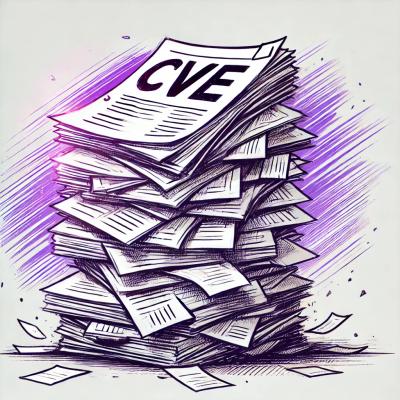
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
globalsprak
Advanced tools
globalsprak is a Node.js package that provides access to various AI models, image generation, content downloading from various platforms, and more.
globalsprak is a Node.js package that provides access to various AI models, image generation, content downloading from various platforms, and more.
Join our discord server
Made by Aryan Chauhan
You can install the package using npm:
npm i globalsprak
All Informations are not available join our discord server for more informations, thank you for using our services 🌷🥰
ai(prompt, model)
prompt
(string): The prompt or question for the AI to generate a response.model
(string): The specific AI model to use for generating responses.const { ai } = require('globalsprak');
async function main() {
const prompt = "What is the capital of France?";
const model = "gpt-4o-mini-free";
const response = await ai(prompt, model);
console.log("AI Response:", response);
}
main();
Get a response from the GPT model based on your prompt.
const { gpt } = require('globalsprak');
async function main() {
const prompt = "What is AI?";
const response = await gpt(prompt);
console.log("GPT Response:", response);
}
main();
Function: chatgpt(prompt)
Description: Retrieves a response from the ChatGPT model based on the provided prompt.
Example:
const { chatgpt } = require('globalsprak');
async function main() {
const prompt = "What's the best way to learn programming?";
const response = await chatgpt(prompt);
console.log("ChatGPT Response:", response);
}
main();
gptweb(prompt)
const { gptweb } = require('globalsprak');
async function main() {
const prompt = "Latest tech trends in 2024";
const response = await gptweb(prompt);
console.log("GPTWeb Response:", response);
}
main();
Get a response from the Llama AI model.
const { llama } = require('globalsprak');
async function main() {
const prompt = "Tell me a joke";
const response = await llama(prompt);
console.log("Llama Response:", response);
}
main();
Get a response from the Mixtral AI model.
const { mixtral } = require('globalsprak');
async function main() {
const prompt = "Explain quantum mechanics";
const response = await mixtral(prompt);
console.log("Mixtral Response:", response);
}
main();
Interact with Coral or reset conversation context.
const { coral } = require('globalsprak');
async function main() {
const prompt = "How's the weather today?";
const response = await coral(prompt);
console.log("Coral Response:", response);
}
main();
const { coralchat } = require('globalsprak');
async function main() {
const prompt = "Tell me a story";
const uid = "user123";
const response = await coralchat(prompt, uid);
console.log("Coral Chat Response:", response);
}
main();
const { coralreset } = require('globalsprak');
async function main() {
const uid = "user123";
const response = await coralreset(uid);
console.log("Coral Reset Response:", response);
}
main();
animagen(prompt, count)
prompt
(string): The prompt to generate anime-style art.count
(number): The number of images to generate.const { animagen } = require('globalsprak');
async function main() {
const prompt = "Create anime-style art";
const count = 3;
const response = await animagen(prompt, count);
console.log("Animagen Response:", response);
}
main();
pixelart(prompt, count)
prompt
(string): The prompt to generate pixel art.count
(number): The number of images to generate.const { pixelart } = require('globalsprak');
async function main() {
const prompt = "Create a pixel art character";
const count = 2;
const response = await pixelArt(prompt, count);
console.log("PixelArt Response:", response);
}
main();
xi(prompt, count)
prompt
(string): The prompt to generate art with the Xi engine.count
(number): The number of images to generate.const { xi } = require('globalsprak');
async function main() {
const prompt = "Create surreal landscape art";
const count = 1;
const response = await xi(prompt, count);
console.log("Xi Response:", response);
}
main();
gen(prompt, count)
prompt
(string): The prompt to generate generic art.count
(number): The number of images to generate.const { gen } = require('globalsprak');
async function main() {
const prompt = "Generate futuristic city art";
const count = 4;
const response = await gen(prompt, count);
console.log("Gen Response:", response);
