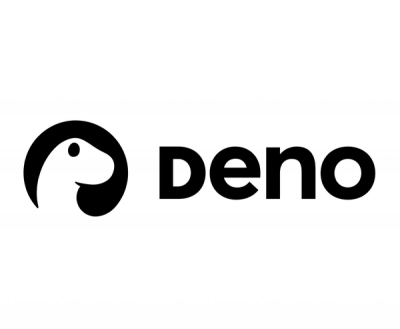
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
generator-spock
Advanced tools
First, install Yeoman and generator-spock using npm (we assume you have pre-installed node.js).
npm install -g yo
npm install -g generator-spock
All generators take these options:
yo spock:action
Actions example output:
/**
* ShoppingCart Actions
*/
// Action Types
// Action Creators
// Async Actions
yo spock:component
Options: class, functional, connected, native, class typescript, functional typescript
Class option example output:
/**
* ShoppingCart
*/
import React from 'react';
export default class ShoppingCart extends React.Component {
render() {
return (
<div className="shopping-cart">
ShoppingCart component
</div>
);
}
}
Functional option example output:
/**
* ShoppingCart
*/
import React from 'react';
const ShoppingCart = () => {
return (
<div className="shopping-cart">
ShoppingCart component
</div>
);
};
export default ShoppingCart;
Connected option example output:
/**
* ShoppingCart
*/
import React from 'react';
import { connect } from 'react-redux';
import ShoppingCart from './ShoppingCart';
const makeMapToStateProps = (state) => ({})
const mapDispatchToProps = (dispath) => ({});
export default connect(makeMapToStateProps, mapDispatchToProps)(ShoppingCart);
Functional Native option example output:
/**
* ShoppingCart
*/
import React from 'react';
import { View } from 'react-native';
const ShoppingCart = () => {
return (
<View>
ShoppingCart component
</View>
);
};
export default ShoppingCart;
Class Typescript option example output:
/**
* ShoppingCart
*/
import * as React from 'react';
interface Props {
}
export default class ShoppingCart extends React.Component<Props, {}> {
render() {
return (
<div className="shopping-cart">
ShoppingCart component
</div>
);
}
}
Functional Typescript option example output:
/**
* ShoppingCart
*/
import * as React from 'react';
interface Props {
}
const ShoppingCart: React.FC = (props: Props) => {
return (
<div className="shopping-cart">
ShoppingCart component
</div>
);
};
export default ShoppingCart;
yo spock:reducer
The reducer generator also supports typescript. The only difference will be the file extension.
Options: page, module
Page option example output:
/**
* ShoppingCart Reducer
*/
import { combineReducers } from 'redux';
export default combineReducers({
});
Module option example output:
/**
* ShoppingCart Reducer
*/
export default (state = {}, action) => {
switch (action.type) {
default:
return state;
}
};
yo spock:selector
The selector generator also supports typescript. The only difference will be the file extension.
Example output:
/**
* ShoppingCart Selectors
*/
import { createSelector } from 'reselect';
const rootSelector = state => state;
export const sampleSelector = createSelector(
[rootSelector],
state => state
);
yo spock:story
Example output
/**
* ShoppingCart Stories
*/
import React from 'react';
import { storiesOf } from '@storybook/react';
const stories = storiesOf('ShoppingCart', module);
stories.add('ShoppingCart', () => (
<div>
ShoppingCart
</div>
));
Example typescript output
/**
* ShoppingCart Stories
*/
import * as React from 'react';
import { storiesOf } from '@storybook/react';
const stories = storiesOf('ShoppingCart', module);
stories.add('ShoppingCart', () => (
<div>
ShoppingCart
</div>
));
MIT © Rafael Rozon
FAQs
React/Redux Yeoman Generators
The npm package generator-spock receives a total of 1 weekly downloads. As such, generator-spock popularity was classified as not popular.
We found that generator-spock demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.