-loader. Useful if you want to keep your tsconfig.json in an external package. Keep in mind that not having a tsconfig.json in your project root can cause different behaviour between fork-ts-checker-webpack-plugin and tsc . When using editors like VS Code it is advised to add a tsconfig.json file to the root of the project and extend the config file referenced in option configFile . |
build | boolean | false | The equivalent of the --build flag for the tsc command. |
mode | 'readonly' or 'write-dts' or 'write-tsbuildinfo' or 'write-references' | build === true ? 'write-tsbuildinfo' ? 'readonly' | Use readonly if you don't want to write anything on the disk, write-dts to write only .d.ts files, write-tsbuildinfo to write only .tsbuildinfo files, write-references to write both .js and .d.ts files of project references (last 2 modes requires build: true ). |
diagnosticOptions | object | { syntactic: false, semantic: true, declaration: false, global: false } | Settings to select which diagnostics do we want to perform. |
profile | boolean | false | Measures and prints timings related to the TypeScript performance. |
typescriptPath | string | require.resolve('typescript') | If supplied this is a custom path where TypeScript can be found. |
Options for the issues filtering (issue
option object).
I could write some plain text explanation of these options but I think code will explain it better:
interface Issue {
severity: 'error' | 'warning';
code: string;
file?: string;
}
type IssueMatch = Partial<Issue>; // file field supports glob matching
type IssuePredicate = (issue: Issue) => boolean;
type IssueFilter = IssueMatch | IssuePredicate | (IssueMatch | IssuePredicate)[];
Name | Type | Default value | Description |
---|---|---|---|
include | IssueFilter | undefined | If object , defines issue properties that should be matched. If function , acts as a predicate where issue is an argument. |
exclude | IssueFilter | undefined | Same as include but issues that match this predicate will be excluded. |
Include issues from the src
directory, exclude issues from .spec.ts
files:
module.exports = {
// ...the webpack configuration
plugins: [
new ForkTsCheckerWebpackPlugin({
issue: {
include: [
{ file: '**/src/**/*' }
],
exclude: [
{ file: '**/*.spec.ts' }
]
}
})
]
};
This plugin provides some custom webpack hooks:
Hook key | Type | Params | Description |
---|---|---|---|
start | AsyncSeriesWaterfallHook | change, compilation | Starts issues checking for a compilation. It's an async waterfall hook, so you can modify the list of changed and removed files or delay the start of the service. |
waiting | SyncHook | compilation | Waiting for the issues checking. |
canceled | SyncHook | compilation | Issues checking for the compilation has been canceled. |
error | SyncHook | compilation | An error occurred during issues checking. |
issues | SyncWaterfallHook | issues, compilation | Issues have been received and will be reported. It's a waterfall hook, so you can modify the list of received issues. |
To access plugin hooks and tap into the event, we need to use the getCompilerHooks
static method.
When we call this method with a webpack compiler instance, it returns the object with
tapable hooks where you can pass in your callbacks.
// ./src/webpack/MyWebpackPlugin.js
const ForkTsCheckerWebpackPlugin = require('fork-ts-checker-webpack-plugin');
class MyWebpackPlugin {
apply(compiler) {
const hooks = ForkTsCheckerWebpackPlugin.getCompilerHooks(compiler);
// log some message on waiting
hooks.waiting.tap('MyPlugin', () => {
console.log('waiting for issues');
});
// don't show warnings
hooks.issues.tap('MyPlugin', (issues) =>
issues.filter((issue) => issue.severity === 'error')
);
}
}
module.exports = MyWebpackPlugin;
// webpack.config.js
const ForkTsCheckerWebpackPlugin = require('fork-ts-checker-webpack-plugin');
const MyWebpackPlugin = require('./src/webpack/MyWebpackPlugin');
module.exports = {
/* ... */
plugins: [
new ForkTsCheckerWebpackPlugin(),
new MyWebpackPlugin()
]
};
When using TypeScript 4.3.0 or newer you can profile long type checks by setting "generateTrace" compiler option. This is an instruction from microsoft/TypeScript#40063:
tsconfig.json
(under compilerOptions
)legend.json
telling you what went where.
Otherwise, there will be trace.json
file and types.json
files.trace.json
types.json
in an editorYou must both set "incremental": true in your tsconfig.json
(under compilerOptions
) and also specify mode: 'write-references' in ForkTsCheckerWebpackPlugin
settings.
ts-loader
- TypeScript loader for webpack.babel-loader
- Alternative TypeScript loader for webpack.fork-ts-checker-notifier-webpack-plugin
- Notifies about build status using system notifications (similar to the webpack-notifier).This plugin was created in Realytics in 2017. Thank you for supporting Open Source.
MIT License
FAQs
Runs typescript type checker and linter on separate process.
The npm package fork-ts-checker-webpack-plugin receives a total of 11,502,215 weekly downloads. As such, fork-ts-checker-webpack-plugin popularity was classified as popular.
We found that fork-ts-checker-webpack-plugin demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.