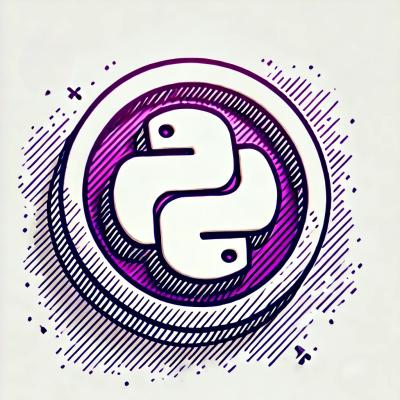
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
formdata-node
Advanced tools
The formdata-node package is a Node.js module that allows you to create, manipulate, and encode multipart/form-data streams. It can be used to submit forms and upload files via HTTP requests in a way that is compatible with browser FormData API.
Creating FormData
This feature allows you to create a new FormData instance and append fields to it, similar to how you would in a browser environment.
const { FormData } = require('formdata-node');
const formData = new FormData();
formData.append('key', 'value');
Appending Files
This feature enables appending files to the FormData instance, which can then be used to upload files to a server.
const { FormData, fileFromPath } = require('formdata-node');
const formData = new FormData();
formData.append('file', fileFromPath('./path/to/file.txt'));
Retrieving FormData Content
This feature allows you to iterate over the entries in the FormData object, enabling you to access the keys and values that have been appended.
const { FormData } = require('formdata-node');
const formData = new FormData();
formData.append('key', 'value');
for (const [key, value] of formData) {
console.log(key, value);
}
Encoding FormData
This feature is used to encode the FormData into a Blob, which can then be used to send the data over an HTTP request.
const { FormData, formDataToBlob } = require('formdata-node');
const formData = new FormData();
formData.append('key', 'value');
const blob = formDataToBlob(formData);
The 'form-data' package is similar to 'formdata-node' and is used to create readable 'multipart/form-data' streams. It can be used in the Node.js environment and is compatible with 'request' library. It differs in API and internal implementation but serves a similar purpose.
Busboy is a Node.js module for parsing incoming HTML form data. It's different from 'formdata-node' as it focuses on parsing form data rather than creating it, but it can handle multipart/form-data, which is commonly used for file uploads.
Multiparty is a Node.js module for parsing multipart/form-data requests, which is used for uploading files. Unlike 'formdata-node', which is for creating and encoding form data, 'multiparty' is designed for the server-side to parse incoming data.
Spec-compliant FormData
implementation for Node.js
FormData interface
.fileFromPath
and fileFromPathSync
helpers to create a File from FS, or you can implement your BlobDataItem
object to use a different source of data.ESM/CJS support
section for details.formdata-polyfill
ponyfill
! Which means, no effect has been caused on globalThis
or native FormData
implementation.You can install this package with npm:
npm install formdata-node
Or yarn:
yarn add formdata-node
Or pnpm
pnpm add formdata-node
This package is targeting ESM and CJS for backwards compatibility reasons and smoothen transition period while you convert your projects to ESM only. Note that CJS support will be removed as Node.js v12 will reach its EOL. This change will be released as major version update, so you won't miss it.
import {FormData} from "formdata-node"
// I assume Got >= 12.x is used for this example
import got from "got"
const form = new FormData()
form.set("greeting", "Hello, World!")
const data = await got.post("https://httpbin.org/post", {body: form}).json()
console.log(data.form.greeting) // => Hello, World!
form-data-encoder
to encode entries:import {Readable} from "stream"
import {FormDataEncoder} from "form-data-encoder"
import {FormData} from "formdata-node"
// Note that `node-fetch` >= 3.x have builtin support for spec-compliant FormData, sou you'll only need the `form-data-encoder` if you use `node-fetch` <= 2.x.
