gradienticons
Advanced tools
Comparing version 1.0.1 to 1.0.2
243
dist/Icon.js
@@ -29,59 +29,46 @@ "use strict"; | ||
icons: _iconData.iconData.icons || _iconData.iconData, | ||
getIcons: function getIcons() { | ||
return this.icons; | ||
}, | ||
getIcon: function getIcon(name) { | ||
return this.icons.find(function (icon) { | ||
return icon.properties ? icon.properties.name === name : icon.name === name; | ||
return IconService.icons.find(function (icon) { | ||
var _icon$properties; | ||
return (((_icon$properties = icon.properties) === null || _icon$properties === void 0 ? void 0 : _icon$properties.name) || icon.name) === name; | ||
}); | ||
} | ||
}; | ||
var getGradientDirection = function getGradientDirection(direction) { | ||
var directions = { | ||
top: { | ||
x: '50%', | ||
y: '0%' | ||
}, | ||
bottom: { | ||
x: '50%', | ||
y: '100%' | ||
}, | ||
left: { | ||
x: '0%', | ||
y: '50%' | ||
}, | ||
right: { | ||
x: '100%', | ||
y: '50%' | ||
}, | ||
topLeft: { | ||
x: '0%', | ||
y: '0%' | ||
}, | ||
topRight: { | ||
x: '100%', | ||
y: '0%' | ||
}, | ||
bottomLeft: { | ||
x: '0%', | ||
y: '100%' | ||
}, | ||
bottomRight: { | ||
x: '100%', | ||
y: '100%' | ||
} | ||
}; | ||
return directions[direction] || { | ||
var DIRECTIONS = { | ||
top: { | ||
x: '50%', | ||
y: '0%' | ||
}, | ||
bottom: { | ||
x: '50%', | ||
y: '100%' | ||
}, | ||
left: { | ||
x: '0%', | ||
y: '50%' | ||
}, | ||
right: { | ||
x: '100%', | ||
y: '50%' | ||
}, | ||
topLeft: { | ||
x: '0%', | ||
y: '0%' | ||
}; | ||
}, | ||
topRight: { | ||
x: '100%', | ||
y: '0%' | ||
}, | ||
bottomLeft: { | ||
x: '0%', | ||
y: '100%' | ||
}, | ||
bottomRight: { | ||
x: '100%', | ||
y: '100%' | ||
} | ||
}; | ||
var parseSize = function parseSize(value) { | ||
var defaultValue = arguments.length > 1 && arguments[1] !== undefined ? arguments[1] : '1em'; | ||
if (typeof value === 'number') return "".concat(value, "px"); | ||
if (typeof value === 'string') { | ||
var num = parseFloat(value); | ||
return isNaN(num) ? value : "".concat(num, "px"); | ||
} | ||
return defaultValue; | ||
return typeof value === 'number' ? "".concat(value, "px") : typeof value === 'string' ? isNaN(parseFloat(value)) ? value : "".concat(parseFloat(value), "px") : defaultValue; | ||
}; | ||
@@ -93,6 +80,3 @@ var parseColor = function parseColor(color) { | ||
var parseGradientColors = function parseGradientColors(colors) { | ||
if (Array.isArray(colors) && colors.length >= 2) { | ||
return [parseColor(colors[0]), parseColor(colors[1])]; | ||
} | ||
return null; | ||
return Array.isArray(colors) && colors.length >= 2 ? [parseColor(colors[0]), parseColor(colors[1])] : null; | ||
}; | ||
@@ -104,4 +88,3 @@ var parseNumber = function parseNumber(value) { | ||
}; | ||
var Icon = function Icon(_ref) { | ||
var _iconData$icon, _iconData$icon2; | ||
var Icon = /*#__PURE__*/_react["default"].memo(function (_ref) { | ||
var name = _ref.name, | ||
@@ -145,55 +128,88 @@ _ref$size = _ref.size, | ||
props = _objectWithoutProperties(_ref, _excluded); | ||
var _useState = (0, _react.useState)(null), | ||
var _useState = (0, _react.useState)(false), | ||
_useState2 = _slicedToArray(_useState, 2), | ||
iconData = _useState2[0], | ||
setIconData = _useState2[1]; | ||
var _useState3 = (0, _react.useState)(false), | ||
_useState4 = _slicedToArray(_useState3, 2), | ||
isHovered = _useState4[0], | ||
setIsHovered = _useState4[1]; | ||
var _useState5 = (0, _react.useState)(null), | ||
_useState6 = _slicedToArray(_useState5, 2), | ||
error = _useState6[0], | ||
setError = _useState6[1]; | ||
(0, _react.useEffect)(function () { | ||
try { | ||
var icon = IconService.getIcon(name); | ||
if (!icon) { | ||
throw new Error("Icon \"".concat(name, "\" not found")); | ||
} | ||
setIconData(icon); | ||
setError(null); | ||
} catch (err) { | ||
