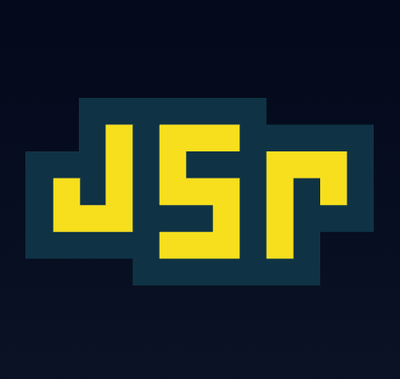
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
git-url-parse
Advanced tools
The git-url-parse npm package is a utility for parsing Git URLs and extracting useful information from them. It supports various Git URL formats and provides methods to manipulate and retrieve different parts of the URL.
Parsing a Git URL
This feature allows you to parse a Git URL and get an object containing various parts of the URL such as the protocol, source, owner, name, and more.
const gitUrlParse = require('git-url-parse');
const parsed = gitUrlParse('https://github.com/IonicaBizau/git-url-parse.git');
console.log(parsed);
Extracting the owner and name
This feature allows you to extract specific parts of the Git URL, such as the owner and repository name.
const gitUrlParse = require('git-url-parse');
const parsed = gitUrlParse('https://github.com/IonicaBizau/git-url-parse.git');
console.log(parsed.owner); // 'IonicaBizau'
console.log(parsed.name); // 'git-url-parse'
Converting to a different URL format
This feature allows you to convert a Git URL from one format to another, such as from HTTPS to SSH.
const gitUrlParse = require('git-url-parse');
const parsed = gitUrlParse('https://github.com/IonicaBizau/git-url-parse.git');
const sshUrl = parsed.toString('ssh');
console.log(sshUrl); // 'git@github.com:IonicaBizau/git-url-parse.git'
The parse-github-url package is a utility for parsing GitHub URLs specifically. It extracts information such as the owner, repository name, and branch. Compared to git-url-parse, it is more focused on GitHub URLs and may not support other Git hosting services.
The git-url package provides similar functionality for parsing and manipulating Git URLs. It offers methods to extract parts of the URL and convert between different URL formats. It is comparable to git-url-parse in terms of functionality but may have different API design and usage patterns.
The url-parse package is a more general-purpose URL parsing library that can handle various types of URLs, not just Git URLs. While it can be used to parse Git URLs, it does not provide Git-specific methods and properties like git-url-parse does.
A high level git url parser for common git providers.
# Using npm
npm install --save git-url-parse
# Using yarn
yarn add git-url-parse
// Dependencies
const GitUrlParse = require("git-url-parse");
console.log(GitUrlParse("git@github.com:IonicaBizau/node-git-url-parse.git"));
// => {
// protocols: []
// , port: null
// , resource: "github.com"
// , user: "git"
// , pathname: "/IonicaBizau/node-git-url-parse.git"
// , hash: ""
// , search: ""
// , href: "git@github.com:IonicaBizau/node-git-url-parse.git"
// , token: ""
// , protocol: "ssh"
// , toString: [Function]
// , source: "github.com"
// , name: "node-git-url-parse"
// , owner: "IonicaBizau"
// }
console.log(GitUrlParse("https://github.com/IonicaBizau/node-git-url-parse.git"));
// => {
// protocols: ["https"]
// , port: null
// , resource: "github.com"
// , user: ""
// , pathname: "/IonicaBizau/node-git-url-parse.git"
// , hash: ""
// , search: ""
// , href: "https://github.com/IonicaBizau/node-git-url-parse.git"
// , token: ""
// , protocol: "https"
// , toString: [Function]
// , source: "github.com"
// , name: "node-git-url-parse"
// , owner: "IonicaBizau"
// }
console.log(GitUrlParse("https://github.com/IonicaBizau/git-url-parse/blob/master/test/index.js"));
// { protocols: [ 'https' ],
// protocol: 'https',
// port: null,
// resource: 'github.com',
// user: '',
// pathname: '/IonicaBizau/git-url-parse/blob/master/test/index.js',
// hash: '',
// search: '',
// href: 'https://github.com/IonicaBizau/git-url-parse/blob/master/test/index.js',
// token: '',
// toString: [Function],
// source: 'github.com',
// name: 'git-url-parse',
// owner: 'IonicaBizau',
// organization: '',
// ref: 'master',
// filepathtype: 'blob',
// filepath: 'test/index.js',
// full_name: 'IonicaBizau/git-url-parse' }
console.log(GitUrlParse("https://github.com/IonicaBizau/node-git-url-parse.git").toString("ssh"));
// => "git@github.com:IonicaBizau/node-git-url-parse.git"
console.log(GitUrlParse("git@github.com:IonicaBizau/node-git-url-parse.git").toString("https"));
// => "https://github.com/IonicaBizau/node-git-url-parse.git"
There are few ways to get help:
gitUrlParse(url)
Parses a Git url.
