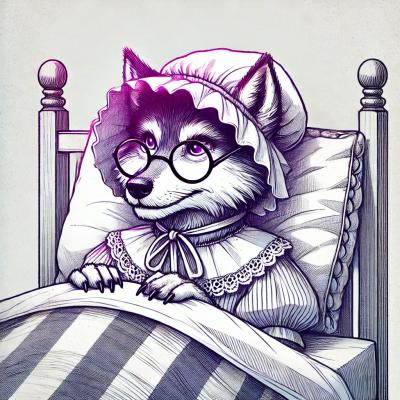
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
geojson-equality
Advanced tools
The geojson-equality npm package is used to compare GeoJSON objects for structural equality. It helps in determining if two GeoJSON objects represent the same geometry, even if their coordinates are ordered differently or if they have different properties.
Compare GeoJSON objects for equality
This feature allows you to compare two GeoJSON objects to check if they are equal. The comparison takes into account the structure and coordinates of the GeoJSON objects.
const GeojsonEquality = require('geojson-equality');
const equality = new GeojsonEquality();
const geojson1 = { "type": "Point", "coordinates": [100.0, 0.0] };
const geojson2 = { "type": "Point", "coordinates": [100.0, 0.0] };
console.log(equality.compare(geojson1, geojson2)); // true
Compare GeoJSON objects with tolerance
This feature allows you to compare two GeoJSON objects with a specified precision tolerance. This is useful when dealing with floating-point inaccuracies.
const GeojsonEquality = require('geojson-equality');
const equality = new GeojsonEquality({ precision: 6 });
const geojson1 = { "type": "Point", "coordinates": [100.000001, 0.000001] };
const geojson2 = { "type": "Point", "coordinates": [100.0, 0.0] };
console.log(equality.compare(geojson1, geojson2)); // true
Compare GeoJSON objects ignoring properties
This feature allows you to compare two GeoJSON objects while ignoring their properties. This is useful when you only care about the geometry and not the associated properties.
const GeojsonEquality = require('geojson-equality');
const equality = new GeojsonEquality({ ignoreProperties: true });
const geojson1 = { "type": "Point", "coordinates": [100.0, 0.0], "properties": { "name": "A" } };
const geojson2 = { "type": "Point", "coordinates": [100.0, 0.0], "properties": { "name": "B" } };
console.log(equality.compare(geojson1, geojson2)); // true
Turf is a powerful geospatial analysis library that includes a wide range of functions for processing GeoJSON data. It offers more comprehensive geospatial analysis capabilities compared to geojson-equality, including measurement, transformation, and data manipulation functions.
Deep-equal is a general-purpose deep comparison library that can be used to compare any JavaScript objects, including GeoJSON objects. While it does not offer GeoJSON-specific features like geojson-equality, it is versatile and can be used for a wide range of deep comparison tasks.
Geojson-utils is a library that provides utility functions for working with GeoJSON data. It includes functions for distance calculation, point-in-polygon tests, and bounding box calculations. While it does not focus on equality comparison, it offers a variety of tools for GeoJSON data manipulation.
Check two valid geojson geometries for equality.
npm install geojson-equality
var GeojsonEquality = require('geojson-equality');
var eq = new GeojsonEquality();
var g1 = { "type": "Polygon", "coordinates": [
[[30, 10], [40, 40], [20, 40], [10, 20], [30, 10]]
]};
var g2 = { "type": "Polygon", "coordinates": [
[[30, 10], [40, 40], [20, 40], [10, 20], [30, 10]]
]};
eq.compare(g1,g2); // returns true
var g3 = { "type": "Polygon", "coordinates": [
[[30o, 100], [400, 400], [200, 400], [100, 200], [300, 100]]
]};
eq.compare(g1,g3); // returns false
For including in browser, download file geojson-equality.min.js
<script type="text/javascipt" src="path/to/geojson-equality.min.js"></script>
This create a global variable 'GeojsonEquality' GeojsonEquality class can be initiated with many options that are used to match the geojson.
var g1 = { "type": "Point", "coordinates": [30.2, 10] };
var g2 = { "type": "Point", "coordinates": [30.22233, 10] };
var eq = new GeojsonEqaulity({precision: 3});
eq.compare(g1,g2); // returns false
var eq = new GeojsonEqaulity({precision: 1});
eq.compare(g1,g2); // returns true
var g1 = { "type": "LineString", "coordinates":
[ [30, 10], [10, 30], [40, 40] ] };
var g2 = { "type": "LineString", "coordinates":
[ [40, 40], [10, 30], [30, 10] ] };
var eq = new GeojsonEqaulity({direction: false});
eq.compare(g1,g2); // returns true
var eq = new GeojsonEqaulity({direction: true});
eq.compare(g1,g2); // returns false
// using lodash isEqual to deep comparison
var isEqual = require('lodash/lang/isEqual')
var eq = new GeojsonEqaulity({objectComparator: isEqual});
Once you run
npm install
then for running test
npm run test
to create build
npm run build
This project is licensed under the terms of the MIT license.
FAQs
Check two valid geojson geometries for equality.
The npm package geojson-equality receives a total of 0 weekly downloads. As such, geojson-equality popularity was classified as not popular.
We found that geojson-equality demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.