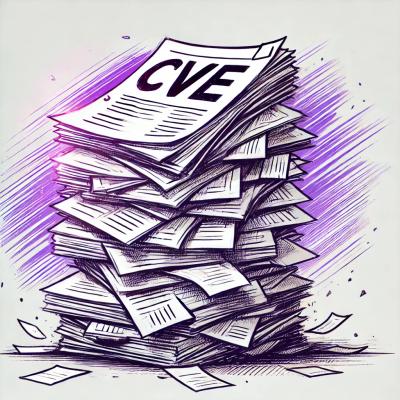
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Fuming is a lightweight and efficient database management system built with TypeScript. It simplifies database operations with type safety, robust query handling, and seamless integration into any modern web application. Fuming is designed as an npm packa
Fuming is a lightweight and efficient database management system built with TypeScript. It simplifies database operations with type safety, robust query handling, and seamless integration into any modern web application.
npm install fuming
import { connect, collection } from 'fuming';
// Connect to database
const db = await connect('mydb');
// Create a collection
collection.create('users');
// Insert data
await collection.insert('users', {
name: 'John Doe',
email: 'john@example.com',
age: 30
});
// Find all documents
const users = collection.find('users');
console.log(users);
connect(dbname: string): Promise<string[]>
Connects to a database. Creates the database directory if it doesn't exist.
const collections = await connect('mydb');
// Returns array of existing collection names
collection.create(collection: string)
Creates a new collection.
collection.create('users');
collection.find(collection: string)
Retrieves all documents from a collection.
const users = collection.find('users');
collection.insert(collection: string, newData: any)
Inserts a new document into a collection. Automatically generates a unique _id
.
await collection.insert('users', {
name: 'Jane Doe',
email: 'jane@example.com'
});
collection.update(collection: string, { key, value }, newData)
Updates a document in a collection based on a key-value pair.
await collection.update('users',
{ key: 'email', value: 'john@example.com' },
{
name: 'John Smith',
email: 'john@example.com'
}
);
collection.remove(collection: string, { key, value })
Removes a document from a collection based on a key-value pair.
await collection.remove('users', {
key: 'email',
value: 'john@example.com'
});
collection.destroy(collection: string)
Deletes an entire collection.
await collection.destroy('users');
Each document in a collection automatically receives a unique _id
field when inserted:
{
"_id": "lqr1k3j4x9ab2m5n",
"name": "John Doe",
"email": "john@example.com"
}
import { connect, collection } from 'fuming';
async function initializeDatabase() {
// Connect to database
await connect('userdb');
// Create users collection
collection.create('users');
}
async function addUser(userData: any) {
return await collection.insert('users', userData);
}
async function updateUserEmail(oldEmail: string, newData: any) {
return await collection.update('users',
{ key: 'email', value: oldEmail },
newData
);
}
async function deleteUser(email: string) {
return await collection.remove('users', {
key: 'email',
value: email
});
}
// Usage
initializeDatabase()
.then(() => addUser({
name: 'John Doe',
email: 'john@example.com',
role: 'admin'
}))
.catch(console.error);
Contributions are welcome! Please feel free to submit a Pull Request. By contributing to this project, you agree to abide by its terms.
This project is licensed under the GNU General Public License v3.0 - see the LICENSE file for details.
Fuming is free software: you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation, either version 3 of the License, or (at your option) any later version.
This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details.
You should have received a copy of the GNU General Public License along with this program. If not, see https://www.gnu.org/licenses/.
FAQs
Fuming is a lightweight and efficient database management system built with TypeScript. It simplifies database operations with type safety, robust query handling, and seamless integration into any modern web application. Fuming is designed as an npm packa
We found that fuming demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.