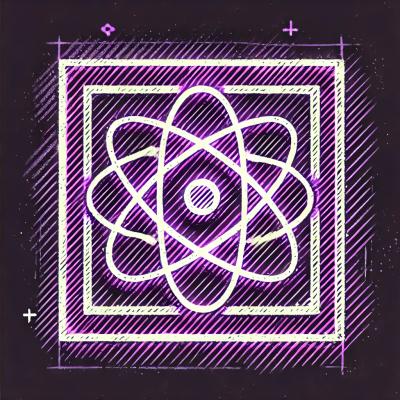
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Promise-based JavaScript FRITZ!Box API.
import FritzBox from 'fritz-box'
const box = new FritzBox({
host: 'fritz.box',
password: '...',
username: '...'
})
const run = async () => {
await box.getSession()
const settings = await box.getGuestWLAN()
settings.active = true
settings.ssid = 'Example!'
await box.setGuestWLAN(settings)
console.log(await box.overview())
}
run()
This will activate the guest WLAN and emit a IFTTT Maker channel notify event. If you create the fitting IFTTT recipe, this snippet should send you the WLAN password right to your smartphone.
import FritzBox from 'fritz-box'
import IFTTT from 'maker-ifttt'
const box = new FritzBox({
host: 'fritz.box',
password: '...',
username: '...'
})
const maker = new IFTTT('IFTTT_MAKER_TOKEN')
const run = async () => {
// generate a random 8-digit hex password
const newPassword = crypto.randomBytes(4).toString('hex');
// sign-in
await box.getSession()
// get current guest WLAN settings
const settings = await box.getGuestWLAN()
// set new password & turn on guest WLAN
settings.key = newPassword
settings.active = true
await box.setGuestWLAN(settings)
// send a message to IFTTT (optional)
maker.triggerEvent('notify', `Guest WLAN password is ${password}.`, response =>
response.on('data', chunk =>
console.info('Response: ' + chunk)
)
)
}
run()
yarn add fritz-box
or
npm i fritz-box
({ host = 'fritz.box', password: String, username: String }: Object): FritzBox
Creates a new FritzBox with the given parameters.
const box = new FritzBox({
host: 'fritz.box',
password: '...',
username: '...'
});
(): Promise
Attempts to log in and fetches a session ID.
;(async () => {
const box = new FritzBox(/* ... */)
await box.getSession()
// fritz-box is now ready for use
})()
(): Promise
Fetches the guest WLAN configuration from the FRITZ!Box.
;(async () => {
const box = new FritzBox(/* ... */)
await box.getSession()
const settings = await box.getGuestWLAN()
})()
(settings: Object): Promise
Applies the (modified) settings
object.
;(async () => {
const box = new FritzBox(/* ... */)
await box.getSession()
await box.setGuestWLAN(settings)
})()
(): Promise
Returns the data contained in the overview tab of the FRITZ!Box user interface.
;(async () => {
const box = new FritzBox(/* ... */)
await box.getSession()
console.log(await box.overview())
})()
(id: String): Promise
Gathers more information about a specific device.
;(async () => {
const box = new FritzBox(/* ... */)
await box.getSession()
console.log(await box.getDeviceDetails('some-id'))
})()
(type = 'all' : String): Promise
Returns log entries. Supported types: 'all'
, 'system'
, 'internet'
, 'wlan'
, 'usb'
.
;(async () => {
const box = new FritzBox(/* ... */)
await box.getSession()
console.log(await box.getLog())
})()
Tested in FRITZ!OS 6.92 on a FRITZ!Box 7590.
FRITZ!Box and FRITZ!OS are registered trademarks of AVM. This project does not grant you any permissions to use them.
1.2.0
getLog
1.1.0
getDeviceDetails
FAQs
FRITZ!Box API
We found that fritz-box demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.