}
main();
gen2(prompt, count)
prompt
(string): The prompt to generate Gen2 art.count
(number): The number of images to generate.const { gen2 } = require('globalsprak');
async function main() {
const prompt = "Create abstract artwork";
const count = 5;
const response = await gen2(prompt, count);
console.log("Gen2 Response:", response);
}
main();
xl3(prompt)
const { xl3 } = require('globalsprak');
async function main() {
const prompt = "Prompts";
const response = await xl3(prompt);
console.log("XL3 Response:", response);
}
main();
flux(prompt)
const { flux } = require('globalsprak');
async function main() {
const prompt = "Your Flux prompt";
const response = await flux(prompt);
console.log("Flux Response:", response);
}
main();
minidalle(prompt)
const { minidalle } = require('globalsprak');
async function main() {
const prompt = "Your Mini DALL-E prompt";
const response = await minidalle(prompt);
console.log("Mini DALL-E Response:", response);
}
main();
dallev2(prompt)
const { dallev2 } = require('globalsprak');
async function main() {
const prompt = "Your DALL-E v2 prompt";
const response = await dallev2(prompt);
console.log("DALL-E v2 Response:", response);
}
main();
dalle(prompt, prompt_negative, width, height, guidance_scale)
const { dalle } = require('globalsprak');
async function main() {
const prompt = "Your DALL-E v3 prompt";
const prompt_negative = "Negative prompt";
const width = 512;
const height = 512;
const guidance_scale = 7.5;
const response = await dalle(prompt, prompt_negative, width, height, guidance_scale);
console.log("DALL-E v3 Response:", response);
}
main();
xl(prompt, prompt_negative, image_style, guidance_scale)
const { xl } = require('globalsprak');
async function main() {
const prompt = "Your XL prompt";
const prompt_negative = "Negative prompt";
const image_style = "artistic";
const guidance_scale = 7.5;
const response = await xl(prompt, prompt_negative, image_style, guidance_scale);
console.log("XL Response:", response);
}
main();
generate(prompt)
const { generate } = require('globalsprak');
async function main() {
const prompt = "Your text prompt";
const response = await generate(prompt);
console.log("Generate Response:", response);
}
main();
Generate image's using EMI Model.
const { emi } = require('globalsprak');
async function main() {
const prompt = "Cute Girl.";
const response = await emi(prompt);
console.log("EMI Response:", response);
}
main();
Generate image's using EMI Model.
const { bing } = require('globalsprak');
async function main() {
const prompt = "Cute Girl.";
const cookie = "";
const response = await bing(prompt, cookie);
console.log("Bing Response:", response);
}
main();
Generate images using a text prompt and a model.
const { prodia } = require('globalsprak');
async function main() {
const prompt = "A beautiful mountain landscape";
const model = "1";
const response = await prodia(prompt, model);
console.log("ProdIA Response:", response);
}
main();
Please choose Prodia models by number (e.g., 1, 2, 3, etc.).
const { twdl } = require('globalsprak');
async function main() {
const url = "https://twitter.com/example/status/12345";
const response = await twdl(url);
console.log("Twitter Download Response:", response);
}
main();
const { fbdl } = require('globalsprak');
async function main() {
const url = "https://www.facebook.com/example/video/12345";
const response = await fbdl(url);
console.log("Facebook Download Response:", response);
}
main();
const { tkdl } = require('globalsprak');
async function main() {
const url = "https://www.tiktok.com/example/video/12345";
const response = await tkdl(url);
console.log("TikTok Download Response:", response);
}
main();
const { drdl } = require('globalsprak');
async function main() {
const url = "";
const response = await drdl(url);
console.log("Google Drive Download Response:", response);
}
main();
const { instadl } = require('globalsprak');
async function main() {
const url = "https://www.instagram.com/p/12345";
const response = await instadl(url);
console.log("Instagram Download Response:", response);
}
main();
const { ytdl } = require('globalsprak');
async function main() {
const url = "https://www.youtube.com/watch?v=12345";
const response = await ytdl(url);
console.log("YouTube Download Response:", response);
}
main();
Function: spdl(url)
Description: Downloads content from Spotify using the provided URL.