setError(err.message); | ||
isHovered = _useState2[0], | ||
setIsHovered = _useState2[1]; | ||
var memoizedValues = (0, _react.useMemo)(function () { | ||
var _icon$icon, _icon$icon2; | ||
var icon = IconService.getIcon(name); | ||
if (!icon) { | ||
console.error("Icon \"".concat(name, "\" not found")); | ||
return null; | ||
} | ||
}, [name]); | ||
if (error) { | ||
console.error("Error in Icon component: ".concat(error)); | ||
return null; // or return a fallback icon | ||
} | ||
var viewBox = (iconData === null || iconData === void 0 || (_iconData$icon = iconData.icon) === null || _iconData$icon === void 0 ? void 0 : _iconData$icon.viewBox) || (iconData === null || iconData === void 0 ? void 0 : iconData.viewBox) || '0 0 1024 1024'; | ||
var paths = (iconData === null || iconData === void 0 || (_iconData$icon2 = iconData.icon) === null || _iconData$icon2 === void 0 ? void 0 : _iconData$icon2.paths) || (iconData === null || iconData === void 0 ? void 0 : iconData.paths) || []; | ||
var parsedSize = parseSize(size); | ||
var parsedColor = parseColor(color); | ||
var parsedHoverColor = parseColor(hoverColor); | ||
var parsedBackgroundColor = parseColor(backgroundColor); | ||
var parsedHoverBackgroundColor = parseColor(hoverBackgroundColor); | ||
var parsedGradientColors = parseGradientColors(gradientColors); | ||
var parsedHoverGradientColors = parseGradientColors(hoverGradientColors); | ||
var parsedOpacity = parseNumber(opacity, 1); | ||
var parsedBorderRadius = parseNumber(borderRadius); | ||
var parsedPadding = parseNumber(padding); | ||
var parsedOuterPadding = parseNumber(outerPadding); | ||
var activeColor = isHovered ? parsedHoverColor : parsedColor; | ||
var activeGradientColors = isHovered ? parsedHoverGradientColors : parsedGradientColors; | ||
var activeBackgroundColor = isHovered ? parsedHoverBackgroundColor : parsedBackgroundColor; | ||
var start = getGradientDirection(directionStart); | ||
var end = getGradientDirection(directionEnd); | ||
var _viewBox$split$slice$ = viewBox.split(' ').slice(2).map(Number), | ||
_viewBox$split$slice$2 = _slicedToArray(_viewBox$split$slice$, 2), | ||
viewBoxWidth = _viewBox$split$slice$2[0], | ||
viewBoxHeight = _viewBox$split$slice$2[1]; | ||
var totalSize = parseFloat(parsedSize) + 2 * parsedOuterPadding; | ||
var innerSize = parseFloat(parsedSize) - 2 * parsedPadding; | ||
var scaleFactor = innerSize / viewBoxWidth; | ||
var parsedSize = parseSize(size); | ||
var parsedColor = parseColor(color); | ||
var parsedHoverColor = parseColor(hoverColor); | ||
var parsedBackgroundColor = parseColor(backgroundColor); | ||
var parsedHoverBackgroundColor = parseColor(hoverBackgroundColor); | ||
var parsedGradientColors = parseGradientColors(gradientColors); | ||
var parsedHoverGradientColors = parseGradientColors(hoverGradientColors); | ||
var parsedOpacity = parseNumber(opacity, 1); | ||
var parsedBorderRadius = parseNumber(borderRadius); | ||
var parsedPadding = parseNumber(padding); | ||
var parsedOuterPadding = parseNumber(outerPadding); | ||
var start = DIRECTIONS[directionStart] || DIRECTIONS.topLeft; | ||
var end = DIRECTIONS[directionEnd] || DIRECTIONS.bottomRight; | ||
var viewBox = ((_icon$icon = icon.icon) === null || _icon$icon === void 0 ? void 0 : _icon$icon.viewBox) || icon.viewBox || '0 0 1024 1024'; | ||
var paths = ((_icon$icon2 = icon.icon) === null || _icon$icon2 === void 0 ? void 0 : _icon$icon2.paths) || icon.paths || []; | ||
var _viewBox$split$slice$ = viewBox.split(' ').slice(2).map(Number), | ||
_viewBox$split$slice$2 = _slicedToArray(_viewBox$split$slice$, 2), | ||
viewBoxWidth = _viewBox$split$slice$2[0], | ||
viewBoxHeight = _viewBox$split$slice$2[1]; | ||
var totalSize = parseFloat(parsedSize) + 2 * parsedOuterPadding; | ||