url
: The Git url to parse.GitUrl
object containing:protocols
(Array): An array with the url protocols (usually it has one element).port
(null|Number): The domain port.resource
(String): The url domain (including subdomains).user
(String): The authentication user (usually for ssh urls).pathname
(String): The url pathname.hash
(String): The url hash.search
(String): The url querystring value.href
(String): The input url.protocol
(String): The git url protocol.token
(String): The oauth token (could appear in the https urls).source
(String): The Git provider (e.g. "github.com"
).owner
(String): The repository owner.name
(String): The repository name.ref
(String): The repository ref (e.g., "master" or "dev").filepath
(String): A filepath relative to the repository root.filepathtype
(String): The type of filepath in the url ("blob" or "tree").full_name
(String): The owner and name values in the owner/name
format.toString
(Function): A function to stringify the parsed url into another url type.organization
(String): The organization the owner belongs to. This is CloudForge specific.git_suffix
(Boolean): Whether to add the .git
suffix or not.stringify(obj, type)
Stringifies a GitUrl
object.
obj
: The parsed Git url object.type
: The type of the stringified url (default obj.protocol
).Have an idea? Found a bug? See how to contribute.
I open-source almost everything I can, and I try to reply to everyone needing help using these projects. Obviously, this takes time. You can integrate and use these projects in your applications for free! You can even change the source code and redistribute (even resell it).
However, if you get some profit from this or just want to encourage me to continue creating stuff, there are few ways you can do it:
Starring and sharing the projects you like :rocket:
—I love books! I will remember you after years if you buy me one. :grin: :book:
—You can make one-time donations via PayPal. I'll probably buy a
coffee tea. :tea:
—Set up a recurring monthly donation and you will get interesting news about what I'm doing (things that I don't share with everyone).
Bitcoin—You can send me bitcoins at this address (or scanning the code below): 1P9BRsmazNQcuyTxEqveUsnf5CERdq35V6
Thanks! :heart:
If you are using this library in one of your projects, add it in this list. :sparkles:
release-it
documentation
apollo
@atomist/automation-client
@storybook/storybook-deployer
@kadira/storybook-deployer
gatsby-source-git
umi-build-dev
@atomist/automation-client-ext-raven
git-source
@atomist/sdm-pack-analysis
@git-stack/server-core
@qiwi/semantic-release-gh-pages-plugin
@nuxt/telemetry
@lerna/github-client
@umijs/block-sdk
@instructure/ui-scripts
lambda-service
@wetrial/block-sdk
hzero-block-sdk
@micro-app/shared-utils
remax-stats
workspace-tools
semantic-release-gitmoji
beachball
gitbook-start-plugin-iaas-ull-es-noejaco2017
autorelease-setup
documentation-custom-markdown
@hygiene/plugin-github-url
sherry-utils
@unibtc/release-it
clipped
@erquhart/lerna-github-client
@0x-lerna-fork/github-client
common-boilerplate
@atomist/uhura
@brisk-docs/website
@hawkingnetwork/react-native-tab-view
miguelcostero-ng2-toasty
docula-ui
@brisk-docs/gatsby-generator
@microservices/cli
@atomist/cli
stylelint-formatter-utils
omg
sync-repos
@facadecompany/ignition-ui
@geut/git-url-parse
@temporg/rds-scripts
@koumoul/gh-pages-multi
@feizheng/next-git-url
@yarnpkg/plugin-git
@git-stack/hemera-plugin
@s-ui/mono
@adminide-stack/git-api-browser
@xdn/cli
@atomist/skill
committing
