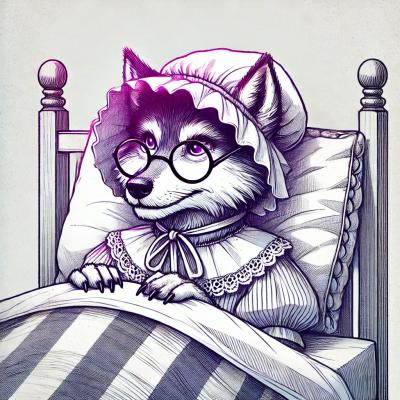
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
gitconfiglocal
Advanced tools
The gitconfiglocal npm package allows you to read and manipulate local Git configuration files. It provides a simple API to access and modify the .git/config file in a Git repository.
Read Local Git Config
This feature allows you to read the local Git configuration of a repository. The code sample demonstrates how to use the gitconfiglocal package to read the .git/config file of a specified repository.
const gitconfiglocal = require('gitconfiglocal');
const path = require('path');
const repoPath = path.resolve('/path/to/repo');
gitconfiglocal(repoPath, (err, config) => {
if (err) throw err;
console.log(config);
});
Modify Local Git Config
This feature allows you to modify the local Git configuration of a repository. The code sample demonstrates how to read the .git/config file, modify the user name and email, and then write the changes back to the file.
const gitconfiglocal = require('gitconfiglocal');
const fs = require('fs');
const ini = require('ini');
const path = require('path');
const repoPath = path.resolve('/path/to/repo');
gitconfiglocal(repoPath, (err, config) => {
if (err) throw err;
config.user.name = 'New User';
config.user.email = 'newuser@example.com';
fs.writeFileSync(path.join(repoPath, '.git', 'config'), ini.stringify(config));
});
The simple-git package provides a simple interface for running Git commands in any Node.js application. It allows you to execute Git commands programmatically and handle the results. Unlike gitconfiglocal, which focuses on reading and modifying the .git/config file, simple-git provides a broader range of Git functionalities.
The nodegit package is a native Node.js binding to the libgit2 library, which provides a comprehensive set of Git functionalities. It allows you to perform various Git operations, such as cloning repositories, creating branches, and committing changes. While gitconfiglocal is specialized in handling Git configuration files, nodegit offers a more extensive set of Git-related features.
The isomorphic-git package is a pure JavaScript implementation of Git that works in both Node.js and browser environments. It provides a wide range of Git functionalities, including reading and writing Git configuration files. Compared to gitconfiglocal, isomorphic-git offers a more versatile solution that can be used in different environments.
parse the .git/config
file into a useful data structure
var gitconfig = require('gitconfiglocal');
gitconfig('./',function(err,config){
console.log(config);
/* prints:
{ core:
{ repositoryformatversion: '0',
filemode: true,
bare: false,
logallrefupdates: true },
remote:
{ origin:
{ url: 'git@github.com:soldair/node-gitconfiglocal.git',
fetch: '+refs/heads/*:refs/remotes/origin/*' } } }
*/
});
gitconfig('./', { gitDir: 'path/to/gitdir' }, cb);
FAQs
parse the .git/config file into a useful data structure
The npm package gitconfiglocal receives a total of 1,437,620 weekly downloads. As such, gitconfiglocal popularity was classified as popular.
We found that gitconfiglocal demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.