var innerSize = parseFloat(parsedSize) - 2 * parsedPadding; | ||
var scaleFactor = innerSize / viewBoxWidth; | ||
return { | ||
icon: icon, | ||
parsedSize: parsedSize, | ||
parsedColor: parsedColor, | ||
parsedHoverColor: parsedHoverColor, | ||
parsedBackgroundColor: parsedBackgroundColor, | ||
parsedHoverBackgroundColor: parsedHoverBackgroundColor, | ||
parsedGradientColors: parsedGradientColors, | ||
parsedHoverGradientColors: parsedHoverGradientColors, | ||
parsedOpacity: parsedOpacity, | ||
parsedBorderRadius: parsedBorderRadius, | ||
parsedPadding: parsedPadding, | ||
parsedOuterPadding: parsedOuterPadding, | ||
start: start, | ||
end: end, | ||
viewBox: viewBox, | ||
paths: paths, | ||
viewBoxWidth: viewBoxWidth, | ||
viewBoxHeight: viewBoxHeight, | ||
totalSize: totalSize, | ||
innerSize: innerSize, | ||
scaleFactor: scaleFactor | ||
}; | ||
}, [name, size, color, hoverColor, backgroundColor, hoverBackgroundColor, gradientColors, hoverGradientColors, opacity, borderRadius, padding, outerPadding, directionStart, directionEnd]); | ||
var handleMouseEnter = (0, _react.useCallback)(function () { | ||
return setIsHovered(true); | ||
}, []); | ||
var handleMouseLeave = (0, _react.useCallback)(function () { | ||
return setIsHovered(false); | ||
}, []); | ||
if (!memoizedValues) return null; | ||
var parsedSize = memoizedValues.parsedSize, | ||
parsedColor = memoizedValues.parsedColor, | ||
parsedHoverColor = memoizedValues.parsedHoverColor, | ||
parsedBackgroundColor = memoizedValues.parsedBackgroundColor, | ||
parsedHoverBackgroundColor = memoizedValues.parsedHoverBackgroundColor, | ||
parsedGradientColors = memoizedValues.parsedGradientColors, | ||
parsedHoverGradientColors = memoizedValues.parsedHoverGradientColors, | ||
parsedOpacity = memoizedValues.parsedOpacity, | ||
parsedBorderRadius = memoizedValues.parsedBorderRadius, | ||
parsedPadding = memoizedValues.parsedPadding, | ||
parsedOuterPadding = memoizedValues.parsedOuterPadding, | ||
start = memoizedValues.start, | ||
end = memoizedValues.end, | ||
viewBox = memoizedValues.viewBox, | ||
paths = memoizedValues.paths, | ||
viewBoxWidth = memoizedValues.viewBoxWidth, | ||
viewBoxHeight = memoizedValues.viewBoxHeight, | ||
totalSize = memoizedValues.totalSize, | ||
scaleFactor = memoizedValues.scaleFactor; | ||
var activeColor = isHovered && hoverColor ? parsedHoverColor : parsedColor; | ||
var activeGradientColors = isHovered && hoverGradientColors ? parsedHoverGradientColors : parsedGradientColors; | ||
var activeBackgroundColor = isHovered && hoverBackgroundColor ? parsedHoverBackgroundColor : parsedBackgroundColor; | ||
var shapeElement = shape === 'circle' ? /*#__PURE__*/_react["default"].createElement("circle", { | ||
@@ -231,8 +247,4 @@ cx: totalSize / 2, | ||
style: svgStyle, | ||
onMouseEnter: function onMouseEnter() { | ||
return setIsHovered(true); | ||
}, | ||
onMouseLeave: function onMouseLeave() { | ||
return setIsHovered(false); | ||
} | ||
onMouseEnter: handleMouseEnter, | ||
onMouseLeave: handleMouseLeave | ||
}, props), /*#__PURE__*/_react["default"].createElement("defs", null, activeGradientColors && /*#__PURE__*/_react["default"].createElement("linearGradient", { | ||
@@ -252,3 +264,3 @@ id: "iconGradient-".concat(name), | ||
transform: "translate(".concat(parsedOuterPadding + parsedPadding, ", ").concat(parsedOuterPadding + parsedPadding, ") scale(").concat(scaleFactor, ")") | ||
}, activeGradientColors ? /*#__PURE__*/_react["default"].createElement("mask", { | ||
}, activeGradientColors ? /*#__PURE__*/_react["default"].createElement(_react["default"].Fragment, null, /*#__PURE__*/_react["default"].createElement("mask", { | ||
id: "iconMask-".concat(name) | ||
@@ -261,3 +273,3 @@ }, paths.map(function (path, index) { | ||
}); | ||