gitbook-start-https-alex-moi
gitbook-start-iaas-ull-es-merquililycony
proyecto-sytw-alex-moi
gitbook-start-iaas-ull-es-alex-moi
gitbook-start-iaas-bbdd-alex-moi
complan
@axetroy/git-clone
generator-cleanphp
@axetroy/gpmx
documentation-habitlab
nodeschool-admin
one-more-gitlab-cli
def-core
gd-cli
node-coverage-server
strapper
github-publish-npm
ogh
sinit
smart-clone
download-repo-cli
generator-nm-bti
gitc
gitline
gitlab-ci-variables-cli
gitlab-ci-variables-setter-cli
documentation-fork
@axetroy/gpm
generator-openapi-repo
git-url-promise
kef-core
@pvdlg/semantic-release
gitlab-tool-cli
snipx
yarn-upgrade-on-ci
@hjin/generator-app
@reframe/github-pages
belt-repo
@esops/publish-github-pages
@hugomrdias/documentation
just-dev-sdk
@activeviam/documentation
git-signed
cz-conventional-changelog-befe
@voodeng/archive
@voodeng/uppacks
create-apex-js-app
auto-changelog-vsts
harry-reporter
@campus-online/gatsby-source-git
gtni
ci-yarn-upgrade
generator-clearphp
openapi-repo-generator
git-imitate
vscode-gpm
@atomist/ci-sdm
documentation42
@limejs/cli
lime-cli
laborious
release-it-http
bibi
lcov-server
create-release-it
git-service-node
@infinitecsolutions/storybook-deployer
@1nd/documentation
git-cherry-fix
branch-release
vuepress-plugin-remote-url
gatsby-source-github-raw
moto-connector
@zpmpkg/zpm
konitor
release2hub
@geut/git-compare-template
@geut/chan-parser
git-observer
docula-ui-express
sn-flutter-boot
spk
umi-plugin-repo
@epranka/create-tsx-package
flutter-boot
node-norman
air-material_cli
@belt/repo
remote-commit-url
@arcanis/sherlock
@dandean/storybook-deployer
git-upstream
@cratosw/gatsby-antd
@tjmonsi/generator-uplb-hci-lab-project-template
@tagoro9/git
tldw
gatsby-theme-hansin
@temporg/ui-scripts
generate-preview
aral-vps-test
bitbucket-pullr
@s-ui/changelog
@whey/gatsby-theme-whey
@docomodigital/pdor
auto-clone
create-sourcegraph-extension
create-n
aral-server
gatsby-source-git-remotes
@pagedip/tool-autorelease
@shopgate/pwa-releaser
@vicoders/cli2
@stavalfi/ci
@amorist/gatsby-theme-antd
cirodown
@apardellass/react-native-audio-stream
gatsby-theme-cone
changelog.md
wp-continuous-deployment
gatsby-source-npmjs
l2forlerna
@patrickhulce/scripts
@cilyn/bitbucket
react-native-plugpag-wrapper
meta-release
@cyber-tools/lib-cli
@feizheng/git-url-cli
@hnp/package-scripts
git-csv
ship-release
ssh-remote
@senti-techlabs/generator-senti-project-template
canarist
mira
fotingo
react-native-pulsator-native
actions-package-update
@epranka/create-package
@bcgov/gatsby-source-github-raw
kit-command
@theowenyoung/gatsby-source-git
git-lab-cli
git-push-pr
@csmith/release-it
query-registry
@git-stack/module-server
cetus-cli
rollman
cc-flow
@s-ui/ssr
@gasket/plugin-metrics
react-native-kakao-maps
@elestu/actions-dependacop
generate-github-directory
lage
@rescribe/cli
sinanews-flutter-boot
@geeky-apo/react-native-advanced-clipboard
dx-scanner
native-kakao-login
native-google-login
native-apple-login
configorama
@backstage/plugin-catalog-backend
@s-ui/ci
kaskadi-cli
documentation-markdown-themes
@backstage/plugin-scaffolder-backend
@backstage/plugin-techdocs-backend
@pubcore/node-docker-build
@tahini/nc
@antv/gatsby-theme-antv
@myetherwallet/mew-components
@datalogic/react-native-datalogic-module
pr-log
git-issues
FAQs
A high level git url parser for common git providers.
The npm package git-url-parse receives a total of 2,888,848 weekly downloads. As such, git-url-parse popularity was classified as popular.
We found that git-url-parse demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.