import fetch from "node-fetch"
const form = new FormData()
form.set("field", "Some value")
const encoder = new FormDataEncoder(form)
const options = {
method: "post",
headers: encoder.headers,
body: Readable.from(encoder)
}
await fetch("https://httpbin.org/post", options)
import {FormData, File} from "formdata-node" // You can use `File` from fetch-blob >= 3.x
import fetch from "node-fetch"
const form = new FormData()
const file = new File(["My hovercraft is full of eels"], "file.txt")
form.set("file", file)
await fetch("https://httpbin.org/post", {method: "post", body: form})
import {FormData, Blob} from "formdata-node" // You can use `Blob` from fetch-blob
const form = new FormData()
const blob = new Blob(["Some content"], {type: "text/plain"})
form.set("blob", blob)
// Will always be returned as `File`
let file = form.get("blob")
// The created file has "blob" as the name by default
console.log(file.name) // -> blob
// To change that, you need to set filename argument manually
form.set("file", blob, "some-file.txt")
file = form.get("file")
console.log(file.name) // -> some-file.txt
fileFromPath
or fileFromPathSync
helpers. It does the same thing as fetch-blob/from
, but returns a File
instead of Blob
:import {fileFromPath} from "formdata-node/file-from-path"
import {FormData} from "formdata-node"
import fetch from "node-fetch"
const form = new FormData()
form.set("file", await fileFromPath("/path/to/a/file"))
await fetch("https://httpbin.org/post", {method: "post", body: form})
import {Readable} from "stream"
import {FormData} from "formdata-node"
class BlobFromStream {
#stream
constructor(stream, size) {
this.#stream = stream
this.size = size
}
stream() {
return this.#stream
}
get [Symbol.toStringTag]() {
return "Blob"
}
}
const content = Buffer.from("Stream content")
const stream = new Readable({
read() {
this.push(content)
this.push(null)
}
})
const form = new FormData()
form.set("stream", new BlobFromStream(stream, content.length), "file.txt")
await fetch("https://httpbin.org/post", {method: "post", body: form})
form-data-encoder
package. This is necessary to control which headers will be sent with your HTTP request:import {Readable} from "stream"
import {Encoder} from "form-data-encoder"
import {FormData} from "formdata-node"
const form = new FormData()
// You can use file-shaped or blob-shaped objects as FormData value instead of creating separate class
form.set("stream", {
type: "text/plain",
name: "file.txt",
[Symbol.toStringTag]: "File",
stream() {
return getStreamFromSomewhere()
}
})
const encoder = new Encoder(form)
const options = {
method: "post",
headers: {
"content-type": encoder.contentType
},
body: Readable.from(encoder)
}
await fetch("https://httpbin.org/post", {method: "post", body: form})
class FormData
constructor([entries]) -> {FormData}
Creates a new FormData instance
set(name, value[, filename]) -> {void}
Set a new value for an existing key inside FormData, or add the new field if it does not already exist.
value
.Blob
or File
. If none of these are specified the value is converted to a string.append(name, value[, filename]) -> {void}
Appends a new value onto an existing key inside a FormData object, or adds the key if it does not already exist.
The difference between set()
and append()
is that if the specified key already exists, set()
will overwrite all existing values with the new one, whereas append()
will append the new value onto the end of the existing set of values.
value
.Blob
or File
. If none of these are specified the value is converted to a string.get(name) -> {FormDataValue}
Returns the first value associated with a given key from within a FormData
object.
If you expect multiple values and want all of them, use the getAll()
method instead.
getAll(name) -> {Array<FormDataValue>}
Returns all the values associated with a given key from within a FormData
object.
has(name) -> {boolean}
Returns a boolean stating whether a FormData
object contains a certain key.
delete(name) -> {void}
Deletes a key and its value(s) from a FormData
object.
forEach(callback[, thisArg]) -> {void}
Executes a given callback for each field of the FormData instance
keys() -> {Generator<string>}
Returns an iterator
allowing to go through all keys contained in this FormData
object.
Each key is a string
.
values() -> {Generator<FormDataValue>}
Returns an iterator
allowing to go through all values contained in this object FormData
object.
Each value is a FormDataValue
.
entries() -> {Generator<[string, FormDataValue]>}
Returns an iterator
allowing to go through key/value pairs contained in this FormData
object.
