What is froala-editor?
Froala Editor is a lightweight WYSIWYG HTML Editor written in JavaScript that enables rich text editing capabilities for web applications. It is designed to be easy to integrate and offers a wide range of features including text formatting, image and video embedding, and more.
What are froala-editor's main functionalities?
Text Formatting
This feature allows users to format text with options like bold, italic, underline, and more. The code sample initializes the Froala Editor with a toolbar that includes these text formatting options.
const FroalaEditor = require('froala-editor');
new FroalaEditor('#editor', {
toolbarButtons: ['bold', 'italic', 'underline', 'strikeThrough', 'subscript', 'superscript']
});
Image Upload
This feature enables users to upload images directly into the editor. The code sample shows how to configure the editor to upload images to a specified URL.
const FroalaEditor = require('froala-editor');
new FroalaEditor('#editor', {
imageUploadURL: '/upload_image'
});
Video Embedding
This feature allows users to embed videos within the editor. The code sample demonstrates how to enable video embedding in the Froala Editor.
const FroalaEditor = require('froala-editor');
new FroalaEditor('#editor', {
videoUpload: true
});
Custom Toolbar
This feature allows customization of the toolbar to include only the desired buttons. The code sample shows how to initialize the editor with a custom toolbar that includes buttons for text formatting, image insertion, and video insertion.
const FroalaEditor = require('froala-editor');
new FroalaEditor('#editor', {
toolbarButtons: ['bold', 'italic', 'underline', 'insertImage', 'insertVideo']
});
Other packages similar to froala-editor
quill
Quill is a modern WYSIWYG editor built for compatibility and extensibility. It offers a similar range of features to Froala Editor, including text formatting, image and video embedding, and a customizable toolbar. Quill is known for its modular architecture and ease of customization.
tinymce
TinyMCE is a popular rich text editor that provides a wide range of features similar to Froala Editor. It supports text formatting, image and video embedding, and a customizable toolbar. TinyMCE is highly extensible and offers a large number of plugins to enhance its functionality.
ckeditor
CKEditor is a powerful WYSIWYG editor that offers a comprehensive set of features, including text formatting, image and video embedding, and a customizable toolbar. CKEditor is known for its robust performance and extensive plugin ecosystem, making it a strong competitor to Froala Editor.

Froala WYSIWYG HTML Editor is one of the most powerful JavaScript rich text editors ever.
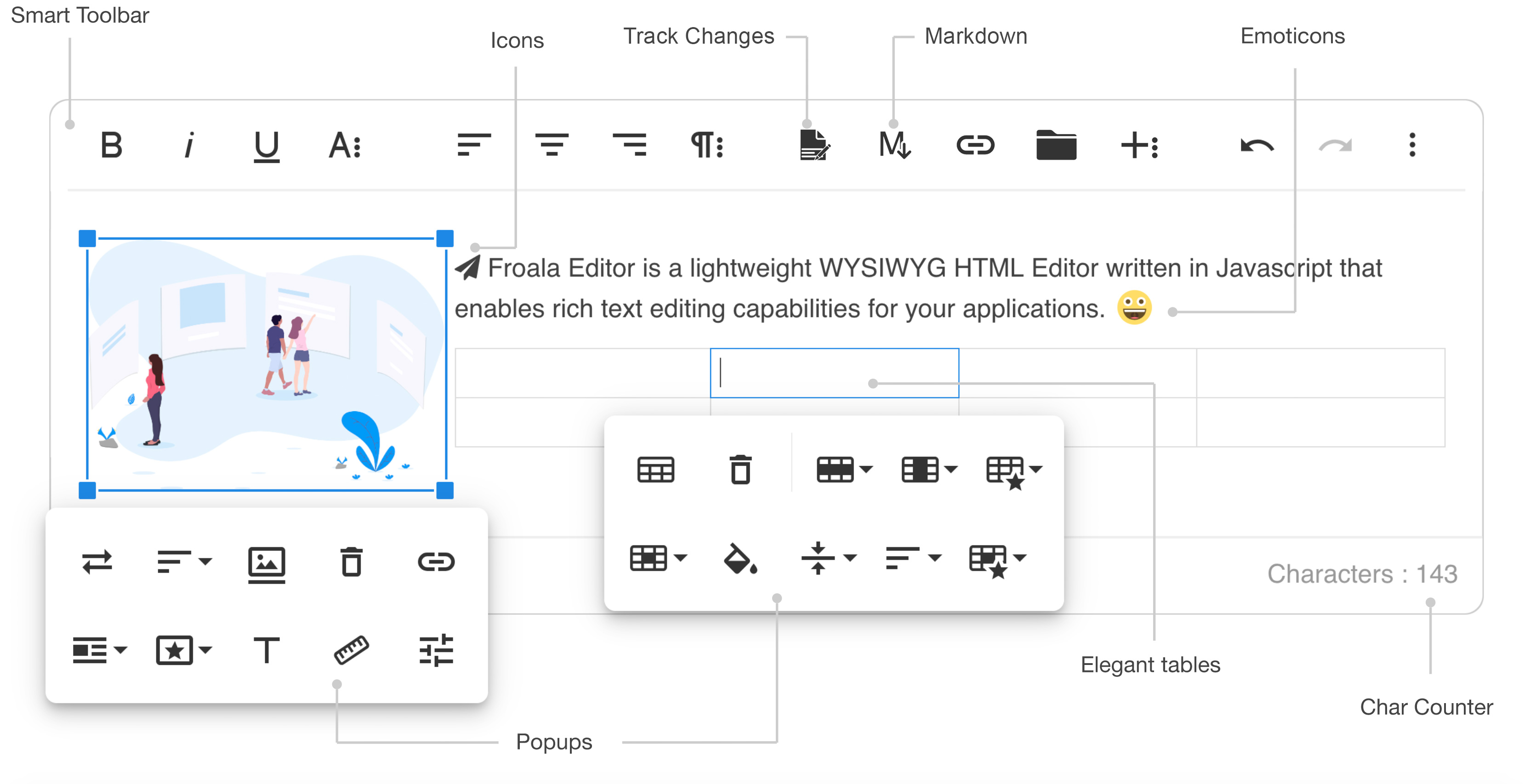
Demos
Download and Install Froala Editor
Install from npm
npm install froala-editor
Install from bower
bower install froala-wysiwyg-editor
Load from CDN
Using Froala Editor from CDN is the easiest way to install it and we recommend using the jsDeliver CDN as it mirrors the NPM package.
<link href="https://cdn.jsdelivr.net/npm/froala-editor@latest/css/froala_editor.pkgd.min.css" rel="stylesheet" type="text/css" />
<textarea></textarea>
<script type="text/javascript" src="https://cdn.jsdelivr.net/npm/froala-editor@latest/js/froala_editor.pkgd.min.js"></script>
