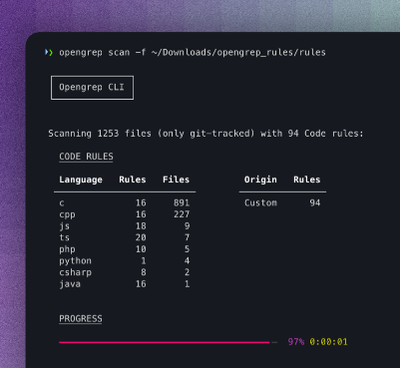
Security News
Opengrep Emerges as Open Source Alternative Amid Semgrep Licensing Controversy
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
The fuzzy npm package is used for implementing fuzzy searching. This means it can match partial strings to a list of strings and return the best matches. It is useful for features like autocomplete or filtering a list based on user input.
Simple fuzzy matching
This feature allows you to perform a simple fuzzy search on an array of strings. It will return an array of matches with their original strings and score of the match.
const fuzzy = require('fuzzy');
const results = fuzzy.filter('twl', ['apple', 'banana', 'kiwi', 'tower']);
console.log(results.map(el => el.string));
Customizing the search
This feature allows you to customize the search with options such as pre and post to highlight the matched substring and extract to specify which property of the objects to search against.
const fuzzy = require('fuzzy');
const options = { pre: '<', post: '>', extract: el => el.title };
const results = fuzzy.filter('twl', [{title: 'apple'}, {title: 'banana'}, {title: 'tower'}], options);
console.log(results.map(el => el.string));
Fuse.js is a powerful, lightweight fuzzy-search library with a rich set of options. It is more feature-rich and configurable than fuzzy, providing options like tokenization, match all tokens, threshold, and distance.
Fuzzysort is a fast SublimeText-like fuzzy search for JavaScript. It focuses on performance and offers features like caching and pre-scoring. It is a good alternative to fuzzy when performance is a critical factor.
Fuzzysearch is a tiny and blazing-fast fuzzy search function that is easy to use and has no dependencies. It is less feature-rich compared to fuzzy but is suitable for simple use cases where size and speed are paramount.
1k standalone fuzzy search / fuzzy filter a la Sublime Text's command-p fuzzy file search. Works in both node and browser.
Try it yourself: Disney Character Search Example
Node:
$ npm install --save fuzzy
$ node
> var fuzzy = require('fuzzy');
> console.log(fuzzy)
{ test: [Function],
match: [Function],
filter: [Function] }
Browser:
<script src="/path/to/fuzzy.js"></script>
<script>
console.log(fuzzy);
// Object >
// filter: function (pattern, arr, opts) {
// match: function (pattern, string, opts) {
// test: function (pattern, string) {
</script>
Padawan: Simply filter an array of strings.
var list = ['baconing', 'narwhal', 'a mighty bear canoe'];
var results = fuzzy.filter('bcn', list)
var matches = results.map(function(el) { return el.string; });
console.log(matches);
// [ 'baconing', 'a mighty bear canoe' ]
Jedi: Wrap matching characters in each string
var list = ['baconing', 'narwhal', 'a mighty bear canoe'];
var options = { pre: '<', post: '>' };
var results = fuzzy.filter('bcn', list, options)
console.log(results);
// [
// {string: '<b>a<c>o<n>ing' , index: 0, score: 3, original: 'baconing'},
// {string: 'a mighty <b>ear <c>a<n>oe', index: 2, score: 3, original: 'a mighty bear canoe'}
// ]
Jedi Master: sometimes the array you give is not an array of strings. You can pass in a function that creates the string to match against from each element in the given array
var list = [
{rompalu: 'baconing', zibbity: 'simba'}
, {rompalu: 'narwhal' , zibbity: 'mufasa'}
, {rompalu: 'a mighty bear canoe', zibbity: 'saddam hussein'}
];
var options = {
pre: '<'
, post: '>'
, extract: function(el) { return el.rompalu; }
};
var results = fuzzy.filter('bcn', list, options);
var matches = results.map(function(el) { return el.string; });
console.log(matches);
// [ '<b>a<c>o<n>ing', 'a mighty <b>ear <c>a<n>oe' ]
Check out the html files in the examples directory.
Try the examples live:
Code is well documented and the unit tests cover all functionality
Fork the repo!
git clone <your_fork>
cd fuzzy
npm install
make
Add unit tests for any new or changed functionality. Lint, test, and minify using make, then shoot me a pull request.
v0.1.0 - July 25, 2012
v0.1.1 - September 19, 2015
v0.1.2 - September 25, 2016
v0.1.3 - October 1, 2016
Copyright (c) 2015 Matt York Licensed under the MIT license.
<b>od<a>ciou<s> ba<s>s
. There is
a test already written, just need to implement it. Naive O(n^2) worst
case: find every match in the string, then select the highest scoring
match. Should benchmark this against current implementation once implemented
Also, "reactive rice" would be <r><e>active r<i><c>e
FAQs
small, standalone fuzzy search / fuzzy filter. browser or node
The npm package fuzzy receives a total of 1,581,680 weekly downloads. As such, fuzzy popularity was classified as popular.
We found that fuzzy demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.