})) : null, activeGradientColors ? /*#__PURE__*/_react["default"].createElement("rect", { | ||
})), /*#__PURE__*/_react["default"].createElement("rect", { | ||
x: "0", | ||
@@ -269,3 +281,3 @@ y: "0", | ||
mask: "url(#iconMask-".concat(name, ")") | ||
}) : paths.map(function (path, index) { | ||
})) : paths.map(function (path, index) { | ||
return /*#__PURE__*/_react["default"].createElement("path", { | ||
@@ -285,3 +297,4 @@ key: index, | ||
}, svgContent) : svgContent); | ||
}; | ||
}); | ||
Icon.displayName = 'Icon'; | ||
var _default = exports["default"] = Icon; |
{ | ||
"name": "gradienticons", | ||
"version": "1.0.1", | ||
"version": "1.0.2", | ||
"description": "A versatile React icon component library with gradient support, hover effects, and customizable shapes. Perfect for modern UI designs.", | ||
@@ -5,0 +5,0 @@ "main": "dist/index.js", |
104
README.md
# Gradienticons | ||
Gradienticons is a versatile and customizable React icon component library with support for gradients, hover effects, and shape variations. It offers flexible input handling for all props, making it easy to use in various scenarios. | ||
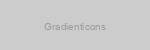 | ||
Gradienticons is a powerful and flexible React icon component library that brings your icons to life with gradient support, hover effects, and shape variations. With its intuitive API and flexible input handling, Gradienticons makes it easy to create stunning, customizable icons for any React project. | ||
[](https://badge.fury.io/js/gradienticons) | ||
[](https://opensource.org/licenses/MIT) | ||
[](https://reactjs.org/) | ||
## Features | ||
@@ -15,4 +21,16 @@ | ||
## Table of Contents | ||
- [Installation](#installation) | ||
- [Quick Start](#quick-start) | ||
- [Usage](#usage) | ||
- [API Reference](#api-reference) | ||
- [Flexible Input Handling](#flexible-input-handling) | ||
- [Contributing](#contributing) | ||
- [License](#license) | ||
## Installation | ||
You can install Gradienticons using npm or yarn: | ||
```bash | ||
@@ -28,5 +46,34 @@ npm install gradienticons | ||
## Quick Start | ||
Here's a quick example to get you started with Gradienticons: | ||
```jsx | ||
import React from 'react'; | ||
import { Icon } from 'gradienticons'; | ||
function App() { | ||
return ( | ||
<div> | ||
<h1>Welcome to My App</h1> | ||
<Icon | ||
name="home" | ||
size={50} | ||
gradientColors={['#ff00ff', '#00ffff']} | ||
shape="circle" | ||
backgroundColor="#f0f0f0" | ||
/> | ||
</div> | ||
); | ||
} | ||
export default App; | ||
``` | ||
## Usage | ||
Gradienticons is designed to be flexible and easy to use. Here's a more detailed example showcasing various features: | ||
```jsx | ||
import React from 'react'; | ||
import { Icon } from 'gradienticons'; | ||
@@ -36,22 +83,31 @@ | ||
return ( | ||
<Icon | ||
name="home" | ||
size={50} // or "50px" or "3em" | ||
color="blue" | ||
gradientColors={['#ff00ff', '#00ffff']} | ||
hoverColor="red" | ||
shape="circle" | ||
backgroundColor="#f0f0f0" | ||
outerPadding={10} // or "10px" | ||
opacity={0.8} // or "0.8" | ||
/> | ||
<div> | ||
<Icon | ||
name="home" | ||
size={50} // or "50px" or "3em" | ||
color="blue" | ||
gradientColors={['#ff00ff', '#00ffff']} | ||
hoverColor="red" | ||
hoverGradientColors={['#ff0000', '#0000ff']} | ||
shape="circle" | ||
backgroundColor="#f0f0f0" | ||
hoverBackgroundColor="#e0e0e0" | ||
outerPadding={10} // or "10px" | ||
opacity={0.8} // or "0.8" | ||
boxShadow="0 4px 6px rgba(0, 0, 0, 0.1)" | ||
iconShadow="2px 2px 2px rgba(0, 0, 0, 0.5)" | ||
cursor="pointer" | ||
/> | ||
</div> | ||
); | ||
} | ||
export default MyComponent; | ||
``` | ||
## API | ||
## API Reference | ||
| Prop | Type | Default | Description | | ||
| -------------------- | ---------------- | -------------- | ---------------------------------------- | | ||