Example:
const { spdl } = require('globalsprak');
async function main() {
const url = "https://open.spotify.com/track/123456";
const response = await spdl(url);
console.log("Spotify Download Response:", response);
}
main();
alldl(url)
const { alldl } = require('globalsprak');
async function main() {
const url = "https://example.com/media";
const response = await alldl(url);
console.log("AllDL Response:", response);
}
main();
const { pinterest } = require('globalsprak');
async function main() {
const query = "nature";
const count = 10;
const response = await pinterest(query, count);
console.log("Pinterest Search Response:", response);
}
main();
const { spsearch } = require('globalsprak');
async function main() {
const query = "Adele";
const response = await spsearch(query);
console.log("Spotify Search Response:", response);
}
main();
const { ytsearch } = require('globalsprak');
async function main() {
const query = "JavaScript tutorials";
const response = await ytsearch(query);
console.log("YouTube Search Response:", response);
}
main();
tiksearch(query)
const { tiksearch } = require('globalsprak');
async function main() {
const query = "dance";
const response = await tiksearch(query);
console.log("TikTok Search Response:", response);
}
main();
Function: wallpaper(query, count)
Description: Search for wallpapers based on a query and number of results.
Example:
const { wallpaper } = require('globalsprak');
async function main() {
const query = "nature";
const count = 5;
const response = await wallpaper(query, count);
console.log("Wallpaper Search Response:", response);
}
main();
Function: shoti()
Description: Fetches TikTok videos using the Shoti API.
Example:
const { shoti } = require('globalsprak');
async function main() {
const response = await shoti();
console.log("ShotI Response:", response);
}
main();
Function: pxsearch(query, count)
Description: Searches for images on Pexels based on the specified query and returns a specified number of results.
Parameters:
query
: The search term.count
: The number of results to return.Example:
const { pxsearch } = require('globalsprak');
async function main() {
const query = "nature";
const count = 5;
const response = await pxsearch(query, count);
console.log("Pexels Search Response:", response);
}
main();
const { waifusearch } = require('globalsprak');
async function main() {
const name = "Naruto";
const response = await waifusearch(name);
console.log("Waifu Search Response:", response);
}
main();
const { waifu } = require('globalsprak');
async function main() {
const response = await waifu();
console.log("Random Waifu Response:", response);
}
main();
movieinfo(name)
const { movieinfo } = require('globalsprak');
async function main() {
const name = "Inception";
const response = await movieinfo(name);
console.log("Movie Info Response:", response);
}
main();
Function: movieinfov2(id)
Description: Get information about a movie using its ID.
Example:
const { movieinfov2 } = require('globalsprak');
async function main() {
const id = "12345";
const response = await movieinfov2(id);
console.log("Movie Info V2 Response:", response);
}
main();
countryinfo(name)
const { countryinfo } = require('globalsprak');
async function main() {
const name = "India";
const response = await countryinfo(name);
console.log("Country Info Response:", response);
}
main();
translate(text, lang)
const { translate } = require('globalsprak');
async function main() {
const text = "Hello, world!";
const lang = "es"; // Spanish
const response = await translate(text, lang);
console.log("Translation:", response);
}
main();
lyrics(songName)
const { lyrics } = require('globalsprak');
async function main() {
const songName = "Bohemian Rhapsody";
const response = await lyrics(songName);
console.log("Lyrics:", response);
}
main();
tikstalk(username)
const { tikstalk } = require('globalsprak');
async function main() {
const username = "user123";
const response = await tikstalk(username);
console.log("TikStalk Data:", response);
}
main();
tempmailGet()
const { tempmailGet } = require('globalsprak');
async function main() {
const response = await tempmailGet();
console.log("Temporary Email:", response);
