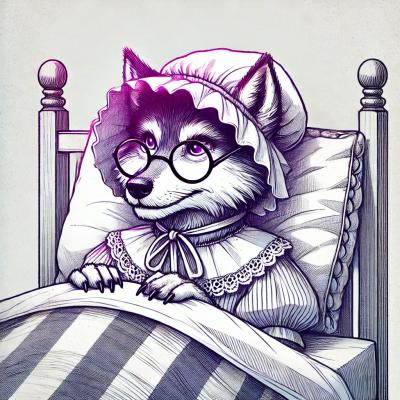
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
fs-tree-diff
Advanced tools
The fs-tree-diff npm package is used to efficiently compare and manage differences between two file system trees. It is particularly useful for tasks that involve synchronizing directories, detecting changes, and applying updates based on those changes.
Compare two directories
This feature allows you to compare two directories and generate a patch that represents the differences between them. The code sample demonstrates how to create two file system trees and calculate the patch.
const FSTree = require('fs-tree-diff');
const entriesA = FSTree.fromEntries([{ relativePath: 'file1.txt', mode: 0o100644, size: 123, mtime: new Date() }]);
const entriesB = FSTree.fromEntries([{ relativePath: 'file2.txt', mode: 0o100644, size: 456, mtime: new Date() }]);
const treeA = new FSTree({ entries: entriesA });
const treeB = new FSTree({ entries: entriesB });
const patch = treeA.calculatePatch(treeB);
console.log(patch);
Apply a patch to a directory
This feature allows you to apply a patch to a directory, effectively synchronizing it with another directory. The code sample demonstrates how to calculate a patch and then apply it to a file system tree.
const FSTree = require('fs-tree-diff');
const entriesA = FSTree.fromEntries([{ relativePath: 'file1.txt', mode: 0o100644, size: 123, mtime: new Date() }]);
const entriesB = FSTree.fromEntries([{ relativePath: 'file2.txt', mode: 0o100644, size: 456, mtime: new Date() }]);
const treeA = new FSTree({ entries: entriesA });
const treeB = new FSTree({ entries: entriesB });
const patch = treeA.calculatePatch(treeB);
FSTree.applyPatch(treeA, patch);
console.log(treeA.entries);
Generate entries from a directory
This feature allows you to generate entries from a directory, which can then be used to create a file system tree. The code sample demonstrates how to read a directory and generate entries for each file.
const FSTree = require('fs-tree-diff');
const fs = require('fs');
const path = require('path');
function generateEntries(dir) {
const entries = [];
fs.readdirSync(dir).forEach(file => {
const filePath = path.join(dir, file);
const stats = fs.statSync(filePath);
entries.push({ relativePath: file, mode: stats.mode, size: stats.size, mtime: stats.mtime });
});
return entries;
}
const entries = generateEntries('./my-directory');
console.log(entries);
The 'diff' package provides a way to compare text differences between two strings or files. While it focuses on text comparison, fs-tree-diff is more specialized in comparing file system trees and managing directory synchronization.
The 'rsync' package is a utility for efficiently transferring and synchronizing files across computer systems. It is more comprehensive and includes network transfer capabilities, whereas fs-tree-diff is focused on local file system tree comparisons.
The 'chokidar' package is a file watcher that tracks changes in the file system and triggers events. While it can detect changes, it does not provide the same level of detailed comparison and patching capabilities as fs-tree-diff.
FSTree provides the means to calculate a patch (set of operations) between one file system tree and another.
The possible operations are:
unlink
– remove the specified filermdir
– remove the specified foldermkdir
– create the specified foldercreate
– create the specified fileupdate
– update the specified fileThe operations choosen aim to minimize the amount of IO required to apply a given patch.
For example, a naive rm -rf
of a directory tree is actually quite costly, as child directories
must be recursively traversed, entries stated.. etc, all to figure out what first must be deleted.
Since we patch from tree to tree, discovering new files is both wasteful and un-needed.
The operations will also be provided in the correct order. So when deleting a large tree, unlink and rmdir operations will be provided depthFirst. Allowing us to safely replay the operations without having to first confirm the FS is as we expected.
A simple example:
var FSTree = require('fs-tree-diff');
var current = FSTree.fromPaths([
'a.js'
]);
var next = FSTree.fromPaths({
'b.js'
});
current.calculatePatch(next) === [
['unlink', 'a.js'],
['create', 'b.js']
];
A slightly more complicated example:
var FSTree = require('fs-tree-diff');
var current = FSTree.fromPaths([
'a.js',
'b/f.js'
]);
var next = FSTree.fromPaths({
'b.js',
'b/c/d.js'
'b/e.js'
});
current.calculatePatch(next) === [
['unlink', 'a.js'],
['unlink', 'b/f.js'],
['create', 'b.js'],
['mkdir', 'b/c'],
['create', 'b/c/d.js'],
['create', 'b/e.js']
];
Now, the above examples do not demonstrate update
operations. This is because when providing only paths, we do not have sufficient information to check if one entry is merely different from another with the same relativePath.
For this, FSTree supports more complex input structure. To demonstrate, We will use the walk-sync module. Which provides higher fidelity input, allowing FSTree to also detect changes. More on what an entry from walkSync.entries is
var walkSync = require('walk-sync');
// path/to/root/foo.js
// path/to/root/bar.js
var current = new FSTree({
entries: walkSync.entries('path/to/root')
});
writeFileSync('path/to/root/foo.js', 'new content');
writeFileSync('path/to/root/baz.js', 'new file');
var next = new FSTree({
entries: walkSync.entries('path/to/root')
});
current.calculatePatch(next) === [
['update', 'foo.js'], // mtime + size changed, so this input is stale and needs updating.
['create', 'baz.js'] // new file, so we should create it
/* bar stays the same and is left inert*/
];
FAQs
Backs out file tree changes
The npm package fs-tree-diff receives a total of 676,008 weekly downloads. As such, fs-tree-diff popularity was classified as popular.
We found that fs-tree-diff demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.