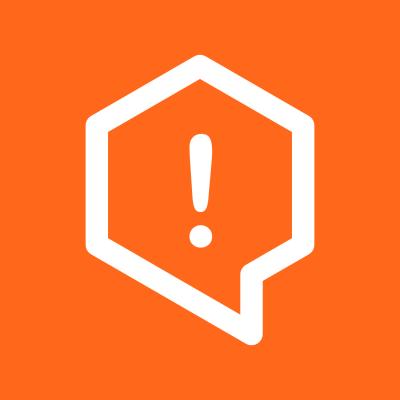
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
glooby-class
Advanced tools
Low level javascript library that helps you to create and work with classes/objects
This library allows you to create Class-like functions in a very effective and nice way. I found this very useful when working with large projects needing structure, scalability and all the other stuff that the ordinary object oriented model gives you in other languages, which JavaScript is kind of missing natively (at least in an easy way).
This project is inspired by John Resig's "Simple JavaScript Inheritance": http://ejohn.org/blog/simple-javascript-inheritance/ and "makeClass": http://ejohn.org/blog/simple-class-instantiation/ They have been integrated with each other and improved in many ways..
I've made a lot of unit testing on this that covers all the functionality, and many ways to use this. Everything around this is also very performance optimized.
The library comes in 3 environment releases:
The source code between these is the same, the only difference is how they are built.
It doesn't have any dependencies and works in all environments. I've ran the unit tests in following environments:
If you find any problems, report a bug, make a pull-request...
The master branch is kind of work in progress, to get the lastest stable version look at the tag with highest version-number...
// You can name 'Class' whatever you want...
var Class = require("path/to/the/lib/node.class.js");
var MyClass = Class({
// define your class...
});
var instance = new MyClass();
var MyClass = Class({
// define your class...
});
var instance = new MyClass();
var MyClass = jQuery.Class({
// define your class...
});
var instance = new MyClass();
This examples below is made with the jQuery release
var YourClass = jQuery.Class({
// Constructor is moved here..
init: function( message ) {
this.message = message;
},
doShit: function() {
// code ....
},
getMessage: function() {
return this.message;
}
// more methods .....
});
var object = new YourClass( "YES" );
// The new keyword isn't needed, but recommended since its
// faster and makes your code more readlable..
var object = YourClass( "YES" );
object.getMessage(); // --> "YES"
object.doShit(); // --> call a method
var ExtendedClass = YourClass.extend({
init: function() {
// constructor code ..
},
doShit: function() {
// code ..
this._parent(); // --> this will call the parent doShit method in the "YourClass" class
// code..
// you could also do like this:
this._parent.getMessage();
// which calls the parent getMessage-method
// that returns this.message instead of null..
},
// Change the behaviour of this
getMessage: function() {
return null;
}
});
ExtendedClass.addMethods({
doSomething: function() {
// code ...
},
doSomethingMore: function() {
// code ...
},
doShit: function() {
this._parent(); // call the doShit method that this method overwrites
}
});
var Class = jQuery.Class({
get: function(){
return "Oh";
}
});
var instance = new Class();
instance.get() === "Oh";
instance.addMethods({
get: function(){
var ret = this._parent();
return ret + "My";
}
});
instance.get() === "OhMy";
var org_instance = new Class();
org_instance.get() === "Oh";
var Base = jQuery.Class({
staticMethod: function() {
return "Hi";
},
prototype: {
init: function() {
this.message = "Hello";
},
getMessage: function() {
return this.message;
}
}
});
var ExtendedClass = Base.extend({
there: " there!",
staticMethod: function(){
return this._parent() + this.there;
},
prototype: {
getMessage: function() {
return this._parent() + ExtendedClass.there;
}
}
});
Base.staticMethod() // -> "Hi"
ExtendedClass.staticMethod() // -> "Hi there!"
var ext = new ExtendedClass();
ext.getMessage() // -> "Hello there!"
var Class = jQuery.Class({
property: "hi there",
prototype: {
get: function() {
return this.constructor.property;
}
}
});
var instance = new Class();
instance.get() -> "hi there"
var Base = jQuery.Class({});
var Ext = Base.extend({});
var Ext2 = Ext.extend({});
Ext.inherits(Base); -> true
Ext.inherits(Ext2); -> false
Ext.inherits(Ext); -> false
Ext2.inherits(Base); -> true
Ext2.inherits(Ext); ->true
var MyClass = $.Class({
prototype: {
// Avoid this as long as the behavior is intended
shared_object: {
prop: 1
},
init: function () {
// A true instance property
this.unshared_object = {
prop: 1
};
}
}
});
var a = new MyClass();
var b = new MyClass();
// This will change shared_object in all
// instances of MyClass the exists today and
// that will be created
a.shared_object.prop = 2;
a.shared_object.prop === 2;
b.shared_object.prop === 2;
// This however will only change 'unshared_object'
// in only instance 'a'.
a.unshared_object.prop = 2;
a.shared_object.prop === 2;
b.unshared_object.prop === 1;
var c = new MyClass();
c.shared_object.prop === 2;
c.shared_object.prop === 1;
FAQs
Low level javascript library that helps you to create and work with classes/objects
The npm package glooby-class receives a total of 7 weekly downloads. As such, glooby-class popularity was classified as not popular.
We found that glooby-class demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.