}
main();
tempmailInbox(email)
const { tempmailInbox } = require('globalsprak');
async function main() {
const email = "your-temp-email@example.com";
const response = await tempmailInbox(email);
console.log("Inbox:", response);
}
main();
quiz(category)
📚 𝗚𝗲𝗻𝗲𝗿𝗮𝗹
➜ english
➜ math
➜ physics
➜ chemistry
➜ history
➜ philosophy
➜ random
➜ science
🖥 𝗣𝗿𝗼𝗴𝗿𝗮𝗺𝗺𝗶𝗻𝗴
➜ coding
➜ javascript
➜ html
➜ java
➜ ruby
➜ python
➜ css
➜ c
➜ c-plus
➜ php
➜ xml
➜ typescript
➜ nodejs
➜ express
🎲 𝗚𝗮𝗺𝗲
➜ minecraft
➜ freefire
➜ roblox
➜ pubg
➜ gta-v
➜ fortnite
🛸 𝗢𝘁𝗵𝗲𝗿
➜ music
➜ youtuber
➜ space
➜ animal
➜ food
➜ country
➜ electronic
➜ sports
🎀 𝗔𝗻𝗶𝗺𝗲
➜ demonslayer
➜ doraemon
➜ anime
➜ one-piece
➜ naruto
➜ deathnote
➜ dragon-ball
➜ attack-on-titan
🌐 𝗟𝗮𝗻𝗴𝘂𝗮𝗴𝗲𝘀
➜ hindi
➜ english
➜ french
➜ filipino
➜ spanish
➜ bengali
➜ vietnamese
➜ japanese
Example Usage:
const { quiz } = require('globalsprak');
async function main() {
const category = "science";
const response = await quiz(category);
console.log("Quiz Data:", response);
}
main();
🥳 Soon I will add more categories and features.
album(category)
➨ 1. English
➨ 2. Anime
➨ 3. Hindi
➨ 4. Free Fire
➨ 5. Football
Soon I will add more album categories.
Example Usage:
const { album } = require('globalsprak');
async function main() {
const category = "Anime";
const response = await album(category);
console.log("Album Data:", response);
}
main();
Get a response from the Weather API based on your City name,
const { weather } = require('globalsprak');
async function main() {
const city = "Nahan";
const response = await weather(city);
console.log("Weather Response:", response);
}
main();
Each function has built-in error handling. If an error occurs during the API request, an error message will be logged, and null
will be returned as the response. Example:
const { gpt } = require('globalsprak');
async function main() {
try {
const response = await gpt("Explain AI");
if (response) {
console.log("GPT Response:", response);
} else {
console.error("Failed to fetch GPT response.");
}
} catch (error) {
console.error("An error occurred:", error);
}
}
main();
Function | Description | Parameters |
---|---|---|
gpt | Get a response from GPT | prompt |
emi | Calculate EMI based on a prompt | prompt |
prodia | Generate an image based on a text prompt and model | prompt , model |
llama | Get a response from the Llama model | prompt |
mixtral | Get a response from the Mixtral model | prompt |
coral | Chat with Coral | prompt |
gptweb | Get web-enhanced GPT responses | prompt |
chatgpt | Get a response from ChatGPT | prompt |
coralchat | Chat with Coral using a specific user ID | prompt , uid |
coralreset | Reset Coral conversation for a specific user | uid |
alldl | Download from any supported URL | url |
twdl | Download media from Twitter | url |
fbdl | Download media from Facebook | url |
tkdl | Download media from TikTok | url |
drdl | Download media from Dailymotion | url |
instadl | Download media from Instagram | url |
ytdl | Download media from YouTube | url |
spdl | Download media from Spotify | url |
pinterest | Search for images on Pinterest | query , count |
spsearch | Search for tracks on Spotify | query |
ytsearch | Search for videos on YouTube | query |
pxsearch | Search for images on Pexels | query , count |
wallpaper | Search for wallpapers | query , count |
tiksearch | Search for TikTok videos | query |
movieinfo | Get detailed information about a movie | name |
movieinfov2 | Get information about a movie using its ID | id |
countryinfo | Retrieve information about a specific country | name |
waifusearch | Find images of characters based on names | name |
waifu | Get a random waifu image | - |
This package is designed to be easy to use and flexible, making it a great choice for integrating AI, media, and utility functions into your applications. For any issues or feature requests, please open an issue in the repository.
FAQs
globalsprak is a Node.js package that provides access to various AI models, image generation, content downloading from various platforms, and more.
We found that globalsprak demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.