<script>
new FroalaEditor('textarea');
</script>
Load from CDN as an AMD module
Froala Editor is compatible with AMD module loaders such as RequireJS. The following example shows how to load it along with the Algin plugin from CDN using RequireJS.
<html>
<head>
<link rel="stylesheet" type="text/css" href="https://cdn.jsdelivr.net/npm/froala-editor@latest/css/froala_editor.css">
<script src="require.js"></script>
<script>
require.config({
packages: [{
name: 'froala-editor',
main: 'js/froala_editor.min'
}],
paths: {
'froala-editor': 'https://cdn.jsdelivr.net/npm/froala-editor@latest'
}
});
</script>
<style>
body {
text-align: center;
}
div#editor {
width: 81%;
margin: auto;
text-align: left;
}
.ss {
background-color: red;
}
</style>
</head>
<body>
<div id="editor">
<div id='edit' style='margin-top:30px;'>
</div>
</div>
<script>
require([
'froala-editor',
'froala-editor/js/plugins/align.min'
], function(FroalaEditor) {
new FroalaEditor('#edit')
});
</script>
</body>
</html>
Load Froala Editor as a CommonJS Module
Froala Editor is using an UMD module pattern, as a result it has support for CommonJS. The following examples presumes you are using npm to install froala-editor, see Download and install FroalaEditor for more details.
var FroalaEditor = require('froala-editor');
require('froala-editor/js/plugins/align.min');
new FroalaEditor('#edit');
Load Froala Editor as a transpiled ES6/UMD module
Since Froala Editor supports ES6 (ESM - ECMAScript modules) and UMD (AMD, CommonJS), it can be also loaded as a module with the use of transpilers. E.g. Babel, Typescript. The following examples presumes you are using npm to install froala-editor, see Download and install FroalaEditor for more details.
import FroalaEditor from 'froala-editor'
import 'froala-editor/js/plugins/align.min.js'
new FroalaEditor('#edit')
For more details on customizing the editor, please check the editor documentation.
Use with your existing framework
Browser Support
At present, we officially aim to support the last two versions of the following browsers:
- Chrome
- Edge
- Firefox
- Safari
- Opera
- Internet Explorer 11
- Safari iOS
- Chrome, Firefox and Default Browser Android
Resources
Reporting Issues
We use GitHub Issues as the official bug tracker for the Froala WYSIWYG HTML Editor. Here are some advices for our users that want to report an issue:
- Make sure that you are using the latest version of the Froala WYSIWYG Editor. The issue that you are about to report may be already fixed in the latest master branch version: https://github.com/froala/froala-wysiwyg/tree/master/js.
- Providing us reproducible steps for the issue will shorten the time it takes for it to be fixed. A JSFiddle is always welcomed, and you can start from this basic one.
- Some issues may be browser specific, so specifying in what browser you encountered the issue might help.
Technical Support or Questions
If you have questions or need help integrating the editor please contact us instead of opening an issue.
Licensing
In order to use the Froala Editor you have to purchase one of the following licenses according to your needs. You can find more about that on our website on the pricing plan page.