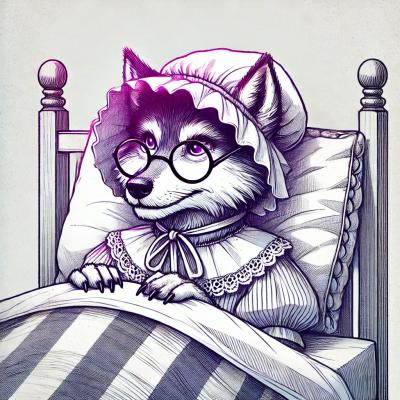
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
geojson-rbush
Advanced tools
The geojson-rbush npm package is a spatial index for GeoJSON objects, built on top of the R-tree data structure. It allows for efficient spatial querying and manipulation of GeoJSON data, such as points, lines, and polygons.
Insert GeoJSON objects
This feature allows you to insert GeoJSON objects into the spatial index. The code sample demonstrates how to create a new GeoJSONRBush instance and insert a GeoJSON FeatureCollection into it.
const GeoJSONRBush = require('geojson-rbush');
const tree = GeoJSONRBush();
const geojson = { "type": "FeatureCollection", "features": [ { "type": "Feature", "geometry": { "type": "Point", "coordinates": [102.0, 0.5] }, "properties": { "prop0": "value0" } } ] };
tree.insert(geojson);
Search for GeoJSON objects
This feature allows you to search for GeoJSON objects within a specified area. The code sample demonstrates how to search for GeoJSON objects within a given polygon.
const searchArea = { "type": "Feature", "geometry": { "type": "Polygon", "coordinates": [ [ [100.0, 0.0], [101.0, 0.0], [101.0, 1.0], [100.0, 1.0], [100.0, 0.0] ] ] } };
const results = tree.search(searchArea);
Remove GeoJSON objects
This feature allows you to remove GeoJSON objects from the spatial index. The code sample demonstrates how to remove a specific GeoJSON object from the index.
const geojsonToRemove = { "type": "Feature", "geometry": { "type": "Point", "coordinates": [102.0, 0.5] }, "properties": { "prop0": "value0" } };
tree.remove(geojsonToRemove);
Bulk load GeoJSON objects
This feature allows you to bulk load multiple GeoJSON objects into the spatial index. The code sample demonstrates how to load a GeoJSON FeatureCollection into the index in one operation.
const bulkGeojson = { "type": "FeatureCollection", "features": [ { "type": "Feature", "geometry": { "type": "Point", "coordinates": [102.0, 0.5] }, "properties": { "prop0": "value0" } }, { "type": "Feature", "geometry": { "type": "Point", "coordinates": [103.0, 1.5] }, "properties": { "prop1": "value1" } } ] };
tree.load(bulkGeojson);
rbush is a high-performance JavaScript library for 2D spatial indexing of points and rectangles. It provides similar functionality to geojson-rbush but does not specifically handle GeoJSON objects. Instead, it works with generic bounding boxes.
Turf is a powerful geospatial analysis library for JavaScript. It provides a wide range of spatial operations and utilities for GeoJSON data, including spatial indexing, but it is more comprehensive and includes many other geospatial functions beyond just indexing.
geokdbush is a geospatial index for points based on the KDBush library. It is optimized for fast nearest-neighbor queries and works with GeoJSON points, but it does not support other GeoJSON geometries like polygons or lines.
GeoJSON implementation of RBush — a high-performance JavaScript R-tree-based 2D spatial index for points and rectangles.
npm
$ npm install --save geojson-rbush
GeoJSON implementation of RBush spatial index.
Parameters
maxEntries
number defines the maximum number of entries in a tree node. 9 (used by default) is a
reasonable choice for most applications. Higher value means faster insertion and slower search, and vice versa. (optional, default 9
)Examples
var rbush = require('geojson-rbush')
var tree = rbush()
Returns RBush GeoJSON RBush
Parameters
feature
Feature<any> insert single GeoJSON FeatureExamples
var polygon = {
"type": "Feature",
"properties": {},
"geometry": {
"type": "Polygon",
"coordinates": [[[-78, 41], [-67, 41], [-67, 48], [-78, 48], [-78, 41]]]
}
}
tree.insert(polygon)
Returns RBush GeoJSON RBush
Parameters
features
(Array<BBox> | FeatureCollection<any>) load entire GeoJSON FeatureCollectionExamples
var polygons = {
"type": "FeatureCollection",
"features": [
{
"type": "Feature",
"properties": {},
"geometry": {
"type": "Polygon",
"coordinates": [[[-78, 41], [-67, 41], [-67, 48], [-78, 48], [-78, 41]]]
}
},
{
"type": "Feature",
"properties": {},
"geometry": {
"type": "Polygon",
"coordinates": [[[-93, 32], [-83, 32], [-83, 39], [-93, 39], [-93, 32]]]
}
}
]
}
tree.load(polygons)
Returns RBush GeoJSON RBush
Parameters
feature
(BBox | Feature<any>) remove single GeoJSON FeatureExamples
var polygon = {
"type": "Feature",
"properties": {},
"geometry": {
"type": "Polygon",
"coordinates": [[[-78, 41], [-67, 41], [-67, 48], [-78, 48], [-78, 41]]]
}
}
tree.remove(polygon)
Returns RBush GeoJSON RBush
Examples
tree.clear()
Returns RBush GeoJSON Rbush
Parameters
geojson
(BBox | FeatureCollection | Feature<any>) search with GeoJSONExamples
var polygon = {
"type": "Feature",
"properties": {},
"geometry": {
"type": "Polygon",
"coordinates": [[[-78, 41], [-67, 41], [-67, 48], [-78, 48], [-78, 41]]]
}
}
tree.search(polygon)
Returns FeatureCollection<any> all features that intersects with the given GeoJSON.
Parameters
geojson
(BBox | FeatureCollection | Feature<any>) collides with GeoJSONExamples
var polygon = {
"type": "Feature",
"properties": {},
"geometry": {
"type": "Polygon",
"coordinates": [[[-78, 41], [-67, 41], [-67, 48], [-78, 48], [-78, 41]]]
}
}
tree.collides(polygon)
Returns boolean true if there are any items intersecting the given GeoJSON, otherwise false.
Examples
tree.all()
//=FeatureCollection
Returns FeatureCollection<any> all the features in RBush
Examples
var exported = tree.toJSON()
//=JSON object
Returns any export data as JSON object
Parameters
json
any import previously exported dataExamples
var exported = {
"children": [
{
"type": "Feature",
"geometry": {
"type": "Point",
"coordinates": [110, 50]
},
"properties": {},
"bbox": [110, 50, 110, 50]
}
],
"height": 1,
"leaf": true,
"minX": 110,
"minY": 50,
"maxX": 110,
"maxY": 50
}
tree.fromJSON(exported)
Returns RBush GeoJSON RBush
FAQs
GeoJSON implementation of RBush
The npm package geojson-rbush receives a total of 118,333 weekly downloads. As such, geojson-rbush popularity was classified as popular.
We found that geojson-rbush demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.