| name | string | - | Name of the icon | | ||
| name | string | - | Name of the icon (required) | | ||
| size | string \| number | '1em' | Size of the icon | | ||
@@ -77,4 +133,6 @@ | color | string | 'currentColor' | Color of the icon | | ||
Gradienticons now supports flexible input handling for all props. You can pass values as strings or numbers, and they will be correctly parsed and applied. For example: | ||
Gradienticons supports flexible input handling for all props. You can pass values as strings or numbers, and they will be correctly parsed and applied. This flexibility makes it easier to use Gradienticons in various contexts and with different styling approaches. | ||
Example: | ||
```jsx | ||
@@ -89,6 +147,20 @@ <Icon | ||
This flexibility makes it easier to use Gradienticons in various contexts and with different styling approaches. | ||
## Contributing | ||
We welcome contributions to Gradienticons! If you'd like to contribute, please follow these steps: | ||
1. Fork the repository | ||
2. Create your feature branch (`git checkout -b feature/AmazingFeature`) | ||
3. Commit your changes (`git commit -m 'Add some AmazingFeature'`) | ||
4. Push to the branch (`git push origin feature/AmazingFeature`) | ||
5. Open a Pull Request | ||
Please make sure to update tests as appropriate and adhere to the existing coding style. | ||
## License | ||
Distributed under the MIT License. See `LICENSE` for more information. | ||
--- | ||
MIT © [DHEERAJ] |
464
src/Icon.js
@@ -1,2 +0,2 @@ | ||
import React, { useState, useEffect } from 'react'; | ||
import React, { useMemo, useState, useCallback } from 'react'; | ||
import { iconData } from './iconData'; | ||
@@ -6,47 +6,35 @@ | ||
icons: iconData.icons || iconData, | ||
getIcons() { | ||
return this.icons; | ||
}, | ||
getIcon(name) { | ||
return this.icons.find((icon) => { | ||
return icon.properties | ||
? icon.properties.name === name | ||
: icon.name === name; | ||
}); | ||
}, | ||
getIcon: (name) => | ||
IconService.icons.find( | ||
(icon) => (icon.properties?.name || icon.name) === name | ||
), | ||
}; | ||
const getGradientDirection = (direction) => { | ||
const directions = { | ||
top: { x: '50%', y: '0%' }, | ||
bottom: { x: '50%', y: '100%' }, | ||
left: { x: '0%', y: '50%' }, | ||
right: { x: '100%', y: '50%' }, | ||
topLeft: { x: '0%', y: '0%' }, | ||
topRight: { x: '100%', y: '0%' }, | ||
bottomLeft: { x: '0%', y: '100%' }, | ||
bottomRight: { x: '100%', y: '100%' }, | ||
}; | ||
return directions[direction] || { x: '0%', y: '0%' }; | ||
const DIRECTIONS = { | ||
top: { x: '50%', y: '0%' }, | ||
bottom: { x: '50%', y: '100%' }, | ||
left: { x: '0%', y: '50%' }, | ||
right: { x: '100%', y: '50%' }, | ||
topLeft: { x: '0%', y: '0%' }, | ||
topRight: { x: '100%', y: '0%' }, | ||
bottomLeft: { x: '0%', y: '100%' }, | ||
bottomRight: { x: '100%', y: '100%' }, | ||
}; | ||
const parseSize = (value, defaultValue = '1em') => { | ||
if (typeof value === 'number') return `${value}px`; | ||
if (typeof value === 'string') { | ||
const num = parseFloat(value); | ||
return isNaN(num) ? value : `${num}px`; | ||
} | ||
return defaultValue; | ||
}; | ||
const parseSize = (value, defaultValue = '1em') => | ||
typeof value === 'number' | ||
? `${value}px` | ||
: typeof value === 'string' | ||
? isNaN(parseFloat(value)) | ||
? value | ||
: `${parseFloat(value)}px` | ||
: defaultValue; | ||
const parseColor = (color, defaultColor = 'currentColor') => { | ||
return color || defaultColor; | ||
}; | ||
const parseColor = (color, defaultColor = 'currentColor') => | ||
color || defaultColor; | ||
const parseGradientColors = (colors) => { | ||
if (Array.isArray(colors) && colors.length >= 2) { | ||
return [parseColor(colors[0]), parseColor(colors[1])]; | ||
} | ||
return null; | ||
}; | ||
