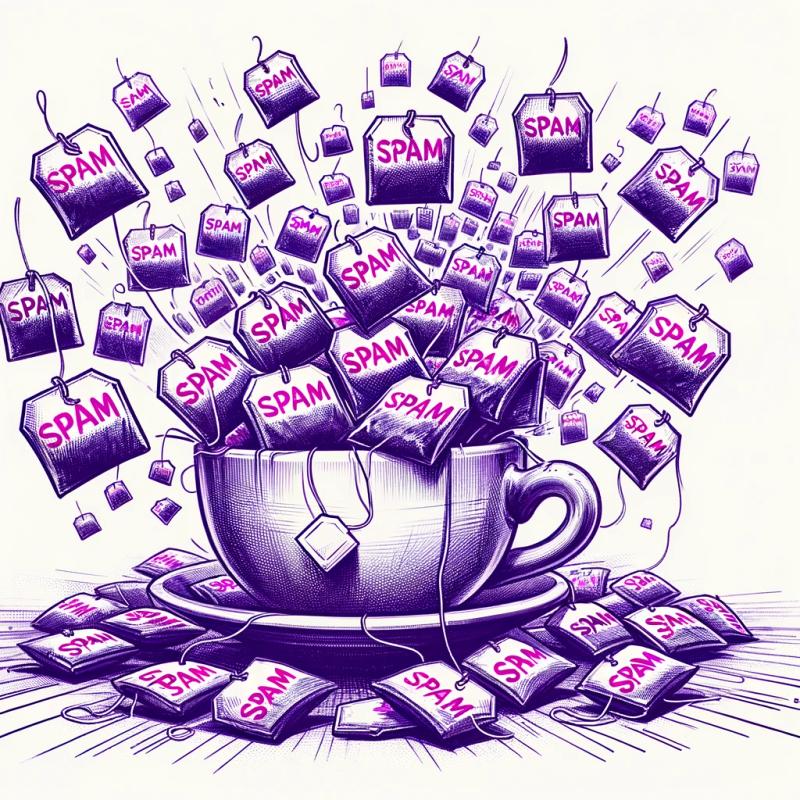
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
google-tts-narrator
Advanced tools
Readme
Use Google Cloud Text-to-Speech to convert SSML (or other types, see below) text into speeches.
GOOGLE_APPLICATION_CREDENTIALS="/FULL/PATH/TO/ACCOUNT_KET.json"
toView here for more Infomations
As a module
npm i google-tts-narrator
As a cli app
npx google-tts-narrator --from my_scenario.ssml.pug --to my_scenario_sounds/
Google Cloud Text-to-Speech only allowed ONE <speak></speak>
for each call,
this library will analyze your SSML file and call for each <speak></speak>
elements with different configs.
You need to set audioConfig
and voice
attributes for each dialogue, which could found & tested here and here.
<speak audioConfig='{"audioEncoding": "MP3", "pitch": 0, "speakingRate": 1}'
voice='{"languageCode": "en-US", "name": "en-US-Wavenet-G"}'>
The <say-as interpret-as="characters">SSML</say-as>standard
<break time="1s"/>is defined by the
<sub alias="World Wide Web Consortium">W3C</sub>.
</speak>
<speak audioConfig='{"audioEncoding": "MP3", "pitch": 0, "speakingRate": 1}'
voice='{"languageCode": "en-ES", "name": "es-ES-Standard-C", "ssmlGender": "MALE"}'>
¡Hola<break time="200ms"/>mundo!
</speak>
Upper scenario file will convert to 2 sound tracks:
demo_1.mp3
demo_2.mp3
Pug is an indent style HTML template DSL(Domain-Specific Language), it can also compiled to XML files.
SSML upper also could written like below
speak(
audioconfig='{"audioEncoding": "MP3", "pitch": 0, "speakingRate": 1}'
voice='{"languageCode": "en-US", "name": "en-US-Wavenet-G"}')
| The #[say-as(interpret-as='characters') SSML] standard #[break(time='1s')/]
| is defined by the #[sub(alias='World Wide Web Consortium') W3C].
speak(
audioconfig='{"audioEncoding": "MP3", "pitch": 0, "speakingRate": 1}'
voice='{"languageCode": "en-ES", "name": "es-ES-Standard-C", "ssmlGender": "MALE"}')
| ¡Hola#[break(time='200ms')/] mundo!
Even could separate speakers' settings from scripts, it will made dialogue script more smoothly.
[Warning] Only Cli mode can deal with separated pug files!
