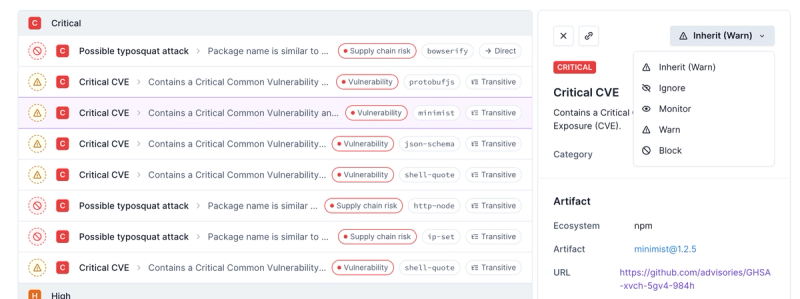
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
fuse.js
Advanced tools
Package description
Fuse.js is a powerful, lightweight fuzzy-search library, with zero dependencies. It provides a simple way to perform approximate string matching (fuzzy searching) by searching for patterns in text, which is useful for implementing features like search-as-you-type, autocomplete, and other search-related functionalities.
Simple Search
This feature allows you to perform a simple fuzzy search on a list of items. The code sample demonstrates searching for the term 'old man' in an array of book titles.
const Fuse = require('fuse.js');
const books = [{ title: 'Old Man's War' }, { title: 'The Lock Artist' }];
const fuse = new Fuse(books, { keys: ['title'] });
const result = fuse.search('old man');
Weighted Search
This feature allows you to perform a search where each field can have a different weight, affecting the relevance of search results. The code sample demonstrates a weighted search where the author field has a higher weight than the title.
const Fuse = require('fuse.js');
const books = [{ title: 'Old Man's War', author: 'John Scalzi' }, { title: 'The Lock Artist', author: 'Steve Hamilton' }];
const fuse = new Fuse(books, { keys: [{ name: 'title', weight: 0.3 }, { name: 'author', weight: 0.7 }] });
const result = fuse.search('john');
Extended Search
This feature allows you to use an extended search syntax to perform searches that include logical operators. The code sample demonstrates searching for items that do not include the word 'lock'.
const Fuse = require('fuse.js');
const books = [{ title: 'Old Man's War' }, { title: 'The Lock Artist' }];
const options = { includeMatches: true, useExtendedSearch: true };
const fuse = new Fuse(books, options);
const result = fuse.search('!lock');
Algolia is a hosted search engine capable of delivering real-time results from the first keystroke. It's more feature-rich and includes a complete search API compared to the client-side library Fuse.js. However, it requires setting up an account and is not a purely client-side solution.
Lunr.js is a small, full-text search library for use in the browser. It indexes data ahead of time and provides a simple search interface. Lunr.js is more suited for smaller datasets and static websites, whereas Fuse.js does not require pre-indexing and can handle dynamic content better.
Elasticlunr.js is a lightweight full-text search engine in JavaScript for browser search and offline search. It is based on lunr.js but provides more flexibility and is faster. Compared to Fuse.js, Elasticlunr.js requires pre-indexing and is more suitable for static content.
Changelog
7.0.0 (2023-10-24)
Readme
Through contributions, donations, and sponsorship, you allow Fuse.js to thrive. Also, you will be recognized as a beacon of support to open-source developers.
|
![]() |
Fuse.js is a lightweight fuzzy-search, in JavaScript, with zero dependencies.
Fuse.js supports all browsers that are ES5-compliant (IE8 and below are not supported).
To check out a live demo and docs, visit fusejs.io.
Here's a separate document for developers.
We've set up a separate document for our contribution guidelines.
FAQs
Lightweight fuzzy-search
The npm package fuse.js receives a total of 2,532,345 weekly downloads. As such, fuse.js popularity was classified as popular.
We found that fuse.js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.