const parseGradientColors = (colors) => | ||
Array.isArray(colors) && colors.length >= 2 | ||
? [parseColor(colors[0]), parseColor(colors[1])] | ||
: null; | ||
@@ -58,188 +46,250 @@ const parseNumber = (value, defaultValue = 0) => { | ||
const Icon = ({ | ||
name, | ||
size = '1em', | ||
color = 'currentColor', | ||
gradientColors = null, | ||
hoverColor = null, | ||
hoverGradientColors = null, | ||
directionStart = 'topLeft', | ||
directionEnd = 'bottomRight', | ||
styles = {}, | ||
opacity = 1, | ||
backgroundColor = 'transparent', | ||
hoverBackgroundColor = null, | ||
shape = 'circle', | ||
borderRadius = 0, | ||
padding = 0, | ||
outerPadding = 0, | ||
boxShadow = null, | ||
iconShadow = null, | ||
cursor = 'default', | ||
...props | ||
}) => { | ||
const [iconData, setIconData] = useState(null); | ||
const [isHovered, setIsHovered] = useState(false); | ||
const [error, setError] = useState(null); | ||
const Icon = React.memo( | ||
({ | ||
name, | ||
size = '1em', | ||
color = 'currentColor', | ||
gradientColors = null, | ||
hoverColor = null, | ||
hoverGradientColors = null, | ||
directionStart = 'topLeft', | ||
directionEnd = 'bottomRight', | ||
styles = {}, | ||
opacity = 1, | ||
backgroundColor = 'transparent', | ||
hoverBackgroundColor = null, | ||
shape = 'circle', | ||
borderRadius = 0, | ||
padding = 0, | ||
outerPadding = 0, | ||
boxShadow = null, | ||
iconShadow = null, | ||
cursor = 'default', | ||
...props | ||
}) => { | ||
const [isHovered, setIsHovered] = useState(false); | ||
useEffect(() => { | ||
try { | ||
const memoizedValues = useMemo(() => { | ||
const icon = IconService.getIcon(name); | ||
if (!icon) { | ||
throw new Error(`Icon "${name}" not found`); | ||
console.error(`Icon "${name}" not found`); | ||
return null; | ||
} | ||
setIconData(icon); | ||
setError(null); | ||
} catch (err) { | ||
setError(err.message); | ||
} | ||
}, [name]); | ||
if (error) { | ||
console.error(`Error in Icon component: ${error}`); | ||
return null; // or return a fallback icon | ||
} | ||
const parsedSize = parseSize(size); | ||
const parsedColor = parseColor(color); | ||
const parsedHoverColor = parseColor(hoverColor); | ||
const parsedBackgroundColor = parseColor(backgroundColor); | ||
const parsedHoverBackgroundColor = parseColor(hoverBackgroundColor); | ||
const parsedGradientColors = parseGradientColors(gradientColors); | ||
const parsedHoverGradientColors = | ||
parseGradientColors(hoverGradientColors); | ||
const parsedOpacity = parseNumber(opacity, 1); | ||
const parsedBorderRadius = parseNumber(borderRadius); | ||
const parsedPadding = parseNumber(padding); | ||
const parsedOuterPadding = parseNumber(outerPadding); | ||
const start = DIRECTIONS[directionStart] || DIRECTIONS.topLeft; | ||
const end = DIRECTIONS[directionEnd] || DIRECTIONS.bottomRight; | ||
const viewBox = icon.icon?.viewBox || icon.viewBox || '0 0 1024 1024'; | ||
const paths = icon.icon?.paths || icon.paths || []; | ||
const viewBox = | ||
iconData?.icon?.viewBox || iconData?.viewBox || '0 0 1024 1024'; | ||
const paths = iconData?.icon?.paths || iconData?.paths || []; | ||
const [viewBoxWidth, viewBoxHeight] = viewBox | ||
.split(' ') | ||
.slice(2) | ||
.map(Number); | ||
const totalSize = parseFloat(parsedSize) + 2 * parsedOuterPadding; | ||
const innerSize = parseFloat(parsedSize) - 2 * parsedPadding; | ||
const scaleFactor = innerSize / viewBoxWidth; | ||
const parsedSize = parseSize(size); | ||
const parsedColor = parseColor(color); | ||
const parsedHoverColor = parseColor(hoverColor); | ||
const parsedBackgroundColor = parseColor(backgroundColor); | ||
const parsedHoverBackgroundColor = parseColor(hoverBackgroundColor); | ||
const parsedGradientColors = parseGradientColors(gradientColors); | ||
const parsedHoverGradientColors = parseGradientColors(hoverGradientColors); | ||
