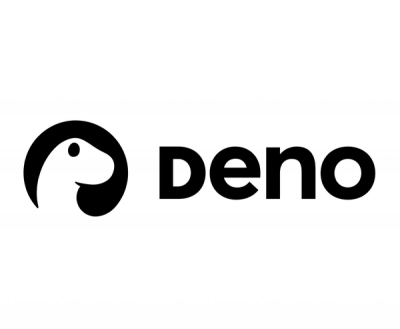
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
gcp-metadata
Advanced tools
The gcp-metadata npm package is a library that allows users to query Google Cloud Platform's (GCP) metadata service for information about the currently running instance and project. This can be useful for applications that need to understand their environment within GCP, such as retrieving instance attributes or project details.
Instance Metadata
Retrieve metadata of the current GCP instance. This includes details like the instance ID, zone, and custom metadata.
const gcpMetadata = require('gcp-metadata');
async function getInstanceMetadata() {
if (await gcpMetadata.isAvailable()) {
const instanceMetadata = await gcpMetadata.instance();
console.log(instanceMetadata);
}
}
getInstanceMetadata();
Project Metadata
Fetch metadata of the current GCP project. This can include project-wide attributes like project ID and numeric project number.
const gcpMetadata = require('gcp-metadata');
async function getProjectMetadata() {
if (await gcpMetadata.isAvailable()) {
const projectMetadata = await gcpMetadata.project();
console.log(projectMetadata);
}
}
getProjectMetadata();
Check Metadata Server Availability
Determine if the GCP metadata server is available and reachable from the instance.
const gcpMetadata = require('gcp-metadata');
async function checkAvailability() {
const isAvailable = await gcpMetadata.isAvailable();
console.log('Metadata service available:', isAvailable);
}
checkAvailability();
The AWS SDK for JavaScript allows developers to interact with AWS services. It includes a module for querying the AWS EC2 instance metadata service, which is similar to what gcp-metadata does for GCP.
Get the metadata from a Google Cloud Platform environment
$ npm install --save gcp-metadata
const gcpMetadata = require('gcp-metadata');
const res = await gcpMetadata.instance();
console.log(res.data); // ... All metadata properties
const res = await gcpMetadata.instance('hostname');
console.log(res.data) // ...All metadata properties
const res = await gcpMetadata.instance({
property: 'tags',
params: { alt: 'text' }
});
console.log(res.data) // ...Tags as newline-delimited list
FAQs
Get the metadata from a Google Cloud Platform environment
The npm package gcp-metadata receives a total of 8,663,206 weekly downloads. As such, gcp-metadata popularity was classified as popular.
We found that gcp-metadata demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.