//- _speaker.pug
mixin enSpeaker(audioConfig = {}, voice = {})
-
audioConfig = Object.assign({
speakingRate: 1.12, audioEncoding: 'MP3',
}, audioConfig)
voice = Object.assign({
languageCode: 'en-US', ssmlGender: 'MALE',
}, voice)
speak(audioConfig=audioConfig voice=voice)
block
mixin jaSpeaker(audioConfig = {}, voice = {})
-
audioConfig = Object.assign({
pitch: 1.6, speakingRate: 1.12,
audioEncoding: 'MP3',
}, audioConfig)
voice = Object.assign({
languageCode: 'ja-JP', name: 'ja-JP-Wavenet-A',
}, voice)
speak(audioConfig=audioConfig voice=voice)
block
//- include_demo.pug
include _speaker.pug
+enSpeaker Hello!
+jaSpeaker こんにちは!
+enSpeaker Goodbye!
+jaSpeaker さようなら!
You may also create an array of Text-to-Speech params in JSON or YAML
YAML
- &staff # Setup staff
audioConfig:
audioEncoding: MP3
pitch: 1.6
speakingRate: 1.07
voice:
languageCode: ja-JP
name: ja-JP-Wavenet-A
input:
text: いらっしゃいませ。
- &customer # Setup customer
audioConfig:
audioEncoding: MP3
pitch: 1.6
speakingRate: 1.07
voice:
languageCode: ja-JP
name: ja-JP-Wavenet-D
input:
text: チーズバーガーをください。
-
<<: *staff
input:
ssml: |-
<speak>
かしこまりました。お召し上がりですか?<break time="300ms"/>お持ち帰りですか?
</speak>
-
<<: *customer
input:
text: 持ち帰ります。
JSON
[
{
"voice": {
"languageCode": "en-US",
"ssmlGender": "NEUTRAL"
},
"audioConfig": {
"audioEncoding": "MP3"
},
"input": {
"text": "Hello, world!"
}
},
{
"voice": {
"languageCode": "en-ES",
"name": "es-ES-Standard-C"
},
"audioConfig": {
"audioEncoding": "MP3"
},
"input": {
"text": "¡Hola, mundo!"
}
}
]
Make sure GOOGLE_APPLICATION_CREDENTIALS
is set, and Cloud Text-to-Speech API permission enabled.
Run command below to generate sound tracks.
npx google-tts-narrator -s PATH/TO/FILE
System will guess type by your scenario file's extension, and create a directory for sound tracks if not given.
Options:
-s, --from Your Scenario File Path [required]
-d, --to Target Directory Path
-t, --type Scenario File Type, One of [pug|xml|yaml|json]
-h, --help Show help [boolean]
-v, --version Show version number [boolean]
Make sure GOOGLE_APPLICATION_CREDENTIALS
is set, or set credentials manually.
const Narrator = require('google-tts-narrator');
const options = { credentials: require('/PATH/TO/credentials.json') }
const scenario = fs.readFileSync('PATH/TO/scenario.pug', 'utf-8');
const gen = Narrator.yieldSpeeches(scenario, 'pug', options);
...
API mode only support single file scenario
Use Generate Style API to create sounds step by step, it will save the costs.
const fs = require('fs');
const Narrator = require('google-tts-narrator');
const scenario = fs.readFileSync('PATH/TO/scenario.pug', 'utf-8');
const gen = Narrator.yieldSpeeches(scenario, 'pug');
(async () => {
for await (let speach of gen) {
const { step } = speach.narratorInfo;
fs.writeFileSync(`PATH/TO/DIR/${step}.mp3`, speach.audioContent, 'binary');
}
})();
Classical async/await style also can be used to batch create sound tracks.
const fs = require('fs');
const Narrator = require('google-tts-narrator');
const scenario = fs.readFileSync('PATH/TO/scenario.pug', 'utf-8');
(async () => {
const speaches = await Narrator.fetchSpeeches();
speaches.forEach((speach, i) => {
fs.writeFileSync(`PATH/TO/DIR/${i}.mp3`, speach.audioContent, 'binary');
})
})();
Ms Rie Ishikawa of Rose Ladies School provides all Japanese dialogue samples for kindness.
All copyrights of Japanese dialogue samples belongs to Rose Ladies School. Rie Ishikawa.
MIT(Except Japanese Dialogue Samples)
FAQs
A Google Text-to-Speech Narrator
The npm package google-tts-narrator receives a total of 0 weekly downloads. As such, google-tts-narrator popularity was classified as not popular.
We found that google-tts-narrator demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.