const parsedOpacity = parseNumber(opacity, 1); | ||
const parsedBorderRadius = parseNumber(borderRadius); | ||
const parsedPadding = parseNumber(padding); | ||
const parsedOuterPadding = parseNumber(outerPadding); | ||
return { | ||
icon, | ||
parsedSize, | ||
parsedColor, | ||
parsedHoverColor, | ||
parsedBackgroundColor, | ||
parsedHoverBackgroundColor, | ||
parsedGradientColors, | ||
parsedHoverGradientColors, | ||
parsedOpacity, | ||
parsedBorderRadius, | ||
parsedPadding, | ||
parsedOuterPadding, | ||
start, | ||
end, | ||
viewBox, | ||
paths, | ||
viewBoxWidth, | ||
viewBoxHeight, | ||
totalSize, | ||
innerSize, | ||
scaleFactor, | ||
}; | ||
}, [ | ||
name, | ||
size, | ||
color, | ||
hoverColor, | ||
backgroundColor, | ||
hoverBackgroundColor, | ||
gradientColors, | ||
hoverGradientColors, | ||
opacity, | ||
borderRadius, | ||
padding, | ||
outerPadding, | ||
directionStart, | ||
directionEnd, | ||
]); | ||
const activeColor = isHovered ? parsedHoverColor : parsedColor; | ||
const activeGradientColors = isHovered | ||
? parsedHoverGradientColors | ||
: parsedGradientColors; | ||
const activeBackgroundColor = isHovered | ||
? parsedHoverBackgroundColor | ||
: parsedBackgroundColor; | ||
const handleMouseEnter = useCallback(() => setIsHovered(true), []); | ||
const handleMouseLeave = useCallback(() => setIsHovered(false), []); | ||
const start = getGradientDirection(directionStart); | ||
const end = getGradientDirection(directionEnd); | ||
if (!memoizedValues) return null; | ||
const [viewBoxWidth, viewBoxHeight] = viewBox.split(' ').slice(2).map(Number); | ||
const totalSize = parseFloat(parsedSize) + 2 * parsedOuterPadding; | ||
const innerSize = parseFloat(parsedSize) - 2 * parsedPadding; | ||
const scaleFactor = innerSize / viewBoxWidth; | ||
const { | ||
parsedSize, | ||
parsedColor, | ||
parsedHoverColor, | ||
parsedBackgroundColor, | ||
parsedHoverBackgroundColor, | ||
parsedGradientColors, | ||
parsedHoverGradientColors, | ||
parsedOpacity, | ||
parsedBorderRadius, | ||
parsedPadding, | ||
parsedOuterPadding, | ||
start, | ||
end, | ||
viewBox, | ||
paths, | ||
viewBoxWidth, | ||
viewBoxHeight, | ||
totalSize, | ||
scaleFactor, | ||
} = memoizedValues; | ||
const shapeElement = | ||
shape === 'circle' ? ( | ||
<circle | ||
cx={totalSize / 2} | ||
cy={totalSize / 2} | ||
r={parseFloat(parsedSize) / 2} | ||
fill={activeBackgroundColor} | ||
/> | ||
) : shape === 'square' ? ( | ||
<rect | ||
x={parsedOuterPadding} | ||
y={parsedOuterPadding} | ||
width={parseFloat(parsedSize)} | ||
height={parseFloat(parsedSize)} | ||
fill={activeBackgroundColor} | ||
rx={parsedBorderRadius} | ||
/> | ||
) : null; | ||
const activeColor = | ||
isHovered && hoverColor ? parsedHoverColor : parsedColor; | ||
const activeGradientColors = | ||
isHovered && hoverGradientColors | ||
? parsedHoverGradientColors | ||
: parsedGradientColors; | ||
const activeBackgroundColor = | ||
isHovered && hoverBackgroundColor | ||
? parsedHoverBackgroundColor | ||
: parsedBackgroundColor; | ||
const wrapperStyle = { | ||
display: 'inline-block', | ||
borderRadius: shape === 'circle' ? '50%' : `${parsedBorderRadius}px`, | ||
lineHeight: 0, | ||
cursor: cursor, | ||
position: 'relative', | ||
}; | ||
const shapeElement = | ||
shape === 'circle' ? ( | ||
<circle | ||
cx={totalSize / 2} | ||
cy={totalSize / 2} | ||
r={parseFloat(parsedSize) / 2} | ||
fill={activeBackgroundColor} | ||
/> | ||
) : shape === 'square' ? ( | ||
<rect | ||
x={parsedOuterPadding} | ||
y={parsedOuterPadding} | ||
width={parseFloat(parsedSize)} | ||
height={parseFloat(parsedSize)} | ||
fill={activeBackgroundColor} | ||
rx={parsedBorderRadius} | ||
/> | ||
) : null; | ||
const svgStyle = { | ||
...styles, | ||
opacity: parsedOpacity, | ||
display: 'block', | ||
