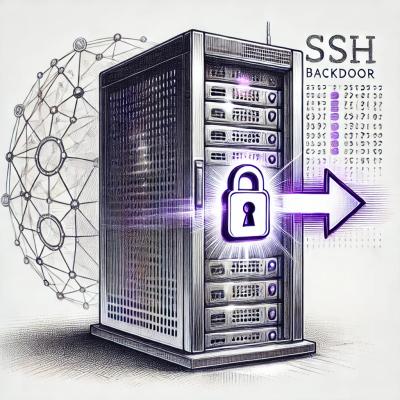
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Hooks for functions.
If you are a fan of React hooks and want to use them everywhere, this is the package for you.
This project is an exploration for what if we could use hooks in functions. It is a proof of concept and not meant to be used in production. The motivation for this is coming from the opposite side of stop using too many hooks inside React components.
useFun
- a hook that allows you to use hooks in functions
useState
- a hook that allows you to create contextual state in functions
useEffect
- a hook that allows you to use effects in functions (effects run on the microtask queue)
useMemo
- a hook that allows you to use memoization in functions
useRef
- a hook that allows you to create refs in functions
useContext
- a hook that allows you to use context value in functions
useCallback
- helper hook that allows you to use memoize functions
createContext
- a function that allows you to create a context
mount
- a function that allows you to mount a scope for a function
autoMount
- same as mount
but waits for all suspended functions to resolve
The basic idea is that you can mount a "scope" on a key, which can be any valid WeakMap key, and then use hooks in that scope. for suspend to work you need to pass a fallback value to useFun
and then use autoMount
to wait for all suspended functions to resolve. This is not the ideal solution and it's not the final API, but it's a start.
Mount a function into a key and use hooks in it.
import { useState, useEffect, mount } from "funooks";
function Demo(number) {
const [state, setState] = useState(0);
console.log(state);
return setState;
}
const key = {};
const setState1 = mount(Demo, [1], key); // not passing a key will use a global Symbol
const setState2 = mount(Demo, [1], key);
assertEquals(setState1, setState2); // true
With different keys, the scopes are not the same
const key1 = {};
const key2 = {};
const setState1 = mount(Demo, [1], key1);
const setState2 = mount(Demo, [1], key2);
assertEquals(setState1, setState2); // false
useFun
and useRef
import { useState, useMemo, useEffect, useRef, mount } from "funooks";
function Component(number) {
return useMemo(() => thisIsExpensive(number), [number]);
}
function Demo() {
const [count1, setCount1] = useState(10);
const [count2, setCount2] = useState(20);
const value1 = useFun(Component, [count1], useRef());
const value2 = useFun(Component, [count2], useRef());
console.log(value1, value2);
return {
setCount1,
setCount2,
};
}
const { setCount1, setCount2 } = mount(Demo, []);
useReducer
- soon to be implementeduseImperativeHandle
- not sure if it's neededNo, you don't. This package is not tied to React in any way. It just uses the same React's hooks API.
No, it's not. It's a serious package that allows you to use hooks in functions.
No, you shouldn't. This is a joke package.
Try to create interesting cases that will justify the existence of this package. If you have any ideas, please open an issue.
FAQs
hooks for functions
We found that funooks demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.