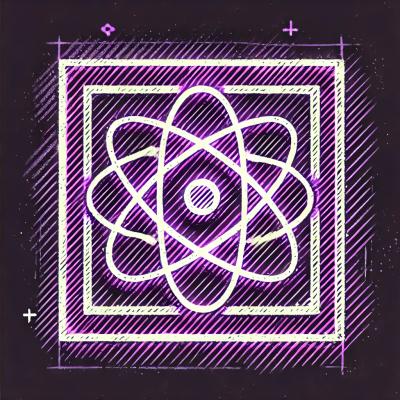
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
form-parser
Advanced tools
A streaming and asynchronous multipart/form-data
parser.
npm install form-parser --save
// Dependencies
const parser = require('form-parser')
const { send } = require('micro')
// Create server
module.exports = async (req, res) => {
// Parse request
await parser(req, async field => {
// Log info
console.log(field) // { fieldType, fieldName, fieldContent }
})
// Reply with finished
return send(res, 200, 'Parsing form succeded.')
}
// Dependencies
const http = require('http')
const parser = require('form-parser')
// Create server
const server = http.createServer(async (req, res) => {
// Wrap in try/catch block
try {
// Parse request
await parser(req, async field => {
// Log info
console.log(field) // { fieldType, fieldName, fieldContent }
})
// Catch errors
} catch (err) {
// Log error
console.error(err)
// Reply with error
res.statusCode = 400
return res.end('Something went wrong.')
}
// Reply with success
return res.end('Parsing form succeded.')
})
// Start server
server.listen(3000, () => {
console.log('Listening on port 3000...')
})
// Dependencies
const http = require('http')
const parser = require('form-parser')
const path = require('path')
const fs = require('fs')
// Create server
const server = http.createServer(async (req, res) => {
try {
// Parse request
await parser(req, async field => {
// Get info
const { fieldType, fieldName, fieldContent } = field
// Only handle files
if (fieldType !== 'file') {
return
}
// Get file info
const { fileName, fileType, fileStream } = fieldContent
// Prepare write stream
const writeFilePath = path.resolve(__dirname, 'files', fileName)
const writeFileStream = fs.createWriteStream(writeFilePath)
// Write file to disk
await new Promise((resolve, reject) => {
fileStream.pipe(writeFileStream).on('error', reject).on('finish', resolve)
})
// Log info
console.log(`${fileName} has been written to disk.`)
})
// Catch errors
} catch (err) {
// Log error
console.error(err)
// Reply with error
res.statusCode = 400
return res.end('Something went wrong.')
}
// Reply with success
return res.end('Parsing form succeded.')
})
// Start server
server.listen(3000, () => {
console.log('Listening on port 3000...')
})
parser(req, callback)
The parser()
function is a top-level function exported by the form-parser
module.
req
HTTP request object.callback(field => {})
An Async function, that's called for each new form field found. Passes field
as argument.field
Is an object containing the following keys:
fieldType
The field type (one of 'text' or 'file').fieldName
The field name.fieldContent
The field content.
fieldType
is 'text', fieldContent
will contain the field text value.fieldType
is 'file', fieldContent
will contain an object with the following keys:
fileName
The name of the file.fileType
The mime type of the file.fileStream
The file stream (ReadableStream).FAQs
A streaming and asynchronous multipart/form-data parser.
The npm package form-parser receives a total of 0 weekly downloads. As such, form-parser popularity was classified as not popular.
We found that form-parser demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.