cursor: cursor, | ||
filter: iconShadow ? `drop-shadow(${iconShadow})` : 'none', | ||
}; | ||
const wrapperStyle = { | ||
display: 'inline-block', | ||
borderRadius: shape === 'circle' ? '50%' : `${parsedBorderRadius}px`, | ||
lineHeight: 0, | ||
cursor, | ||
position: 'relative', | ||
}; | ||
const svgContent = ( | ||
<svg | ||
width={totalSize} | ||
height={totalSize} | ||
viewBox={`0 0 ${totalSize} ${totalSize}`} | ||
xmlns="http://www.w3.org/2000/svg" | ||
style={svgStyle} | ||
onMouseEnter={() => setIsHovered(true)} | ||
onMouseLeave={() => setIsHovered(false)} | ||
{...props} | ||
> | ||
<defs> | ||
{activeGradientColors && ( | ||
<linearGradient | ||
id={`iconGradient-${name}`} | ||
x1={start.x} | ||
y1={start.y} | ||
x2={end.x} | ||
y2={end.y} | ||
const svgStyle = { | ||
...styles, | ||
opacity: parsedOpacity, | ||
display: 'block', | ||
cursor, | ||
filter: iconShadow ? `drop-shadow(${iconShadow})` : 'none', | ||
}; | ||
const svgContent = ( | ||
<svg | ||
width={totalSize} | ||
height={totalSize} | ||
viewBox={`0 0 ${totalSize} ${totalSize}`} | ||
xmlns="http://www.w3.org/2000/svg" | ||
style={svgStyle} | ||
onMouseEnter={handleMouseEnter} | ||
onMouseLeave={handleMouseLeave} | ||
{...props} | ||
> | ||
<defs> | ||
{activeGradientColors && ( | ||
<linearGradient | ||
id={`iconGradient-${name}`} | ||
x1={start.x} | ||
y1={start.y} | ||
x2={end.x} | ||
y2={end.y} | ||
> | ||
<stop offset="0%" stopColor={activeGradientColors[0]} /> | ||
<stop offset="100%" stopColor={activeGradientColors[1]} /> | ||
</linearGradient> | ||
)} | ||
</defs> | ||
{shapeElement} | ||
<g | ||
transform={`translate(${parsedOuterPadding + parsedPadding}, ${ | ||
parsedOuterPadding + parsedPadding | ||
}) scale(${scaleFactor})`} | ||
> | ||
{activeGradientColors ? ( | ||
<> | ||
<mask id={`iconMask-${name}`}> | ||
{paths.map((path, index) => ( | ||
<path key={index} d={path} fill="white" /> | ||
))} | ||
</mask> | ||
<rect | ||
x="0" | ||
y="0" | ||
width={viewBoxWidth} | ||
height={viewBoxHeight} | ||
fill={`url(#iconGradient-${name})`} | ||
mask={`url(#iconMask-${name})`} | ||
/> | ||
</> | ||
) : ( | ||
paths.map((path, index) => ( | ||
<path key={index} d={path} fill={activeColor} /> | ||
)) | ||
)} | ||
</g> | ||
</svg> | ||
); | ||
return ( | ||
<div style={wrapperStyle}> | ||
{boxShadow ? ( | ||
<div | ||
style={{ | ||
boxShadow, | ||
borderRadius: | ||
shape === 'circle' ? '50%' : `${parsedBorderRadius}px`, | ||
}} | ||
> | ||
<stop offset="0%" stopColor={activeGradientColors[0]} /> | ||
<stop offset="100%" stopColor={activeGradientColors[1]} /> | ||
</linearGradient> | ||
)} | ||
</defs> | ||
{shapeElement} | ||
<g | ||
transform={`translate(${parsedOuterPadding + parsedPadding}, ${ | ||
parsedOuterPadding + parsedPadding | ||
}) scale(${scaleFactor})`} | ||
> | ||
{activeGradientColors ? ( | ||
<mask id={`iconMask-${name}`}> | ||
{paths.map((path, index) => ( | ||
<path key={index} d={path} fill="white" /> | ||
))} | ||
</mask> | ||
) : null} | ||
{activeGradientColors ? ( | ||
<rect | ||
x="0" | ||
y="0" | ||
width={viewBoxWidth} | ||
height={viewBoxHeight} | ||
fill={`url(#iconGradient-${name})`} | ||
mask={`url(#iconMask-${name})`} | ||
/> | ||
{svgContent} | ||
</div> | ||
) : ( | ||
paths.map((path, index) => ( | ||
<path key={index} d={path} fill={activeColor} /> | ||
)) | ||
svgContent | ||
)} | ||
</g> | ||
</svg> | ||
); | ||
</div> | ||
); | ||
} | ||
); | ||
return ( | ||
<div style={wrapperStyle}> | ||
{boxShadow ? ( | ||
<div | ||
style={{ | ||
boxShadow: boxShadow, | ||
borderRadius: | ||
shape === 'circle' ? '50%' : `${parsedBorderRadius}px`, | ||
}} | ||
> | ||
{svgContent} | ||
</div> | ||
) : ( | ||
svgContent | ||
)} | ||
</div> | ||
); | ||
}; | ||
Icon.displayName = 'Icon'; | ||
export default Icon; |
783238
13843
162