The key of each pair is a string; the value is a FormDataValue
.
[Symbol.iterator]() -> {Generator<[string, FormDataValue]>}
An alias for FormData#entries()
class Blob
The Blob
object represents a blob, which is a file-like object of immutable, raw data;
they can be read as text or binary data, or converted into a ReadableStream
so its methods can be used for processing the data.
constructor(blobParts[, options]) -> {Blob}
Creates a new Blob
instance. The Blob
constructor accepts following arguments:
Array
strings, or ArrayBuffer
, ArrayBufferView
, Blob
objects, or a mix of any of such objects, that will be put inside the Blob
;MIME
) of the blob represented by a Blob
object.type -> {string}
Returns the MIME type
of the Blob
or File
.
size -> {number}
Returns the size of the Blob
or File
in bytes.
slice([start, end, contentType]) -> {Blob}
Creates and returns a new Blob
object which contains data from a subset of the blob on which it's called.
{number} [start = 0] An index into the Blob
indicating the first byte to include in the new Blob
. If you specify a negative value, it's treated as an offset from the end of the Blob
toward the beginning. For example, -10 would be the 10th from last byte in the Blob
. The default value is 0. If you specify a value for start that is larger than the size of the source Blob
, the returned Blob
has size 0 and contains no data.
{number} [end = blob
.size] An index into the Blob
indicating the first byte that will not be included in the new Blob
(i.e. the byte exactly at this index is not included). If you specify a negative value, it's treated as an offset from the end of the Blob
toward the beginning. For example, -10 would be the 10th from last byte in the Blob
. The default value is size.
{string} [contentType = ""] The content type to assign to the new Blob
; this will be the value of its type property. The default value is an empty string.
stream() -> {ReadableStream<Uint8Array>}
Returns a ReadableStream
which upon reading returns the data contained within the Blob
.
arrayBuffer() -> {Promise<ArrayBuffer>}
Returns a Promise
that resolves with the contents of the blob as binary data contained in an ArrayBuffer
.
text() -> {Promise<string>}
Returns a Promise
that resolves with a string containing the contents of the blob, interpreted as UTF-8.
class File extends Blob
The File
class provides information about files. The File
class inherits Blob
.
constructor(fileBits, filename[, options]) -> {File}
Creates a new File
instance. The File
constructor accepts following arguments:
Array
strings, or ArrayBuffer
, ArrayBufferView
, Blob
objects, or a mix of any of such objects, that will be put inside the File
;MIME
) of the file represented by a File
object.fileFromPath(path[, filename, options]) -> {Promise<File>}
Available from formdata-node/file-from-path
subpath.
Creates a File
referencing the one on a disk by given path.
File
constructor. If not presented, the file path will be used to get it.MIME
) of the file represented by a File
object.fileFromPathSync(path[, filename, options]) -> {File}
Available from formdata-node/file-from-path
subpath.
Creates a File
referencing the one on a disk by given path. Synchronous version of the fileFromPath
.
File
constructor. If not presented, the file path will be used to get it.MIME
) of the file represented by a File
object.isFile(value) -> {boolean}
Available from formdata-node/file-from-path
subpath.
Checks if given value is a File, Blob or file-look-a-like object.
FormData
documentation on MDNFile
documentation on MDNBlob
documentation on MDNFormDataValue
documentation on MDN.formdata-polyfill
HTML5 FormData
for Browsers & NodeJS.node-fetch
a light-weight module that brings the Fetch API to Node.jsfetch-blob
a Blob implementation on node.js, originally from node-fetch
.form-data-encoder
spec-compliant multipart/form-data
encoder implementation.then-busboy
a promise-based wrapper around Busboy. Process multipart/form-data content and returns it as a single object. Will be helpful to handle your data on the server-side applications.@octetstream/object-to-form-data
converts JavaScript object to FormData.FAQs
Spec-compliant FormData implementation for Node.js
The npm package formdata-node receives a total of 1,402,943 weekly downloads. As such, formdata-node popularity was classified as popular.
We found that formdata-node demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.